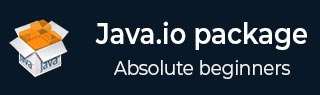
- Java.io package classes
- Java.io - Home
- Java.io - BufferedInputStream
- Java.io - BufferedOutputStream
- Java.io - BufferedReader
- Java.io - BufferedWriter
- Java.io - ByteArrayInputStream
- Java.io - ByteArrayOutputStream
- Java.io - CharArrayReader
- Java.io - CharArrayWriter
- Java.io - Console
- Java.io - DataInputStream
- Java.io - DataOutputStream
- Java.io - File
- Java.io - FileDescriptor
- Java.io - FileInputStream
- Java.io - FileOutputStream
- Java.io - FilePermission
- Java.io - FileReader
- Java.io - FileWriter
- Java.io - FilterInputStream
- Java.io - FilterOutputStream
- Java.io - FilterReader
- Java.io - FilterWriter
- Java.io - InputStream
- Java.io - InputStreamReader
- Java.io - LineNumberInputStream
- Java.io - LineNumberReader
- Java.io - ObjectInputStream
- Java.io - ObjectInputStream.GetField
- Java.io - ObjectOutputStream
- io - ObjectOutputStream.PutField
- Java.io - ObjectStreamClass
- Java.io - ObjectStreamField
- Java.io - OutputStream
- Java.io - OutputStreamWriter
- Java.io - PipedInputStream
- Java.io - PipedOutputStream
- Java.io - PipedReader
- Java.io - PipedWriter
- Java.io - PrintStream
- Java.io - PrintWriter
- Java.io - PushbackInputStream
- Java.io - PushbackReader
- Java.io - RandomAccessFile
- Java.io - Reader
- Java.io - SequenceInputStream
- Java.io - SerializablePermission
- Java.io - StreamTokenizer
- Java.io - StringBufferInputStream
- Java.io - StringReader
- Java.io - StringWriter
- Java.io - Writer
- Java.io package extras
- Java.io - Interfaces
- Java.io - Exceptions
- Java.io package Useful Resources
- Java.io - Discussion
Java - DataInputStream read(byte[] b, int off, int len) method
Description
The Java DataInputStream read(byte[] b, int off, int len) method reads len bytes from the contained input stream and allocate them in the buffer b starting at b[off]. The method is blocked until input data is available, an exception is thrown or end of file is detected.
Declaration
Following is the declaration for java.io.DataInputStream.read(byte[] b, int off, int len) method −
public final int read(byte[] b, int off, int len)
Parameters
b − The byte[] into which the data is read from the input stream.
off − The start offset in the b[].
len − The maximum number of bytes read.
Return Value
Total number of bytes read, else -1 if the stream has reached the end.
Exception
IOException − If an I/O error occurs, the first byte cannot be read, or close() is invoked before this method.
NullPointerException − If b is null.
IndexOutOfBoundsException − If len is greater than b.length - off, off is negative, or len is negative
Example 1
The following example shows the usage of Java DataInputStream read(byte[] b, int offset, int len) method. We've created InputStream and DataInputStream references and then initialized them with FileInputStream and DataInputStream objects. In order to initialize DataInputStream(), we requires FileInputStream object. Once objects are created, we're checking if inputStream has content using available() method. Then a bytearray of available bytes is created which is then used in DataInputStream read() method, which populates the bytearray as per given input. Then this bytearray is iterated and printed.
import java.io.DataInputStream; import java.io.FileInputStream; import java.io.IOException; import java.io.InputStream; public class DataInputStreamDemo { public static void main(String[] args) throws IOException { InputStream is = null; DataInputStream dis = null; try { // create input stream from file input stream is = new FileInputStream("F:\\test.txt"); // create data input stream dis = new DataInputStream(is); // count the available bytes form the input stream int count = is.available(); // create buffer byte[] bs = new byte[count]; // read data into buffer dis.read(bs, 4, 3); // for each byte in the buffer for (byte b:bs) { // print the byte System.out.print(b+" "); } } catch(Exception e) { // if any I/O error occurs e.printStackTrace(); } finally { // releases any associated system files with this stream if(is!=null) is.close(); if(dis!=null) dis.close(); } } }
Assuming we have a text file F:/test.txt, which has the following content. This file will be used as an input for our example program −
ABCDEFGH
Output
Let us compile and run the above program, this will produce the following result −
0 0 0 0 65 66 67 0
Example 2
The following example shows the usage of Java DataInputStream read(byte[] b, int offset, int len) method. We've created InputStream and DataInputStream references and then initialized them with FileInputStream and DataInputStream objects. In order to initialize DataInputStream(), we requires FileInputStream object. Once objects are created, we're checking if inputStream has content using available() method. Then a bytearray of available bytes is created which is then used in DataInputStream read() method, which populates the bytearray with an invalid length required as input. Then this bytearray is iterated and printed.
import java.io.DataInputStream; import java.io.FileInputStream; import java.io.IOException; import java.io.InputStream; public class DataInputStreamDemo { public static void main(String[] args) throws IOException { InputStream is = null; DataInputStream dis = null; try { // create input stream from file input stream is = new FileInputStream("F:\\test.txt"); // create data input stream dis = new DataInputStream(is); // count the available bytes form the input stream int count = is.available(); // create buffer byte[] bs = new byte[count]; // read data into buffer with an invalid length dis.read(bs, 4, 10); // for each byte in the buffer for (byte b:bs) { // print the byte System.out.print(b+" "); } } catch(Exception e) { // if any I/O error occurs e.printStackTrace(); } finally { // releases any associated system files with this stream if(is!=null) is.close(); if(dis!=null) dis.close(); } } }
Assuming we have a text file F:/test.txt, which has the following content. This file will be used as an input for our example program −
ABCDEFGH
Output
Let us compile and run the above program, this will produce the following result −
java.lang.IndexOutOfBoundsException at java.base/java.io.FileInputStream.readBytes(Native Method) at java.base/java.io.FileInputStream.read(FileInputStream.java:279) at java.base/java.io.DataInputStream.read(DataInputStream.java:149) at DataInputStreamDemo.main(DataInputStreamDemo.java:25)
Example 3
The following example shows the usage of Java DataInputStream read(byte[] b, int offset, int len) method. We've created InputStream and DataInputStream references and then initialized them with FileInputStream and DataInputStream objects. In order to initialize DataInputStream(), we requires FileInputStream object. Once objects are created, we're checking if inputStream has content using available() method. Then a bytearray of available bytes is created which is then used in DataInputStream read() method, which populates the bytearray as per given input to get all the bytes of the stream. Then this bytearray is iterated and printed.
import java.io.DataInputStream; import java.io.FileInputStream; import java.io.IOException; import java.io.InputStream; public class DataInputStreamDemo { public static void main(String[] args) throws IOException { InputStream is = null; DataInputStream dis = null; try { // create input stream from file input stream is = new FileInputStream("F:\\test.txt"); // create data input stream dis = new DataInputStream(is); // count the available bytes form the input stream int count = is.available(); // create buffer byte[] bs = new byte[count]; // read data into buffer dis.read(bs, 0, count); // for each byte in the buffer for (byte b:bs) { // print the byte System.out.print(b+" "); } } catch(Exception e) { // if any I/O error occurs e.printStackTrace(); } finally { // releases any associated system files with this stream if(is!=null) is.close(); if(dis!=null) dis.close(); } } }
Assuming we have a text file F:/test.txt, which has the following content. This file will be used as an input for our example program −
ABCDEFGH
Output
Let us compile and run the above program, this will produce the following result −
65 66 67 68 69 70 71 72