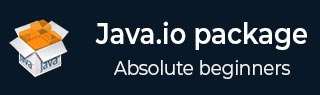
- Java.io package classes
- Java.io - Home
- Java.io - BufferedInputStream
- Java.io - BufferedOutputStream
- Java.io - BufferedReader
- Java.io - BufferedWriter
- Java.io - ByteArrayInputStream
- Java.io - ByteArrayOutputStream
- Java.io - CharArrayReader
- Java.io - CharArrayWriter
- Java.io - Console
- Java.io - DataInputStream
- Java.io - DataOutputStream
- Java.io - File
- Java.io - FileDescriptor
- Java.io - FileInputStream
- Java.io - FileOutputStream
- Java.io - FilePermission
- Java.io - FileReader
- Java.io - FileWriter
- Java.io - FilterInputStream
- Java.io - FilterOutputStream
- Java.io - FilterReader
- Java.io - FilterWriter
- Java.io - InputStream
- Java.io - InputStreamReader
- Java.io - LineNumberInputStream
- Java.io - LineNumberReader
- Java.io - ObjectInputStream
- Java.io - ObjectInputStream.GetField
- Java.io - ObjectOutputStream
- io - ObjectOutputStream.PutField
- Java.io - ObjectStreamClass
- Java.io - ObjectStreamField
- Java.io - OutputStream
- Java.io - OutputStreamWriter
- Java.io - PipedInputStream
- Java.io - PipedOutputStream
- Java.io - PipedReader
- Java.io - PipedWriter
- Java.io - PrintStream
- Java.io - PrintWriter
- Java.io - PushbackInputStream
- Java.io - PushbackReader
- Java.io - RandomAccessFile
- Java.io - Reader
- Java.io - SequenceInputStream
- Java.io - SerializablePermission
- Java.io - StreamTokenizer
- Java.io - StringBufferInputStream
- Java.io - StringReader
- Java.io - StringWriter
- Java.io - Writer
- Java.io package extras
- Java.io - Interfaces
- Java.io - Exceptions
- Java.io package Useful Resources
- Java.io - Discussion
Java.io.OutputStream.write() Method
Description
The java.io.OutputStream.write(int b) method writes the specified byte to this output stream. The general contract for write is that one byte is written to the output stream. The byte to be written is the eight low-order bits of the argument b. The 24 high-order bits of b are ignored.
Subclasses of OutputStream must provide an implementation for this method.
Declaration
Following is the declaration for java.io.OutputStream.write() method.
public abstract void write(int b)
Parameters
b − The byte.
Return Value
This method does not return a value.
Exception
IOException − If an I/O error occurs. In particular, an IOException is thrown if the output stream is closed.
Example
The following example shows the usage of java.io.OutputStream.write() method.
package com.tutorialspoint; import java.io.*; public class OutputStreamDemo { public static void main(String[] args) { try { // create a new output stream OutputStream os = new FileOutputStream("test.txt"); // craete a new input stream InputStream is = new FileInputStream("test.txt"); // write something os.write(70); os.write(71); // read what we wrote for (int i = 0; i < 2; i++) { System.out.print("" + (char) is.read()); } } catch (Exception ex) { ex.printStackTrace(); } } }
Let us compile and run the above program, this will produce the following result −
FG
java_io_outputstream.htm
Advertisements