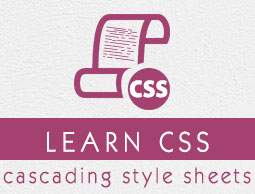
- CSS Tutorial
- CSS - Home
- CSS - Introduction
- CSS - Syntax
- CSS - Selectors
- CSS - Inclusion
- CSS - Measurement Units
- CSS - Colors
- CSS - Backgrounds
- CSS - Fonts
- CSS - Text
- CSS - Images
- CSS - Links
- CSS - Tables
- CSS - Borders
- CSS - Border Block
- CSS - Border Inline
- CSS - Margins
- CSS - Lists
- CSS - Padding
- CSS - Cursor
- CSS - Outlines
- CSS - Dimension
- CSS - Scrollbars
- CSS - Inline Block
- CSS - Dropdowns
- CSS - Visibility
- CSS - Overflow
- CSS - Clearfix
- CSS - Float
- CSS - Arrows
- CSS - Resize
- CSS - Quotes
- CSS - Order
- CSS - Position
- CSS - Hyphens
- CSS - Hover
- CSS - Display
- CSS - Focus
- CSS - Zoom
- CSS - Translate
- CSS - Height
- CSS - Hyphenate Character
- CSS - Width
- CSS - Opacity
- CSS - Z-Index
- CSS - Bottom
- CSS - Navbar
- CSS - Overlay
- CSS - Forms
- CSS - Align
- CSS - Icons
- CSS - Image Gallery
- CSS - Comments
- CSS - Loaders
- CSS - Attr Selectors
- CSS - Combinators
- CSS - Root
- CSS - Box Model
- CSS - Counters
- CSS - Clip
- CSS - Writing Mode
- CSS - Unicode-bidi
- CSS - min-content
- CSS - All
- CSS - Inset
- CSS - Isolation
- CSS - Overscroll
- CSS - Justify Items
- CSS - Justify Self
- CSS - Tab Size
- CSS - Pointer Events
- CSS - Place Content
- CSS - Place Items
- CSS - Place Self
- CSS - Max Block Size
- CSS - Min Block Size
- CSS - Mix Blend Mode
- CSS - Max Inline Size
- CSS - Min Inline Size
- CSS - Offset
- CSS - Accent Color
- CSS - User Select
- CSS Advanced
- CSS - Grid
- CSS - Grid Layout
- CSS - Flexbox
- CSS - Visibility
- CSS - Positioning
- CSS - Layers
- CSS - Pseudo Classes
- CSS - Pseudo Elements
- CSS - @ Rules
- CSS - Text Effects
- CSS - Paged Media
- CSS - Printing
- CSS - Layouts
- CSS - Validations
- CSS - Image Sprites
- CSS - Important
- CSS - Data Types
- CSS3 Tutorial
- CSS3 - Tutorial
- CSS - Rounded Corner
- CSS - Border Images
- CSS - Multi Background
- CSS - Color
- CSS - Gradients
- CSS - Box Shadow
- CSS - Box Decoration Break
- CSS - Caret Color
- CSS - Text Shadow
- CSS - Text
- CSS - 2d transform
- CSS - 3d transform
- CSS - Transition
- CSS - Animation
- CSS - Multi columns
- CSS - Box Sizing
- CSS - Tooltips
- CSS - Buttons
- CSS - Pagination
- CSS - Variables
- CSS - Media Queries
- CSS - Functions
- CSS - Math Functions
- CSS - Masking
- CSS - Shapes
- CSS - Style Images
- CSS - Specificity
- CSS - Custom Properties
- CSS Responsive
- CSS RWD - Introduction
- CSS RWD - Viewport
- CSS RWD - Grid View
- CSS RWD - Media Queries
- CSS RWD - Images
- CSS RWD - Videos
- CSS RWD - Frameworks
- CSS References
- CSS - Questions and Answers
- CSS - Quick Guide
- CSS - References
- CSS - Color References
- CSS - Web browser References
- CSS - Web safe fonts
- CSS - Units
- CSS - Animation
- CSS Resources
- CSS - Useful Resources
- CSS - Discussion
CSS grid - grid-auto-flow Property
The CSS property grid-auto-flow manages the behavior of the auto-placement algorithm and determines the exact way in which the items intended for auto-placement are arranged in the grid.
You can express this property in one of two ways.
A single keyword, choosing from either row, column, or dense.
A combination of two keywords: either row dense or column dense.
