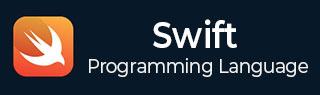
- Swift Tutorial
- Swift - Home
- Swift - Overview
- Swift - Environment
- Swift - Basic Syntax
- Swift - Variables
- Swift - Constants
- Swift - Literals
- Swift - Comments
- Swift Operators
- Swift - Operators
- Swift - Arithmetic Operators
- Swift - Comparison Operators
- Swift - Logical Operators
- Swift - Assignment Operators
- Swift - Bitwise Operators
- Swift - Misc Operators
- Swift Advanced Operators
- Swift - Operator Overloading
- Swift - Arithmetic Overflow Operators
- Swift - Identity Operators
- Swift - Range Operators
- Swift Data Types
- Swift - Data Types
- Swift - Integers
- Swift - Floating-Point Numbers
- Swift - Double
- Swift - Boolean
- Swift - Strings
- Swift - Characters
- Swift - Type Aliases
- Swift - Optionals
- Swift - Tuples
- Swift - Assertions and Precondition
- Swift Control Flow
- Swift - Decision Making
- Swift - if statement
- Swift - if...else if...else Statement
- Swift - if-else Statement
- Swift - nested if statements
- Swift - switch statement
- Swift - Loops
- Swift - for in loop
- Swift - While loop
- Swift - repeat...while loop
- Swift - continue statement
- Swift - break statement
- Swift - fall through statement
- Swift Collections
- Swift - Arrays
- Swift - Sets
- Swift - Dictionaries
- Swift Functions
- Swift - Functions
- Swift - Nested Functions
- Swift - Function Overloading
- Swift - Recursion
- Swift - Higher-Order Functions
- Swift Closures
- Swift - Closures
- Swift-Escaping and Non-escaping closure
- Swift - Auto Closures
- Swift OOps
- Swift - Enumerations
- Swift - Structures
- Swift - Classes
- Swift - Properties
- Swift - Methods
- Swift - Subscripts
- Swift - Inheritance
- Swift-Overriding
- Swift - Initialization
- Swift - Deinitialization
- Swift Advanced
- Swift - ARC Overview
- Swift - Optional Chaining
- Swift - Error handling
- Swift - Concurrency
- Swift - Type Casting
- Swift - Nested Types
- Swift - Extensions
- Swift - Protocols
- Swift - Generics
- Swift - Access Control
- Swift - Function vs Method
- Swift - SwiftyJSON
- Swift - Singleton class
- Swift Random Numbers
- Swift Opaque and Boxed Type
- Swift Useful Resources
- Swift - Compile Online
- Swift - Quick Guide
- Swift - Useful Resources
- Swift - Discussion
Swift - Auto Closures
A closure is a self-contained block of functionality that can passed around and used inside the code to perform any specific task. It can be assigned to a variable or passed as a function parameter. It can capture values from its surrounding context which is useful while working with asynchronous tasks. Swift supports various types of closures like escaping, non-escaping, and Auto closures. In this chapter, we will discuss about auto closure in detail.
Auto Closures in Swift
Swift supports a special type of closure that is known as auto closure. An auto closure is automatically created to wrap a statement that will passed as an argument to a specified function. It does not take any argument and only returns the value of the expression that it wraps inside at the time of calling. It is generally used with the function that takes closure as an argument and allows us to delay the evaluation of the expression until it is called or required.
Auto closure provides a concise syntax and is useful for passing a simple expression to the function as an argument instead of creating a separate closure. Also, remember that do not overuse auto closure because it makes our code harder to read.
Declaring Auto Closure
To declare a closure as an auto closure we have to use the @autoclosure keyword.
Syntax
Following is the syntax for auto closure −
func functionname(_closureParameter: @autoclosure() -> ReturnType){ // body }
Example
Swift simple program to demonstrate auto closure.
import Foundation // Defining a function that takes an auto-closure func functionForAutoclosure(myClosure: @autoclosure () -> Void) { print("Performing Action") myClosure() } // Calling the function with a closure as an argument functionForAutoclosure(myClosure: print("Hello! This is the auto closure"))
Output
It will produce the following output −
Performing Action Hello! This is the auto closure
Example
Swift program to add two numbers using auto closure.
// Function to find the sum of two numbers using auto-closure func addition(_ x: Int, _ y: Int, add: @autoclosure () -> Int) { let output = x + y + add() print("The sum of \(x) and \(y) is \(output)") } // Calling the function with a closure as an argument addition(2, 4, add: 7 + 8)
Output
It will produce the following output −
The sum of 2 and 4 is 21
Auto Closures as an Escape Closure
In Swift, we are allowed to escape an auto closure. An escaping closure is a special closure that passes as an argument to a function but is called after the function return. Auto closure and escaping closure both are different terms but we can combine them to delay the evaluation of the expression until it is required.
To declare an auto closure as an escape closure we have to use both the @autoclosure and @escaping keywords together.
Syntax
Following is the syntax for auto closure as an escape closure −
func functionName(_closureParameter: @autoclosure @escaping() -> ReturnType){ // body }
Example
Swift simple program to demonstrate auto closure as an escaping closure.
import Foundation class AutoClosureExample { var queue: [() -> Void] = [] // Method that takes an auto closure as an escaping closure func closureToQueue(_ myClosure: @autoclosure @escaping () -> Void) { queue.append(myClosure) } // Method that executes the closures in the queue func runClosure() { for closure in queue { closure() } } } // Creating object of AutoClosureExample class let obj = AutoClosureExample() // Using an auto closure as an escaping closure obj.closureToQueue(print("My Closure 1")) obj.closureToQueue(print("My Closure 2")) // Activating the passage of time DispatchQueue.main.asyncAfter(deadline: .now() + 2) { // Execute the closures in the queue obj.runClosure() }
Output
It will produce the following output −
My Closure 1 My Closure 2
This program will run on Xcode or local Swift playground. It does not work on online Swift compilers because online Swift compilers are not compatible with executing asynchronous operations.