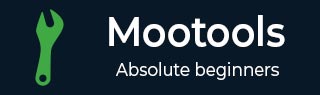
- MooTools Tutorial
- MooTools - Home
- MooTools - Introduction
- MooTools - Installation
- MooTools - Program Structure
- MooTools - Selectors
- MooTools - Using Arrays
- MooTools - Functions
- MooTools - Event Handling
- MooTools - DOM Manipulations
- MooTools - Style Properties
- MooTools - Input Filtering
- MooTools - Drag and Drop
- MooTools - Regular Expression
- MooTools - Periodicals
- MooTools - Sliders
- MooTools - Sortables
- MooTools - Accordion
- MooTools - Tooltips
- MooTools - Tabbed Content
- MooTools - Classes
- MooTools - Fx.Element
- MooTools - Fx.Slide
- MooTools - Fx.Tween
- MooTools - Fx.Morph
- MooTools - Fx.Options
- MooTools - Fx.Events
- MooTools Useful Resources
- MooTools - Quick Guide
- MooTools - Useful Resources
- MooTools - Discussion
MooTools - Event Handling
Like Selectors, Event Handling is also an essential concept of MooTools. This concept is used to create events and actions for events. We also need to have a grasp of the actions and their effects. Let us try a few events in this chapter.
Single left click
The most common event in web development is Single Left Click. For example, Hyperlink recognizes a single click event and takes you to another DOM element. The first step is to add a click event to the DOM element. Let us take an example that adds a click event to the button. When you click on that button, it will display a message.
Example
<!DOCTYPE html> <html> <head> <script type = "text/javascript" src = "MooTools-Core-1.6.0.js"></script> <script type = "text/javascript" src = "MooTools-More-1.6.0.js"></script> <script type = "text/javascript"> var clickFunction = function(){ //put whatever you want to happen in here document.write('This button element recognizes the click event'); } window.addEvent('domready', function() { $('id_name').addEvent('click', clickFunction); }); </script> </head> <body> <input type = "button" id = "id_name" value = "click here"/> </body> </html>
You will receive the following output −
Output
When you click on the button, you will get the following message −
This button element recognizes the click event
Mouse Enter and Mouse Leave
Mouse Enter and Mouse Leave are the most common events in event handling. The action is applied based on the position of the mouse. If the position of the mouse is ENTER into the DOM element, then it will apply one action. If it leaves the DOM element area, then it will apply another action.
Let us take an example that explains how mouse Enter event works. Take a look at the following code.
Example
<!DOCTYPE html> <html> <head> <script type = "text/javascript" src = "MooTools-Core-1.6.0.js"></script> <script type = "text/javascript" src = "MooTools-More-1.6.0.js"></script> <script type = "text/javascript"> var mouseEnterFunction = function(){ //put whatever you want to happen in here $('result').set('html', "Recognizes the mouse enter event"); } window.addEvent('domready', function() { $('id_name').addEvent('mouseenter', mouseEnterFunction); }); </script> </head> <body> <input type = "button" id = "id_name" value = "Mouse Enter"/> <br/><br/> <lable id = "result"></lable> </body> </html>
You will receive the following output −
Output
If you keep your mouse pointer on the button, then you will get the following message.
Recognizes the mouse enter event
Let us take an example that explains how the Mouse Leave event works. Take a look at the following code.
Example
<!DOCTYPE html> <html> <head> <script type = "text/javascript" src = "MooTools-Core-1.6.0.js"></script> <script type = "text/javascript" src = "MooTools-More-1.6.0.js"></script> <script type = "text/javascript"> var mouseLeaveFunction = function(){ //put whatever you want to happen in here $('result').set('html', "Recognizes the mouse leave event"); } window.addEvent('domready', function() { $('id_name').addEvent('mouseleave', mouseLeaveFunction); }); </script> </head> <body> <input type = "button" id = "id_name" value = "Mouse Leave"/><br/> <lable id = "result"></lable> </body> </html>
You will receive the following output −
Output
If you keep your mouse pointer on the button, then you will get the following message.
Recognizes the mouse leave event
Remove an Event
This method is used to remove an event. Removing an event is just as easy as adding an event and it follows the same structure. Take a look at the following syntax.
Syntax
//works just like the previous examplesuse .removeEvent method $('id_name').removeEvent('mouseleave', mouseLeaveFunction);
Keystrokes as Input
MooTools can recognize your actions — the kind of input you have given through the DOM element. By using the keydown function, you can read each and every key from the input type DOM element.
Let us take an example wherein, there is a text area element. Let us now add a keydown event to the text area that whenever the text area recognizes any keystore, it will respond with an alert message immediately. Take a look at the following code.
Example
<!DOCTYPE html> <html> <head> <script type = "text/javascript" src = "MooTools-Core-1.6.0.js"></script> <script type = "text/javascript" src = "MooTools-More-1.6.0.js"></script> <script type = "text/javascript"> var keydownEventFunction = function () { alert('This textarea can now recognize keystroke value'); }; window.addEvent('domready', function() { $('myTextarea').addEvent('keydown', keydownEventFunction); }); </script> </head> <body> Write Something: <textarea id = "myTextarea"> </textarea> </body> </html>
You will receive the following output −
Output
Try to enter something into the text area. You will find an alert box along with the following message.
This textarea can now recognize keystroke value
Try to add some text to the same example that reads the value from the textarea when you entered into it. It is possible by using event.key function with the event. Take a look at the following code.
Example
<!DOCTYPE html> <html> <head> <script type = "text/javascript" src = "MooTools-Core-1.6.0.js"></script> <script type = "text/javascript" src = "MooTools-More-1.6.0.js"></script> <script type = "text/javascript"> //notice the parameter "event" within the function parenthesis var keyStrokeEvent = function(event){ var x = event.key; alert("The enter value is: "+x) } window.addEvent('domready', function() { $('myTextarea').addEvent('keydown', keyStrokeEvent); }); </script> </head> <body> <lable>Write Something:</lable> <br/> <textarea id = "myTextarea"> </textarea> </body> </html>
You will receive the following output −
Output
Try to enter text in the text area. You will be directed to an alert box along with the value you entered into the text area.