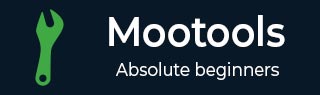
- MooTools Tutorial
- MooTools - Home
- MooTools - Introduction
- MooTools - Installation
- MooTools - Program Structure
- MooTools - Selectors
- MooTools - Using Arrays
- MooTools - Functions
- MooTools - Event Handling
- MooTools - DOM Manipulations
- MooTools - Style Properties
- MooTools - Input Filtering
- MooTools - Drag and Drop
- MooTools - Regular Expression
- MooTools - Periodicals
- MooTools - Sliders
- MooTools - Sortables
- MooTools - Accordion
- MooTools - Tooltips
- MooTools - Tabbed Content
- MooTools - Classes
- MooTools - Fx.Element
- MooTools - Fx.Slide
- MooTools - Fx.Tween
- MooTools - Fx.Morph
- MooTools - Fx.Options
- MooTools - Fx.Events
- MooTools Useful Resources
- MooTools - Quick Guide
- MooTools - Useful Resources
- MooTools - Discussion
MooTools - Input Filtering
MooTools can filter the user input and it can easily recognize the type of input. The basic input types are Number and String.
Number Functions
Let us discuss a few methods that will check if an input value is a number or not. These methods will also help you manipulate the number input.
toInt()
This method converts any input value to the integer. You can call it on a variable and it will try to give the regular integer from whatever the variable contains.
Let us take an example that design a web page that contain a textbox and a button named TO INT. The button will check and return the value that you entered into the textbox as real integer. If the value is not an integer, then it will return the NaN symbol. Take a look at the following code.
Example
<!DOCTYPE html> <html> <head> <script type = "text/javascript" src = "MooTools-Core-1.6.0.js"></script> <script type = "text/javascript" src = "MooTools-More-1.6.0.js"></script> <script type = "text/javascript"> var toIntDemo = function(){ var input = $('input').get('value'); var number = input.toInt(); alert ('Value is : ' + number); } window.addEvent('domready', function() { $('toint').addEvent('click', toIntDemo); }); </script> </head> <body> Enter some value: <input type = "text" id = "input" /> <input type = "button" id = "toint" value = "TO INT"/> </body> </html>
You will receive the following output −
Output
Try different values and convert them into real integers.
typeOf()
This method examines the value of a variable you pass and, it returns the type of that value.
Let us take an example wherein, we design a webpage and check if the input value is Number, String or Boolean. Take a look at the following code.
Example
<!DOCTYPE html> <html> <head> <script type = "text/javascript" src = "MooTools-Core-1.6.0.js"></script> <script type = "text/javascript" src = "MooTools-More-1.6.0.js"></script> <script type = "text/javascript"> var checkType = function(){ var input = $('input').get('value'); var int_input = input.toInt(); if(typeOf(int_input) != 'number'){ if(input == 'false' || input == 'true'){ alert("Variable type is : Boolean"+" - and value is: "+input); } else{ alert("Variable type is : "+typeof(input)+" - and value is: "+input); } } else{ alert("Variable type is : "+typeof(int_input)+" - and value is:"+int_input); } } window.addEvent('domready', function() { $('checktype').addEvent('click', checkType); }); </script> </head> <body> Enter some value: <input type = "text" id = "input" /> <input type = "button" id = "checktype" value = "CHECK TYPE"/> </body> </html>
You will receive the following output −
Output
Try the different values and check the type.
limit()
The limit() method is used to set the lower bound and upper bound values for a particular number. The number should not exceed the upper bound value. If it exceeds, then the number is changed to the upper bound value. This process is same with the lower bound also.
Let us take an example that provides a text box for entering a value, provide a button to check the limit of that value. The default limit that we used in the example is 0 to 255. Take a look at the following code.
Example
<!DOCTYPE html> <html> <head> <script type = "text/javascript" src = "MooTools-Core-1.6.0.js"></script> <script type = "text/javascript" src = "MooTools-More-1.6.0.js"></script> <script type = "text/javascript"> var checkLimit = function(){ var input = $('input').get('value'); var number = input.toInt(); var limited_number = number.limit(0, 255); alert("Number is : " + limited_number); } window.addEvent('domready', function() { $('check_limit').addEvent('click', checkLimit); }); </script> </head> <body> Enter some value: <input type = "text" id = "input" /> <input type = "button" id = "check_limit" value = "Check Limit (0 to 255)"/> </body> </html>
You will receive the following output −
Output
Try different numbers to check the limit.
rgbToHex()
The rgbToHex() method is to convert from the red, green, and blue values to the Hexadecimal value. This function deals with numbers and belongs to the Array collection. Let us take an example wherein, we will design a web page to enter the individual values for Red, Green, and Blue. Provide a button to convert all three into hexadecimal values. Take a look at the following code.
