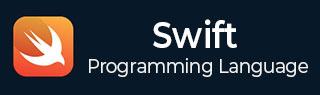
- Swift Tutorial
- Swift - Home
- Swift - Overview
- Swift - Environment
- Swift - Basic Syntax
- Swift - Variables
- Swift - Constants
- Swift - Literals
- Swift - Comments
- Swift Operators
- Swift - Operators
- Swift - Arithmetic Operators
- Swift - Comparison Operators
- Swift - Logical Operators
- Swift - Assignment Operators
- Swift - Bitwise Operators
- Swift - Misc Operators
- Swift Advanced Operators
- Swift - Operator Overloading
- Swift - Arithmetic Overflow Operators
- Swift - Identity Operators
- Swift - Range Operators
- Swift Data Types
- Swift - Data Types
- Swift - Integers
- Swift - Floating-Point Numbers
- Swift - Double
- Swift - Boolean
- Swift - Strings
- Swift - Characters
- Swift - Type Aliases
- Swift - Optionals
- Swift - Tuples
- Swift - Assertions and Precondition
- Swift Control Flow
- Swift - Decision Making
- Swift - if statement
- Swift - if...else if...else Statement
- Swift - if-else Statement
- Swift - nested if statements
- Swift - switch statement
- Swift - Loops
- Swift - for in loop
- Swift - While loop
- Swift - repeat...while loop
- Swift - continue statement
- Swift - break statement
- Swift - fall through statement
- Swift Collections
- Swift - Arrays
- Swift - Sets
- Swift - Dictionaries
- Swift Functions
- Swift - Functions
- Swift - Nested Functions
- Swift - Function Overloading
- Swift - Recursion
- Swift - Higher-Order Functions
- Swift Closures
- Swift - Closures
- Swift-Escaping and Non-escaping closure
- Swift - Auto Closures
- Swift OOps
- Swift - Enumerations
- Swift - Structures
- Swift - Classes
- Swift - Properties
- Swift - Methods
- Swift - Subscripts
- Swift - Inheritance
- Swift-Overriding
- Swift - Initialization
- Swift - Deinitialization
- Swift Advanced
- Swift - ARC Overview
- Swift - Optional Chaining
- Swift - Error handling
- Swift - Concurrency
- Swift - Type Casting
- Swift - Nested Types
- Swift - Extensions
- Swift - Protocols
- Swift - Generics
- Swift - Access Control
- Swift - Function vs Method
- Swift - SwiftyJSON
- Swift - Singleton class
- Swift Random Numbers
- Swift Opaque and Boxed Type
- Swift Useful Resources
- Swift - Compile Online
- Swift - Quick Guide
- Swift - Useful Resources
- Swift - Discussion
Swift - Double
Double is a standard data type in Swift. Double data type is used to store decimal numbers like 23.344, 45.223221, 0.324343454, etc. It is a 64-bit floating-point number and stores values up to 15 decimal digits which makes it more accurate as compared to Float.
If you create a variable to store a decimal number without specifying its type, then by default compiler will assume it is a Double type instead of a Float type, due to high precision.
Syntax
Following is the syntax of the Double data type −
let num : Double = 23.4554
Following is the shorthand syntax of the Double data type −
let num = 2.73937
Example
Swift program to calculate the sum of two double numbers.
import Foundation // Defining double numbers let num1 : Double = 2.3764 let num2 : Double = 12.738 // Store the sum of two double numbers var sum : Double = 0.0 sum = num1 + num2 print("Sum of \(num1) and \(num2) = \(sum)")
Output
Sum of 2.3764 and 12.738 = 15.1144
Example
Swift program to calculate the product of two double numbers.
import Foundation // Defining double numbers let num1 = 12.3764832 let num2 = 22.7388787779074 // Store the product of two double numbers var product = 0.0 product = num1 * num2 print("Product of \(num1) and \(num2) = \(product)")
Output
Product of 12.3764832 and 22.7388787779074 = 281.42735118160743
Difference Between Float and Double
The following are the major differences between the floating point data type and the double data type.
Double | Float |
---|---|
It has precision of at least 15 decimal digits. | It has precision of at least 6 decimal digits. |
Memory size is of 8 bytes. | Memory size is of 4 bytes. |
If no data type is defined, then compiler will treat it as Double. | It is not preferred by the compiler by default. |