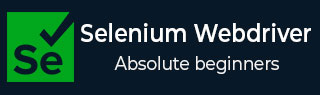
- Selenium Webdriver Tutorial
- Home
- Introduction
- Installation
- Browser Navigation
- Identify Single Element
- Identify Multiple Elements
- Explicit and Implicit Wait
- Pop-ups
- Backward and Forward Navigation
- Cookies
- Exceptions
- Action Class
- Create a Basic Test
- Forms
- Drag and Drop
- Windows
- Alerts
- Handling Links
- Handling Edit Boxes
- Color Support
- Generating HTML Test Reports in Python
- Read/Write data from Excel
- Handling Checkboxes
- Executing Tests in Multiple Browsers
- Headless Execution
- Wait Support
- Select Support
- JavaScript Executor
- Chrome WebDriver Options
- Scroll Operations
- Capture Screenshots
- Right Click
- Double Click
- Selenium Webdriver Useful Resources
- Selenium WebDriver - Quick Guide
- Selenium WebDriver - Useful Resources
- Selenium WebDriver - Discussion
Selenium Webdriver - Identify Single Element
Once we navigate to a webpage, we have to interact with the web elements available on the page like clicking a link/button, entering text within an edit box, and so on to complete our automation test case.
By Id
For this, our first job is to identify the element. We can use the id attribute for an element for its identification and utilize the method find_element_by_id. With this, the first element with the matching value of the attribute id is returned.
In case there is no element with the matching value of the id attribute, NoSuchElementException shall be thrown.
The syntax for identifying an element is as follows −
driver.find_element_by_id("value of id attribute")
Let us see the html code of a web element −
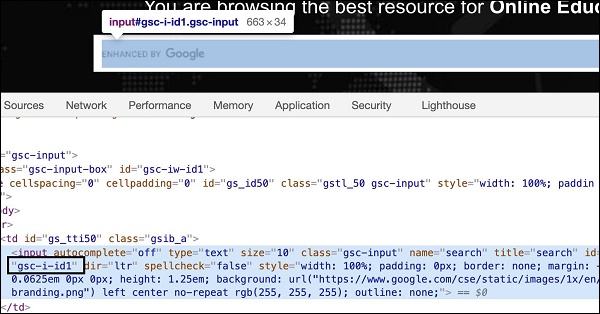
The edit box highlighted in the above image has an id attribute with value gsc-i-id1. Let us try to input some text into this edit box after identifying it.
Code Implementation
The code implementation of identifying a web element is as follows −
from selenium import webdriver #set chromedriver.exe path driver = webdriver.Chrome(executable_path='../drivers/chromedriver') #url launch driver.get("https://www.tutorialspoint.com/index.htm") #identify edit box with id l = driver.find_element_by_id('gsc-i-id1') #input text l.send_keys('Selenium') #obtain value entered v = l.get_attribute('value') print('Value entered: ' + v) #driver quit driver.quit()
Output

The output shows the message - Process with exit code 0 meaning that the above Python code executed successfully. Also, the value entered within the edit box (obtained from the get_attribute method) - Selenium gets printed in the console.
By Name
Once we navigate to a webpage, we have to interact with the web elements available on the page like clicking a link/button, entering text within an edit box, and so on to complete our automation test case.
For this, our first job is to identify the element. We can use the name attribute for an element for its identification and utilize the method find_element_by_name. With this, the first element with the matching value of the attribute name is returned.
In case there is no element with the matching value of the name attribute, NoSuchElementException shall be thrown.
The syntax for identifying single element by name is as follows:
driver.find_element_by_name("value of name attribute")
Let us see the html code of a web element as given below −
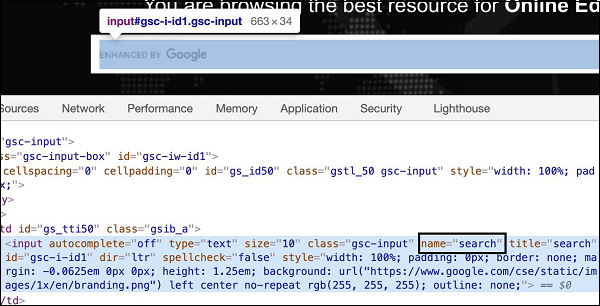
The edit box highlighted in the above image has a name attribute with value search. Let us try to input some text into this edit box after identifying it.
