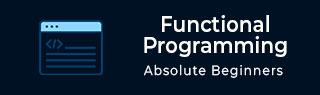
- Functional Programming Tutorial
- Home
- Introduction
- Functions Overview
- Function Types
- Call By Value
- Call By Reference
- Function Overloading
- Function Overriding
- Recursion
- Higher Order Functions
- Data Types
- Polymorphism
- Strings
- Lists
- Tuple
- Records
- Lambda Calculus
- Lazy Evaluation
- File I/O Operations
- Functional Programming Resources
- Quick Guide
- Useful Resources
- Discussion
File I/O Operations
We need files to store the output of a program when the program terminates. Using files, we can access related information using various commands in different languages.
Here is a list of some operations that can be carried out on a file −
- Creating a new file
- Opening an existing file
- Reading file contents
- Searching data on a file
- Writing into a new file
- Updating contents to an existing file
- Deleting a file
- Closing a file
Writing into a File
To write contents into a file, we will first need to open the required file. If the specified file does not exist, then a new file will be created.
Let’s see how to write contents into a file using C++.
Example
#include <iostream> #include <fstream> using namespace std; int main () { ofstream myfile; myfile.open ("Tempfile.txt", ios::out); myfile << "Writing Contents to file.\n"; cout << "Data inserted into file"; myfile.close(); return 0; }
Note −
fstream is the stream class used to control file read/write operations.
ofstream is the stream class used to write contents into file.
Let’s see how to write contents into a file using Erlang, which is a functional programming language.
-module(helloworld). -export([start/0]). start() -> {ok, File1} = file:open("Tempfile.txt", [write]), file:write(File1,"Writting contents to file"), io:fwrite("Data inserted into file\n").
Note −
To open a file we have to use, open(filename,mode).
Syntax to write contents to file: write(filemode,file_content).
Output − When we run this code “Writing contents to file” will be written into the file Tempfile.txt. If the file has any existing content, then it will be overwritten.
Reading from a File
To read from a file, first we have to open the specified file in reading mode. If the file doesn’t exist, then its respective method returns NULL.
The following program shows how to read the contents of a file in C++ −
#include <iostream> #include <fstream> #include <string> using namespace std; int main () { string readfile; ifstream myfile ("Tempfile.txt",ios::in); if (myfile.is_open()) { while ( getline (myfile,readfile) ) { cout << readfile << '\n'; } myfile.close(); } else cout << "file doesn't exist"; return 0; }
It will produce the following output −
Writing contents to file
Note − In this program, we opened a text file in read mode using “ios::in” and then print its contents on the screen. We have used while loop to read the file contents line by line by using “getline” method.
The following program shows how to perform the same operation using Erlang. Here, we will use the read_file(filename) method to read all the contents from the specified file.
-module(helloworld). -export([start/0]). start() -> rdfile = file:read_file("Tempfile.txt"), io:fwrite("~p~n",[rdfile]).
It will produce the following output −
ok, Writing contents to file
Delete an Existing File
We can delete an existing file using file operations. The following program shows how to delete an existing file using C++ −
#include <stdio.h> int main () { if(remove( "Tempfile.txt" ) != 0 ) perror( "File doesn’t exist, can’t delete" ); else puts( "file deleted successfully " ); return 0; }
It will produce the following output −
file deleted successfully
The following program shows how you can perform the same operation in Erlang. Here, we will use the method delete(filename) to delete an existing file.
-module(helloworld). -export([start/0]). start() -> file:delete("Tempfile.txt").
Output − If the file “Tempfile.txt” exists, then it will be deleted.
Determining the Size of a File
The following program shows how you can determine the size of a file using C++. Here, the function fseek sets the position indicator associated with the stream to a new position, whereas ftell returns the current position in the stream.
#include <stdio.h> int main () { FILE * checkfile; long size; checkfile = fopen ("Tempfile.txt","rb"); if (checkfile == NULL) perror ("file can’t open"); else { fseek (checkfile, 0, SEEK_END); // non-portable size = ftell (checkfile); fclose (checkfile); printf ("Size of Tempfile.txt: %ld bytes.\n",size); } return 0; }
Output − If the file “Tempfile.txt” exists, then it will show its size in bytes.
The following program shows how you can perform the same operation in Erlang. Here, we will use the method file_size(filename) to determine the size of the file.
-module(helloworld). -export([start/0]). start() -> io:fwrite("~w~n",[filelib:file_size("Tempfile.txt")]).
Output − If the file “Tempfile.txt” exists, then it will show its size in bytes. Else, it will display “0”.