Example
<!DOCTYPE html> <html> <head> <script type = "text/javascript" src = "MooTools-Core-1.6.0.js"></script> <script type = "text/javascript" src = "MooTools-More-1.6.0.js"></script> <script type = "text/javascript"> var rgbToHexa_Demo = function(){ var red = $('red').get('value'); var red_value = red.toInt(); var green = $('green').get('value'); var green_value = green.toInt(); var blue = $('blue').get('value'); var blue_value = blue.toInt(); var color = [red_value, green_value, blue_value].rgbToHex(); alert(" Hexa color is : " + color); } window.addEvent('domready', function() { $('rgbtohex').addEvent('click', rgbToHexa_Demo); }); </script> </head> <body> Red Value: <input type = "text" id = "red" /><br/><br/> Green Value: <input type = "text" id = "green" /><br/><br/> Blue Value: <input type = "text" id = "blue" /><br/><br/> <input type = "button" id = "rgbtohex" value = "RGB To HEX"/> </body> </html>
You will receive the following output −
Output
Try different Red, Green, and Blue values and find the hexadecimal values.
String Functions
Let us discuss a few methods of String class which can manipulate the input String value. Before we proceed, let us take a look at the following syntax of how to call a string function.
String
var my_variable = "Heres some text"; var result_of_function = my_variable.someStringFunction();
Or,
var result_of_function = "Heres some text".someStringFunction();
trim()
This method is used to remove the whitespace of the front position and the end position of a given string. It does not touch any white spaces inside the string. Take a look at the following code.
Example
<!DOCTYPE html> <html> <head> <script type = "text/javascript" src = "MooTools-Core-1.6.0.js"></script> <script type = "text/javascript" src = "MooTools-More-1.6.0.js"></script> <script type = "text/javascript"> window.addEvent('domready', function() { var input_str = " This is tutorialspoint.com "; document.writeln("<pre>Before trim String is : |-"+input_str+"-|</pre>"); var trim_string = input_str.trim(); document.writeln("<pre>After trim String is : |-"+trim_string+"-|</pre>"); }); </script> </head> <body> </body> </html>
You will receive the following output −
Output
In the above alert boxes, you can find the differences in String before calling trim() method and after calling trim() method.
clean()
This method is used to remove all white spaces from the given string and maintain single space between the words. Take a look at the following code.
Example
<!DOCTYPE html> <html> <head> <script type = "text/javascript" src = "MooTools-Core-1.6.0.js"></script> <script type = "text/javascript" src = "MooTools-More-1.6.0.js"></script> <script type = "text/javascript"> window.addEvent('domready', function() { var input_str = " This is tutorialspoint.com "; document.writeln("<pre>Before clean String is : |-"+input_str+"-|</pre>"); var trim_string = input_str.clean(); document.writeln("<pre>After clean String is : |-"+trim_string+"-|</pre>"); }); </script> </head> <body> </body> </html>
You will receive the following output −
Output
contains()
This method is used to search a sub-string in a given string. If the given string contains the search string, it returns true otherwise it returns false. Take a look at the following code.
Example
<!DOCTYPE html> <html> <head> <script type = "text/javascript" src = "MooTools-Core-1.6.0.js"></script> <script type = "text/javascript" src = "MooTools-More-1.6.0.js"></script> <script type = "text/javascript"> var containsString = function(){ var input_string = "Hai this is tutorialspoint"; var search_string = $('input').get('value'); var string_contains = input_string.contains(search_string); alert("contains : " + string_contains); } window.addEvent('domready', function() { $('contains').addEvent('click', containsString); }); </script> </head> <body> Given String : <p>Hai this is tutorialspoint</p> Enter search string: <input type = "text" id = "input" /> <input type = "button" id = "contains" value = "Search String"/> </body> </html>
You will receive the following output −
Output
substitute()
This method is used to insert the input string into the main string. Take a look at the following code.
Example
<!DOCTYPE html> <html> <head> <script type = "text/javascript" src = "MooTools-Core-1.6.0.js"></script> <script type = "text/javascript" src = "MooTools-More-1.6.0.js"></script> <script type = "text/javascript"> var containsString = function(){ var input_string = "One is {one}, Two is {two}, Three is {three}"; var one_str = $('one').get('value'); var two_str = $('two').get('value'); var three_str = $('three').get('value'); var substitution_string = { one : one_str, two : two_str, three : three_str } var new_string = input_string.substitute(substitution_string); document.write("NEW STRING IS : " + new_string); } window.addEvent('domready', function() { $('contains').addEvent('click', containsString); }); </script> </head> <body> Given String : <p>One is {one}, Two {two}, Three is {three}</p> one String : <input type = "text" id = "one" /><br/><br/> two String : <input type = "text" id = "two" /><br/><br/> three String : <input type = "text" id = "three" /><br/><br/> <input type = "button" id = "contains" value = "Substitute String"/> </body> </html>
You will receive the following output −
Output
Enter text in the three text boxes and click on the substitute string button, then you will get to see the substitution string.