Code Implementation
The code implementation of identifying single element by name is as follows −
from selenium import webdriver #set chromedriver.exe path driver = webdriver.Chrome(executable_path='../drivers/chromedriver') #url launch driver.get("https://www.tutorialspoint.com/index.htm") #identify edit box with name l = driver.find_element_by_name('search') #input text l.send_keys('Selenium Java') #obtain value entered v = l.get_attribute('value') print('Value entered: ' + v) #driver close driver.close()
Output

The output shows the message - Process with exit code 0 meaning that the above Python code executed successfully. Also, the value entered within the edit box (obtained from the get_attribute method) - Selenium Java gets printed in the console.
By ClassName
Once we navigate to a webpage, we have to interact with the web elements available on the page like clicking a link/button, entering text within an edit box, and so on to complete our automation test case.
For this, our first job is to identify the element. We can use the class attribute for an element for its identification and utilise the method find_element_by_class_name. With this, the first element with the matching value of the attribute class is returned.
In case there is no element with the matching value of the class attribute, NoSuchElementException shall be thrown.
The syntax for identifying single element by Classname is as follows :
driver.find_element_by_class_name("value of class attribute")
Let us see the html code of a web element as given below −
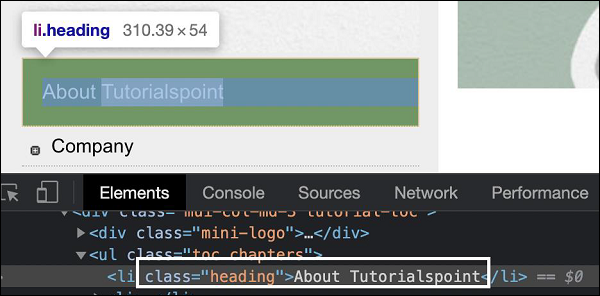
The web element highlighted in the above image has a class attribute with value heading. Let us try to obtain the text of that element after identifying it.
Code Implementation
The code implementation of identifying single element by Classname is as follows −
from selenium import webdriver #set chromedriver.exe path driver = webdriver.Chrome(executable_path='../drivers/chromedriver') #url launch driver.get("https://www.tutorialspoint.com/about/about_careers.htm") #identify edit box with class l = driver.find_element_by_class_name('heading') #identify text v = l.text #text obtained print('Text is: ' + v) #driver close driver.close()
Output

The output shows the message - Process with exit code 0 meaning that the above Python code executed successfully. Also, the text of the webelement (obtained from the text method) - About Tutorialspoint gets printed in the console.
By TagName
Once we navigate to a webpage, we have to interact with the webelements available on the page like clicking a link/button, entering text within an edit box, and so on to complete our automation test case.
For this, our first job is to identify the element. We can use the tagname for an element for its identification and utilise the method find_element_by_tag_name. With this, the first element with the matching tagname is returned.
In case there is no element with the matching tagname, NoSuchElementException shall be thrown.
The syntax for identifying single element by Tagname is as follows:
driver.find_element_by_tag_name("tagname of element")
Let us see the html code of a web element as given below −
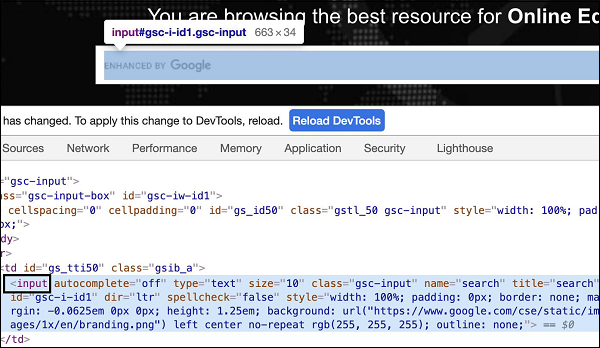
The edit box highlighted in the above image has a tagname - input. Let us try to input some text into this edit box after identifying it.
Code Implementation
The code implementation of identifying single element by Tagname is as follows −
from selenium import webdriver #set chromedriver.exe path driver = webdriver.Chrome(executable_path='../drivers/chromedriver') #url launch driver.get("https://www.tutorialspoint.com/index.htm") #identify edit box with tagname l = driver.find_element_by_tag_name('input') #input text l.send_keys('Selenium Python') #obtain value entered v = l.get_attribute('value') print('Value entered: ' + v) #driver close driver.close()
Output

The output shows the message - Process with exit code 0 meaning that the above Python code executed successfully. Also, the value entered within the edit box (obtained from the get_attribute method) - Selenium Python gets printed in the console.
By Link Text
Once we navigate to a webpage, we may interact with a webelement by clicking a link to complete our automation test case. The link text is used for an element having the anchor tag.
