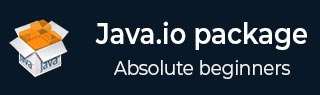
- Java.io package classes
- Java.io - Home
- Java.io - BufferedInputStream
- Java.io - BufferedOutputStream
- Java.io - BufferedReader
- Java.io - BufferedWriter
- Java.io - ByteArrayInputStream
- Java.io - ByteArrayOutputStream
- Java.io - CharArrayReader
- Java.io - CharArrayWriter
- Java.io - Console
- Java.io - DataInputStream
- Java.io - DataOutputStream
- Java.io - File
- Java.io - FileDescriptor
- Java.io - FileInputStream
- Java.io - FileOutputStream
- Java.io - FilePermission
- Java.io - FileReader
- Java.io - FileWriter
- Java.io - FilterInputStream
- Java.io - FilterOutputStream
- Java.io - FilterReader
- Java.io - FilterWriter
- Java.io - InputStream
- Java.io - InputStreamReader
- Java.io - LineNumberInputStream
- Java.io - LineNumberReader
- Java.io - ObjectInputStream
- Java.io - ObjectInputStream.GetField
- Java.io - ObjectOutputStream
- io - ObjectOutputStream.PutField
- Java.io - ObjectStreamClass
- Java.io - ObjectStreamField
- Java.io - OutputStream
- Java.io - OutputStreamWriter
- Java.io - PipedInputStream
- Java.io - PipedOutputStream
- Java.io - PipedReader
- Java.io - PipedWriter
- Java.io - PrintStream
- Java.io - PrintWriter
- Java.io - PushbackInputStream
- Java.io - PushbackReader
- Java.io - RandomAccessFile
- Java.io - Reader
- Java.io - SequenceInputStream
- Java.io - SerializablePermission
- Java.io - StreamTokenizer
- Java.io - StringBufferInputStream
- Java.io - StringReader
- Java.io - StringWriter
- Java.io - Writer
- Java.io package extras
- Java.io - Interfaces
- Java.io - Exceptions
- Java.io package Useful Resources
- Java.io - Discussion
Java.io.FilterInputStream.markSupported() Method
Description
The java.io.FilterInputStream.markSupported() method tests if this input stream supports mark() and reset() invocations.
Declaration
Following is the declaration for java.io.FilterInputStream.markSupported() method −
public boolean markSupported()
Parameters
NA
Return Value
The method returns true if the stream type supports mark and reset methods.
Exception
NA
Example
The following example shows the usage of java.io.FilterInputStream.markSupported() method.
package com.tutorialspoint; import java.io.BufferedInputStream; import java.io.FileInputStream; import java.io.FilterInputStream; import java.io.IOException; import java.io.InputStream; public class FilterInputStreamDemo { public static void main(String[] args) throws Exception { InputStream is = null; FilterInputStream fis = null; boolean bool = false; try { // create input streams is = new FileInputStream("C://test.txt"); fis = new BufferedInputStream(is); // tests if the input stream supports mark() and reset() bool = fis.markSupported(); // prints System.out.print("Supports mark and reset methods: "+bool); } catch(IOException e) { // if any I/O error occurs e.printStackTrace(); } finally { // releases any system resources associated with the stream if(is!=null) is.close(); if(fis!=null) fis.close(); } } }
Assuming we have a text file c:/test.txt, which has the following content. This file will be used as an input for our example program −
ABCDEF
Let us compile and run the above program, this will produce the following result −
Supports mark and reset methods: true
java_io_filterinputstream.htm
Advertisements