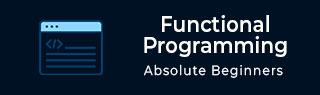
- Functional Programming Tutorial
- Home
- Introduction
- Functions Overview
- Function Types
- Call By Value
- Call By Reference
- Function Overloading
- Function Overriding
- Recursion
- Higher Order Functions
- Data Types
- Polymorphism
- Strings
- Lists
- Tuple
- Records
- Lambda Calculus
- Lazy Evaluation
- File I/O Operations
- Functional Programming Resources
- Quick Guide
- Useful Resources
- Discussion
Functional Programming - Lists
List is the most versatile data type available in functional programming languages used to store a collection of similar data items. The concept is similar to arrays in object-oriented programming. List items can be written in a square bracket separated by commas. The way to writing data into a list varies from language to language.
Program to Create a List of Numbers in Java
List is not a data type in Java/C/C++, but we have alternative ways to create a list in Java, i.e., by using ArrayList and LinkedList.
The following example shows how to create a list in Java. Here we are using a Linked List method to create a list of numbers.
import java.util.*; import java.lang.*; import java.io.*; /* Name of the class has to be "Main" only if the class is public. */ public class HelloWorld { public static void main (String[] args) throws java.lang.Exception { List<String> listStrings = new LinkedList<String>(); listStrings.add("1"); listStrings.add("2"); listStrings.add("3"); listStrings.add("4"); listStrings.add("5"); System.out.println(listStrings); } }
It will produce the following output −
[1, 2, 3, 4, 5]
Program to Create a List of Numbers in Erlang
-module(helloworld). -export([start/0]). start() -> Lst = [1,2,3,4,5], io:fwrite("~w~n",[Lst]).
It will produce the following output −
[1 2 3 4 5]
List Operations in Java
In this section, we will discuss some operations that can be done over lists in Java.
Adding Elements into a List
The methods add(Object), add(index, Object), addAll() are used to add elements into a list. For example,
ListStrings.add(3, “three”)
Removing Elements from a List
The methods remove(index) or removeobject() are used to remove elements from a list. For example,
ListStrings.remove(3,”three”)
Note − To remove all elements from the list clear() method is used.
Retrieving Elements from a List
The get() method is used to retrieve elements from a list at a specified location. The getfirst() & getlast() methods can be used in LinkedList class. For example,
String str = ListStrings.get(2)
Updating Elements in a List
The set(index,element) method is used to update an element at a specified index with a specified element. For Example,
listStrings.set(2,”to”)
Sorting Elements in a List
The methods collection.sort() and collection.reverse() are used to sort a list in ascending or descending order. For example,
Collection.sort(listStrings)
Searching Elements in a List
The following three methods are used as per the requirement −
Boolean contains(Object) method returns true if the list contains the specified element, else it returns false.
int indexOf(Object) method returns the index of the first occurrence of a specified element in a list, else it returns -1 when the element is not found.
int lastIndexOf(Object) returns the index of the last occurrence of a specified element in a list, else it returns -1 when the element is not found.
List Operations in Erlang
In this section, we will discuss some operations that can be done over lists in Erlang.
Adding two lists
The append(listfirst, listsecond) method is used to create a new list by adding two lists. For example,
append(list1,list2)
Deleting an element
The delete(element, listname) method is used to delete the specified element from the list & it returns the new list. For example,
delete(5,list1)
Deleting last element from the list
The droplast(listname) method is used to delete the last element from a list and return a new list. For example,
droplast(list1)
Searching an element
The member(element, listname) method is used to search the element into the list, if found it returns true else it returns false. For Example,
member(5,list1)
Getting maximum and minimum value
The max(listname) and min(listname) methods are used to find the maximum and minimum values in a list. For example,
max(list1)
Sorting list elements
The methods sort(listname) and reverse(listname) are used to sort a list in ascending or descending order. For example,
sort(list1)
Adding list elements
The sum(listname) method is used to add all the elements of a list and return their sum. For example,
sum(list1)
Sort a list in ascending and descending order using Java
The following program shows how to sort a list in ascending and descending order using Java −
import java.util.*; import java.lang.*; import java.io.*; public class SortList { public static void main (String[] args) throws java.lang.Exception { List<String> list1 = new ArrayList<String>(); list1.add("5"); list1.add("3"); list1.add("1"); list1.add("4"); list1.add("2"); System.out.println("list before sorting: " + list1); Collections.sort(list1); System.out.println("list in ascending order: " + list1); Collections.reverse(list1); System.out.println("list in dsending order: " + list1); } }
It will produce the following output −
list before sorting : [5, 3, 1, 4, 2] list in ascending order : [1, 2, 3, 4, 5] list in dsending order : [5, 4, 3, 2, 1]
Sort a list in ascending order using Erlang
The following program shows how to sort a list in ascending and descending order using Erlang, which is a functional programming language −
-module(helloworld). -import(lists,[sort/1]). -export([start/0]). start() -> List1 = [5,3,4,2,1], io:fwrite("~p~n",[sort(List1)]),
It will produce the following output −
[1,2,3,4,5]