For this, our first job is to identify the element. We can use the link text attribute for an element for its identification and utilize the method find_element_by_link_text. With this, the first element with the matching value of the given link text is returned.
In case there is no element with the matching value of the link text, NoSuchElementException shall be thrown.
The syntax for identifying single element by Link Text is as follows:
driver.find_element_by_link_text("value of link text")
Let us see the html code of a web element as given below −
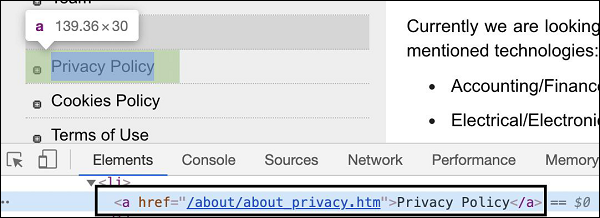
The link highlighted in the above image has a tagname - a and the link text - Privacy Policy. Let us try to click on this link after identifying it.
Code Implementation
The code implementation of identifying single element by Link Text is as follows −
from selenium import webdriver driver = webdriver.Chrome(executable_path='../drivers/chromedriver') #url launch driver.get("https://www.tutorialspoint.com/about/about_careers.htm") #identify link with link text l = driver.find_element_by_link_text('Privacy Policy') #perform click l.click() print('Page navigated after click: ' + driver.title) #driver quit driver.quit()
Output

The output shows the message - Process with exit code 0 meaning that the above Python code executed successfully. Also, the page title of the application (obtained from the driver.title method) - About Privacy Policy at Tutorials Point - Tutorialspoint gets printed in the console.
By Partial Link Text
Once we navigate to a webpage, we may interact with a web element by clicking a link to complete our automation test case. The partial link text is used for an element having the anchor tag.
For this, our first job is to identify the element. We can use the partial link text attribute for an element for its identification and utilize the method find_element_by_partial_link_text. With this, the first element with the matching value of the given partial link text is returned.
In case there is no element with the matching value of the partial link text, NoSuchElementException shall be thrown.
The syntax for identifying single element by Partial Link Text is as follows −
driver.find_element_by_partial_link_text("value of partial ink text")
Let us see the html code of a web element as given below −
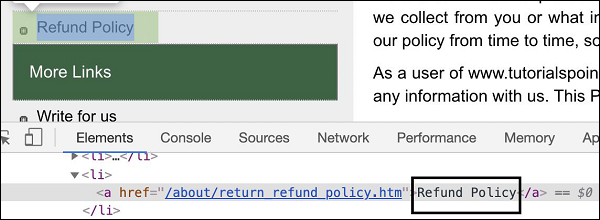
The link highlighted in the above image has a tagname - a and the partial link text - Refund. Let us try to click on this link after identifying it.
Code Implementation
The code implementation for identifying single element by Partial Link Text is as follows −
from selenium import webdriver driver = webdriver.Chrome(executable_path='../drivers/chromedriver') #url launch driver.get("https://www.tutorialspoint.com/about/about_careers.htm") #identify link with partial link text l = driver.find_element_by_partial_link_text('Refund') #perform click l.click() print('Page navigated after click: ' + driver.title) #driver quit driver.quit()
Output

The output shows the message - Process with exit code 0 meaning that the above Python code executed successfully. Also, the page title of the application (obtained from the driver.title method) - Return, Refund & Cancellation Policy - Tutorialspoint gets printed in the console.
By CSS Selector
Once we navigate to a webpage, we have to interact with the webelements available on the page like clicking a link/button, entering text within an edit box, and so on to complete our automation test case.
For this, our first job is to identify the element. We can create a css selector for an element for its identification and use the method find_element_by_css_selector. With this, the first element with the matching value of the given css is returned.
In case there is no element with the matching value of the css, NoSuchElementException shall be thrown.
The syntax for identifying single element by CSS Selector is as follows −
driver.find_element_by_css_selector("value of css")
Rules to create CSS Expression
The rules to create a css expression are discussed below
To identify the element with css, the expression should be tagname[attribute='value']. We can also specifically use the id attribute to create a css expression.
With id, the format of a css expression should be tagname#id. For example, input#txt [here input is the tagname and the txt is the value of the id attribute].
With class, the format of css expression should be tagname.class. For example, input.cls-txt [here input is the tagname and the cls-txt is the value of the class attribute].
If there are n children of a parent element, and we want to identify the nth child, the css expression should have nth-of –type(n).
