
- NHibernate Tutorial
- NHibernate - Home
- NHibernate - Overview
- NHibernate - Architecture
- NHibernate - Orm
- NHibernate - Environment Setup
- NHibernate - Getting Started
- NHibernate - Basic Orm
- NHibernate - Basic Crud Operations
- NHibernate - Profiler
- Add Intelliesnse To Mapping File
- NHibernate - Data Types Mapping
- NHibernate - Configuration
- NHibernate - Override Configuration
- NHibernate - Batch Size
- NHibernate - Caching
- NHibernate - Mapping Component
- NHibernate - Relationships
- NHibernate - Collection Mapping
- NHibernate - Cascades
- NHibernate - Lazy Loading
- NHibernate - Inverse Relationships
- NHibernate - Load/Get
- NHibernate - Linq
- NHibernate - Query Language
- NHibernate - Criteria Queries
- NHibernate - QueryOver Queries
- NHibernate - Native Sql
- NHibernate - Fluent Hibernate
- NHibernate Useful Resources
- NHibernate - Quick Guide
- NHibernate - Useful Resources
- NHibernate - Discussion
NHibernate - Mapping Component
In this chapter, we will be talking about mapping components. In NHibernate, component is a value object. It does not have an identity of its own.
An example of this would be a money object, a purse or a wallet might have money in it, but the exact identity of that money is irrelevant.
It doesn't have its own primary key, but components themselves are persistent in the same table as the owning object.
Let’s have a look at a simple example in which a student has an Address, which is an object of Location class associated with it.
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace NHibernateDemoApp { class Student { public virtual int ID { get; set; } public virtual string LastName { get; set; } public virtual string FirstName { get; set; } public virtual StudentAcademicStanding AcademicStanding { get; set; } public virtual Location Address { get; set; } } public class Location { public virtual string Street { get; set; } public virtual string City { get; set; } public virtual string Province { get; set; } public virtual string Country { get; set; } } public enum StudentAcademicStanding { Excellent, Good, Fair, Poor, Terrible } }
Now, we also need to update the database by executing the following query, which will first drop the Student table and then create a new table that will also contain a column for Location class.
DROP TABLE [dbo].[Student] CREATE TABLE [dbo].[Student] ( [ID] INT IDENTITY (1, 1) NOT NULL, [LastName] NVARCHAR (MAX) NULL, [FirstMidName] NVARCHAR (MAX) NULL, [AcademicStanding] NCHAR(10) NULL, [Street] NVARCHAR (100) NULL, [City] NVARCHAR (100) NULL, [Province] NVARCHAR (100) NULL, [Country] NVARCHAR (100) NULL, CONSTRAINT [PK_dbo.Student] PRIMARY KEY CLUSTERED ([ID] ASC) );
Now to map those columns that are not directly a part of Student class, but they are properties of Location class and Location class object is defined in the student class. We need a component to map it correctly. Let’s create a component in student.hbm.xml file as shown in the following code.
<?xml version = "1.0" encoding = "utf-8" ?> <hibernate-mapping xmlns = "urn:nhibernate-mapping-2.2" assembly = "NHibernateDemoApp" namespace = "NHibernateDemoApp"> <class name = "Student"> <id name = "ID"> <generator class = "native"/> </id> <property name = "LastName"/> <property name = "FirstName" column = "FirstMidName" type = "String"/> <property name = "AcademicStanding"/> <component name = "Address"> <property name = "Street"/> <property name = "City"/> <property name = "Province"/> <property name = "Country"/> </component> </class> </hibernate-mapping>
This component is the Address and it has these different properties on it. With this information, NHibernate now has enough that it can actually map this.
Now here is the Program.cs file in which a new student object is created and initialized and then saved to the database. It will then retrieve the list from the database.
using HibernatingRhinos.Profiler.Appender.NHibernate; using NHibernate.Cache; using NHibernate.Caches.SysCache; using NHibernate.Cfg; using NHibernate.Dialect; using NHibernate.Driver; using NHibernate.Linq; using System; using System.Linq; using System.Reflection; namespace NHibernateDemoApp { class Program { static void Main(string[] args) { NHibernateProfiler.Initialize(); var cfg = new Configuration(); String Data Source = asia13797\\sqlexpress; String Initial Catalog = NHibernateDemoDB; String Integrated Security = True; String Connect Timeout = 15; String Encrypt = False; String TrustServerCertificate = False; String ApplicationIntent = ReadWrite; String MultiSubnetFailover = False; cfg.DataBaseIntegration(x = > { x.ConnectionString = "Data Source + Initial Catalog + Integrated Security + Connect Timeout + Encrypt + TrustServerCertificate + ApplicationIntent + MultiSubnetFailover"; x.Driver<SqlClientDriver>(); x.Dialect<MsSql2008Dialect>(); }); cfg.AddAssembly(Assembly.GetExecutingAssembly()); var sefact = cfg.BuildSessionFactory(); using (var session = sefact.OpenSession()) { using (var tx = session.BeginTransaction()) { var student1 = new Student { ID = 1, FirstName = "Allan", LastName = "Bommer", AcademicStanding = StudentAcademicStanding.Poor, Address = new Location { Street = "123 Street", City = "Lahore", Province = "Punjab", Country = "Pakistan" } }; session.Save(student1); tx.Commit(); var students = session.Query<Student>().ToList<Student>(); Console.WriteLine("\nFetch the complete list again\n"); foreach (var student in students) { Console.WriteLine("{0} \t{1} \t{2} \t{3} \t{4} \t{5} \t{6} \t{7}", student.ID, student.FirstName, student.LastName, student.AcademicStanding, student.Address.Street, student.Address.City, student.Address.Province, student.Address.Country ); } } Console.ReadLine(); } } } }
Now we can run this application and NHibernate can save those values to the database. When you run the application, you will see the following output.
Fetch the complete list again 2 Allan Bommer Poor 123 Street Lahore Punjab Pakistan
Here are the values in the database.
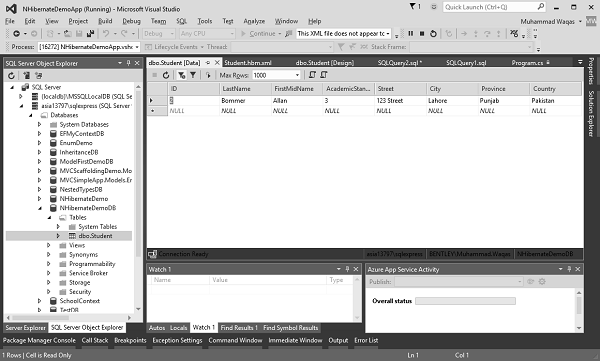
The components allow us to separate out columns that are in a database table into their own separate class.
The other thing to notice here is because the Location is a class, it is not an entity.
It is a value type object and it doesn't have its own primary key.
It is saved in the same table as the Student that contains it.
That's why we're using the component here.
This allows a lot of flexibility to change our class layer, how our classes are defined versus how our database is laid out.