Possible Values
row - The objects are positioned by sequentially filling each row and creating new rows as required. If neither a row nor a column is specified, a row is assumed by default.
column - Objects are positioned by filling each column sequentially, with additional columns being created if required.
dense - With the dense packing method, empty spaces in the grid are filled with priority, which can lead to items appearing out of sequence to fill gaps created by larger items.
If this is omitted, the sparse algorithm is used. This only moves forward when placing the elements, and maintaining the order, even if this results in empty spaces that could have been filled later.
Syntax
grid-auto-flow = [ row | column ] || dense
Applies to
Grid containers.
CSS grid-auto-flow - Basic Example
The following example demonstrates the use of grid-auto-flow
The CSS property grid-auto-flow is set to column and determines the direction in which the grid items are automatically placed within the grid container.
This property controls the order of placement of additional elements that don't have a fixed position by first filling the columns before the next elements are inserted.
To change this behavior, you can change the value of grid-auto-flow to row for a row-based placement strategy or to dense for a more compact filling of the available space, either in rows or in columns.
<html> <head> <style> .grid-container { display: grid; grid-template-columns: repeat(3, 150px); grid-template-rows: repeat(3, 150px); gap: 15px; grid-auto-flow: column; /* Change this property to see different effects */ } .item:nth-child(1) { background-color: #3498db; } .item:nth-child(2) { background-color: #27ae60; } .item:nth-child(3) { background-color: #e74c3c; } .item:nth-child(4) { background-color: #f39c12; } .item:nth-child(5) { background-color: #9b59b6; } .item:nth-child(6) { background-color: #2c3e50; } .item:nth-child(7) { background-color: #1abc9c; } .item:nth-child(8) { background-color: #34495e; } .item:nth-child(9) { background-color: #e67e22; } .item { color: white; display: flex; align-items: center; justify-content: center; font-size: 50px; } </style> </head> <body> <div class="grid-container"> <div class="item">1</div> <div class="item">2</div> <div class="item">3</div> <div class="item">4</div> <div class="item">5</div> <div class="item">6</div> <div class="item">7</div> <div class="item">8</div> <div class="item">9</div> </div> </body> </html>
CSS grid-auto-flow - row Value
In the following example, the auto-placement technique for grid items is specified by the CSS rule grid-auto-flow: row;, which makes sure that the items are arranged in rows from top to bottom and left to right, filling each row before going on to the next.
This shows how the grid-auto-flow property can be used to regulate the grid layout.
<html> <head> <style> body { background-color: #F2F2F2; font-family: 'Arial', sans-serif; margin: 0; padding: 0; } #customDIV { height: 300px; display: grid; gap: 10px; background-color: #49a4e6; padding: 10px; grid-auto-flow: row; } #customDIV div { background-color: rgba(255, 255, 255, 1); border-radius: 15px; text-align: center; padding: 20px 0; font-size: 30px; } .customItem3 { grid-row: span 2; grid-column: span 2; } </style> </head> <body> <h1>Grid Layout with grid-auto-flow</h1> <div id="customDIV"> <div class="customItem1">Alpha</div> <div class="customItem2">Beta</div> <div class="customItem3">Gamma</div> <div class="customItem4">Delta</div> </div> </body> </html>
CSS grid-auto-flow - column Value
In the following example, the auto-placement technique for grid items is specified by the CSS rule grid-auto-flow: column;, which ensures that the items are arranged in columns from left to right and top to bottom, filling each column before moving on to the next.
<html> <head> <style> body { background-color: #F2F2F2; font-family: 'Arial', sans-serif; margin: 0; padding: 0; } #customDIV { width: 400px; display: grid; gap: 10px; background-color: #7fc6bc; padding: 10px; grid-auto-flow: column; } #customDIV div { background-color: rgba(255, 255, 255, 1); border-radius: 5px; text-align: center; padding: 20px 0; font-size: 30px; } .customItem1 { grid-column: span 2; } .customItem3 { grid-row: span 2; } </style> </head> <body> <h1>Grid Layout with grid-auto-flow: column;</h1> <div id="customDIV"> <div class="customItem1">Element Alpha</div> <div class="customItem2">Element Beta</div> <div class="customItem3">Element Gamma</div> <div class="customItem4">Element Delta</div> </div> </body> </html>
CSS grid-auto-flow - dense Value
In the following example, the grid is set to arrange items using grid-auto-flow: dense;. This means that the items will be tightly packed, filling empty spaces intelligently and maintaining their original order in the code.
