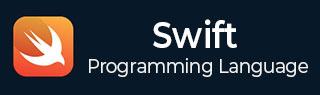
- Swift Tutorial
- Swift - Home
- Swift - Overview
- Swift - Environment
- Swift - Basic Syntax
- Swift - Variables
- Swift - Constants
- Swift - Literals
- Swift - Comments
- Swift Operators
- Swift - Operators
- Swift - Arithmetic Operators
- Swift - Comparison Operators
- Swift - Logical Operators
- Swift - Assignment Operators
- Swift - Bitwise Operators
- Swift - Misc Operators
- Swift Advanced Operators
- Swift - Operator Overloading
- Swift - Arithmetic Overflow Operators
- Swift - Identity Operators
- Swift - Range Operators
- Swift Data Types
- Swift - Data Types
- Swift - Integers
- Swift - Floating-Point Numbers
- Swift - Double
- Swift - Boolean
- Swift - Strings
- Swift - Characters
- Swift - Type Aliases
- Swift - Optionals
- Swift - Tuples
- Swift - Assertions and Precondition
- Swift Control Flow
- Swift - Decision Making
- Swift - if statement
- Swift - if...else if...else Statement
- Swift - if-else Statement
- Swift - nested if statements
- Swift - switch statement
- Swift - Loops
- Swift - for in loop
- Swift - While loop
- Swift - repeat...while loop
- Swift - continue statement
- Swift - break statement
- Swift - fall through statement
- Swift Collections
- Swift - Arrays
- Swift - Sets
- Swift - Dictionaries
- Swift Functions
- Swift - Functions
- Swift - Nested Functions
- Swift - Function Overloading
- Swift - Recursion
- Swift - Higher-Order Functions
- Swift Closures
- Swift - Closures
- Swift-Escaping and Non-escaping closure
- Swift - Auto Closures
- Swift OOps
- Swift - Enumerations
- Swift - Structures
- Swift - Classes
- Swift - Properties
- Swift - Methods
- Swift - Subscripts
- Swift - Inheritance
- Swift-Overriding
- Swift - Initialization
- Swift - Deinitialization
- Swift Advanced
- Swift - ARC Overview
- Swift - Optional Chaining
- Swift - Error handling
- Swift - Concurrency
- Swift - Type Casting
- Swift - Nested Types
- Swift - Extensions
- Swift - Protocols
- Swift - Generics
- Swift - Access Control
- Swift - Function vs Method
- Swift - SwiftyJSON
- Swift - Singleton class
- Swift Random Numbers
- Swift Opaque and Boxed Type
- Swift Useful Resources
- Swift - Compile Online
- Swift - Quick Guide
- Swift - Useful Resources
- Swift - Discussion
Swift - for-in Loop
Swift for-in Loop
The for-in loop iterates over collections of elements, such as ranges arrays, sets, dictionaries, etc. It iterates through each element of the specified collection without any explicit index manipulation. It is the most commonly used control flow statement because it expresses logic in a very clean and readable way.
It is also compatible with functional programming, which means you can easily use high-order functions like filter(), map(), reduce, etc with a for-in loop.
Syntax
Following is the syntax of the for-in loop −
for index in var{ Statement(s) }
Flow Diagram
The following flow diagram will show how the for-in loop works −
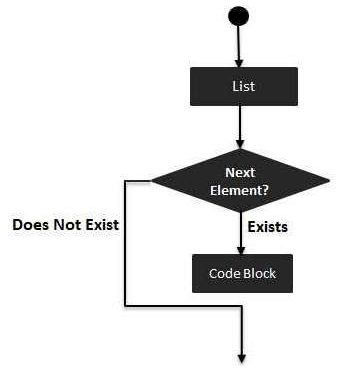
Example
Swift program to demonstrate the use of for-in loop.
import Foundation var someInts:[Int] = [10, 20, 30] for index in someInts { print( "Value of index is \(index)") }
Output
It will produce the following output −
Value of index is 10 Value of index is 20 Value of index is 30
Swift for-in Loop with Underscore "_"
In a for-in loop, we can also ignore values from the given collection with the help of underscore "_". Here we use underscore "_" in place of variable Name in the for-in loop. It will ignore the current value while iterating. It is commonly used when we only want to iterate a given number of times and does not require the value from the collection.
Syntax
Following is the syntax of the for-in loop with underscore −
for _ in var{ Statement(s) }
Example
Swift program to demonstrate how to use for-in loop with underscore.
import Foundation let numbers = [3, 5, 76, 12, 4] // Using the underscore to ignore the values for _ in numbers { // Following code will execute in each iteration print("Hello!! for-in loop!") }
Output
It will produce the following output −
Hello!! for-in loop! Hello!! for-in loop! Hello!! for-in loop! Hello!! for-in loop! Hello!! for-in loop!
Swift for-in Loop with Ranges
We can also use range with a for-in loop. A range is the easiest way to represent a range of values in the for-in loop, it can be open, closed or half-open. The for-in loop iterates over each value in the given range and in each iteration, the loop variable takes the value of the current element in the range.
Syntax
Following is the syntax of the for-in loop with range −
// With a closed range for variableName in start…end{ Statement(s) } // With half-open range for variableName in start..<end{ Statement(s) }
Example
Swift program to demonstrate how to use for-in loop with range.
import Foundation // Using closed Range print("Loop 1:") for x in 1...6 { print(x) } // Using half-open Range print("Loop 2:") for y in 1..<6 { print(y) }
Output
It will produce the following output −
Loop 1: 1 2 3 4 5 6 Loop 2: 1 2 3 4 5
Swift for-in Loop with stride() Function
We can use the stride() function with a for-in loop. The stride() function iterates over a range of values with a specific step between them. It is generally used to achieve more complex iteration patterns or can create a loop with a customized iteration pattern.
Syntax
Following is the syntax of the for-in loop with stride() function −
for variableName in stride(from: value, to: value, by: value){ statement(s) }
Parameters
The stride() function takes three parameters −
from − It contains the starting value of the range.
to − It contains the end value of the range. Its value does not include.
by − It contains the number of steps or strides between the values. If its value is positive, then the stride iterates in an upward direction or if the value is negative, then the stride iterates in the downward direction.
Example
Swift program to demonstrate how to use for-in loop with stride() function.
import Foundation // The stride() function moves in upward direction print("Loop 1:") for x in stride(from: 1, to: 7, by: 2) { print(x) } // The stride() function moves in downward direction print("\nLoop 2:") for y in stride(from: 7, to: 1, by: -2) { print(y) }
Output
It will produce the following output −
Loop 1: 1 3 5 Loop 2: 7 5 3
Swift for-in Loop with where Clause
Swift also provides a where clause which we can use with a for-in loop. A where clause is used to filter out only those elements that satisfy the condition specified in the where clause from the given collection. It can work with all iterable collections such as arrays, sets, and dictionaries.
Syntax
Following is the syntax of the for-in loop with where clause −
for variableName in collectionName where condition{ Statement(s) }
Example
Swift program to demonstrate how to use for-in loop with where clause.
import Foundation let nums = [43, 23, 66, 12, 2, 45, 33] // Using the where clause to filter out only even elements from the array for n in nums where n % 2 == 0 { print("Even number is \(n)") }
Output
It will produce the following output −
Even number is 66 Even number is 12 Even number is 2