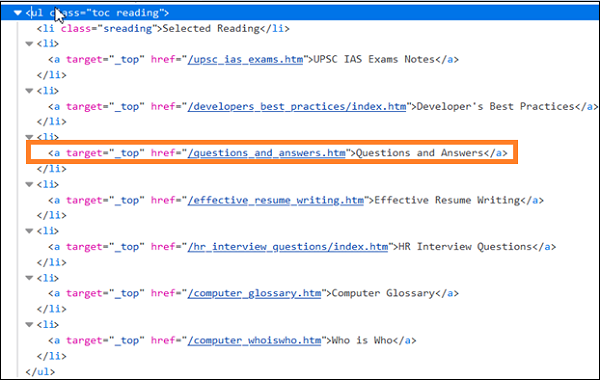
In the above code, if we want to identify the fourth li childof ul[Questions and Answers], the css expression should be ul.reading li:nth-of-type(4). Similarly, to identify the last child, the css expression should be ul.reading li:last-child.
For attributes whose values are dynamically changing, we can use ^= to locate an element whose attribute value starts with a particular text. For example, input[name^='qa'] Here, input is the tagname and the value of the name attribute starts with qa.
For attributes whose values are dynamically changing, we can use $ = to locate an element whose attribute value ends with a particular text. For example, input[class $ ='txt'] Here, input is the tagname and the value of the class attribute ends with txt.
For attributes whose values are dynamically changing, we can use *= to locate an element whose attribute value contains a specific sub-text. For example, input[name*='nam'] Here, input is the tagname and the value of the name attribute contains the sub-text nam.
Let us see the html code of a web element as given below −
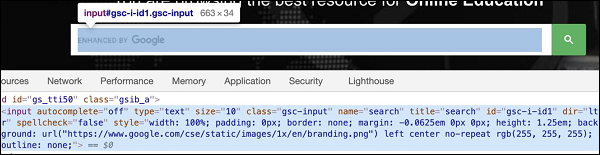
The edit box highlighted in the above image has a name attribute with value search, the css expression should be input[name='search']. Let us try to input some text into this edit box after identifying it.
Code Implementation
The code implementation of identifying single element by CSS Selector is as follows −
from selenium import webdriver driver = webdriver.Chrome(executable_path='../drivers/chromedriver') #implicit wait time driver.implicitly_wait(5) #url launch driver.get("https://www.tutorialspoint.com/index.htm") #identify element with css l = driver.find_element_by_css_selector("input[name='search']") l.send_keys('Selenium Python') v = l.get_attribute('value') print('Value entered is: ' + v) #driver quit driver.quit()
Output

The output shows the message - Process with exit code 0 meaning that the above Python code executed successfully. Also, the value entered within the edit box (obtained from the get_attribute method) - Selenium Python gets printed in the console.
ByXpath
Once we navigate to a webpage, we have to interact with the webelements available on the page like clicking a link/button, entering text within an edit box, and so on to complete our automation test case.
For this, our first job is to identify the element. We can create an xpath for an element for its identification and use the method find_element_by_xpath. With this, the first element with the matching value of the given xpath is returned.
In case there is no element with the matching value of the xpath, NoSuchElementException shall be thrown.
The syntax for identifying single element by Xpath is as follows −
driver.find_element_by_xpath("value of xpath")
Rules to create Xpath Expression
The rules to create a xpath expression are discussed below −
To identify the element with xpath, the expression should be //tagname[@attribute='value']. There can be two types of xpath – relative and absolute. The absolute xpath begins with / symbol and starts from the root node upto the element that we want to identify.
For example,
/html/body/div[1]/div/div[1]/a
The relative xpath begins with // symbol and does not start from the root node.
For example,
//img[@alt='tutorialspoint']
Let us see the html code of the highlighted link - Home starting from the root.
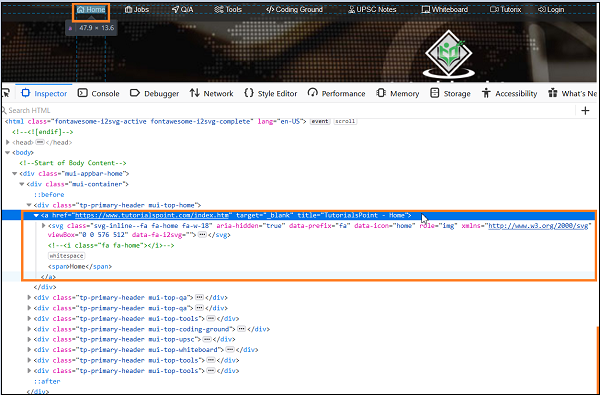
The absolute xpath for this element can be as follows −
/html/body/div[1]/div/div[1]/a.