<html> <head> <style> body { background-color: #E0E0E0; font-family: 'Verdana', sans-serif; margin: 0; padding: 0; } #customDIV { width: 400px; display: grid; gap: 10px; background-color: #9B59B6; padding: 10px; grid-auto-flow: dense; } #customDIV div { background-color: rgba(255, 255, 255, 0.8); border-radius: 8px; text-align: center; padding: 15px 0; font-size: 24px; } .customItem1 { grid-column: span 2; } .customItem3 { grid-row: span 2; } </style> </head> <body> <h1>Grid Layout with grid-auto-flow: dense;</h1> <div id="customDIV"> <div class="customItem1">Alpha</div> <div class="customItem2">Beta</div> <div class="customItem3">Gamma</div> <div class="customItem4">Delta</div> </div> </body> </html>
CSS grid-auto-flow - row dense Value
In the following example, the grid is set to arrange items using grid-auto-flow: row dense;. This means that the grid arranges items in rows, compactly filling available spaces and maintaining the specified item order.
<html> <head> <style> body { background-color: #EAEAEA; font-family: 'Courier New', monospace; margin: 0; padding: 0; } #customDIV { width: 400px; display: grid; gap: 10px; background-color: #3498DB; padding: 10px; grid-auto-flow: row dense; } #customDIV div { background-color: rgba(255, 255, 255, 0.9); border-radius: 10px; text-align: center; padding: 15px 0; font-size: 20px; } .customItem1 { grid-column: span 2; } .customItem3 { grid-row: span 2; } </style> </head> <body> <h1>Grid Layout with grid-auto-flow: row dense;</h1> <div id="customDIV"> <div class="customItem1">Element Alpha</div> <div class="customItem2">Element Beta</div> <div class="customItem3">Element Gamma</div> <div class="customItem4">Element Delta</div> <div class="customItem5">Theta Element</div> <div class="customItem6">Lambda Element</div> </div> </body> </html>
CSS grid-auto-flow - column dense Value
In the following example, the grid is set to arrange items using grid-auto-flow: column dense;. This means that the grid organizes items in columns, cleverly filling gaps to create a condensed layout while keeping the original order of items intact.
<html> <head> <style> body { background-color: #F8F8F8; font-family: 'Georgia', serif; margin: 0; padding: 0; } #customDIV { width: 400px; display: grid; gap: 10px; background-color: #FF6347; padding: 10px; grid-auto-flow: column dense; } #customDIV div { background-color: rgba(255, 255, 255, 0.8); border-radius: 12px; text-align: center; padding: 15px 0; font-size: 18px; } .customItem1 { grid-row: span 2; } .customItem3 { grid-column: span 2; } </style> </head> <body> <h1>Grid Layout with grid-auto-flow: column dense;</h1> <div id="customDIV"> <div class="customItem1">Alpha Element</div> <div class="customItem2">Beta Element</div> <div class="customItem3">Gamma Element</div> <div class="customItem4">Delta Element</div> <div class="customItem5">Theta Element</div> <div class="customItem6">Lambda Element</div> </div> </body> </html>
CSS grid-auto-flow - Configuring Automatic Grid Placement
In the following example there is a grid layout that allows users to change between row, column, and dense configurations for the grid elements.
The grid-auto-flow property changes dynamically based on the choice picked from a dropdown menu.
<html> <head> <style> body { background-color: #f2dfaa; font-family: 'Arial', sans-serif; margin: 0; padding: 0; } #customDIV { height: 300px; display: grid; gap: 10px; background-color: #fcba03; padding: 10px; } #customDIV div { background-color: rgba(255, 255, 255, 0.9); text-align: center; padding: 20px 0; font-size: 30px; } .customItem3 { grid-row: span 2; grid-column: span 2; } select { margin: 10px; padding: 5px; font-size: 16px; } </style> </head> <body> <h1>Grid Layout with grid-auto-flow</h1> <label for="gridAutoFlow">Change grid-auto-flow: </label> <select id="gridAutoFlow" onchange="changeGridAutoFlow()"> <option value="row">Row</option> <option value="column">Column</option> <option value="dense">Dense</option> </select> <div id="customDIV"> <div class="customItem1">BOX - A</div> <div class="customItem2">BOX - B</div> <div class="customItem3">BOX - C</div> <div class="customItem4">BOX - D</div> </div> <script> function changeGridAutoFlow() { var selectedValue = document.getElementById("gridAutoFlow").value; document.getElementById("customDIV").style.gridAutoFlow = selectedValue; } </script> </body> </html>