The relative xpath for element Home can be as follows −
//a[@title='TutorialsPoint - Home'].
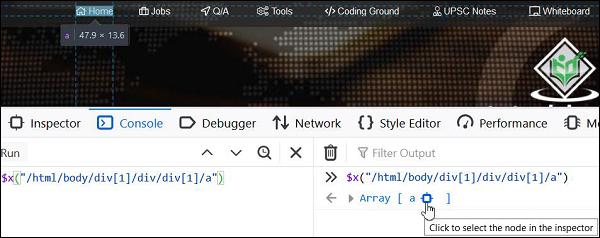
Functions
There are also functions available which help to frame relative xpath expressions.
text()
It is used to identify an element with its visible text on the page. The xpath expression is as follows −
//*[text()='Home'].
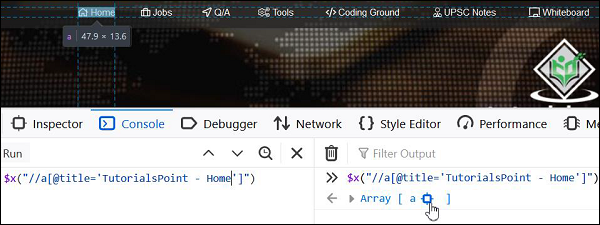
starts-with
It is used to identify an element whose attribute value begins with a specific text. This function is normally used for attributes whose value changes on each page load.
Let us see the html of the link Q/A −
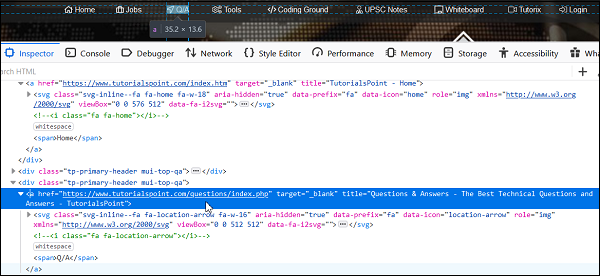
The xpath expression should be as follows:
//a[starts-with(@title, 'Questions &')].
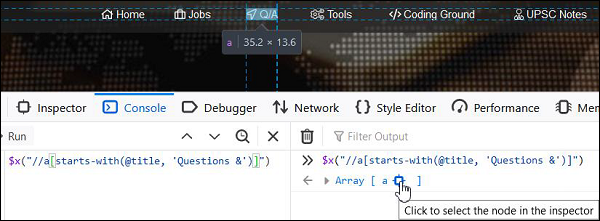
contains()
It identifies an element whose attribute value contains a sub-text. This function is normally used for attributes whose value changes on each page load.
The xpath expression is as follows −
//a[contains(@title, 'Questions & Answers')].
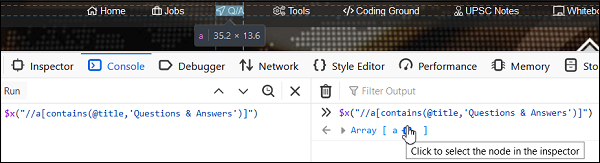
Let us see the html code of a webelement as shown below −
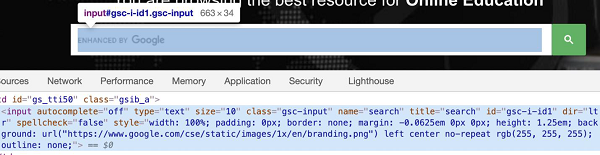
The edit box highlighted in the above image has a name attribute with value search, the xpath expression should be //input[@name='search']. Let us try to input some text into this edit box after identifying it.
Code Implementation
The code implementation of identifying single element by XPath is as follows −
from selenium import webdriver driver = webdriver.Chrome(executable_path='../drivers/chromedriver') #implicit wait time driver.implicitly_wait(5) #url launch driver.get("https://www.tutorialspoint.com/index.htm") #identify element with xpath l = driver.find_element_by_xpath("//input[@name='search']") l.send_keys('Selenium Python') v = l.get_attribute('value') print('Value entered is: ' + v) #driver quit driver.quit()
Output

The output shows the message - Process with exit code 0 meaning that the above Python code executed successfully. Also, the value entered within the edit box (obtained from the get_attribute method) - Selenium Python gets printed in the console.