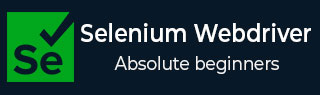
- Selenium Webdriver Tutorial
- Home
- Introduction
- Installation
- Browser Navigation
- Identify Single Element
- Identify Multiple Elements
- Explicit and Implicit Wait
- Pop-ups
- Backward and Forward Navigation
- Cookies
- Exceptions
- Action Class
- Create a Basic Test
- Forms
- Drag and Drop
- Windows
- Alerts
- Handling Links
- Handling Edit Boxes
- Color Support
- Generating HTML Test Reports in Python
- Read/Write data from Excel
- Handling Checkboxes
- Executing Tests in Multiple Browsers
- Headless Execution
- Wait Support
- Select Support
- JavaScript Executor
- Chrome WebDriver Options
- Scroll Operations
- Capture Screenshots
- Right Click
- Double Click
- Selenium Webdriver Useful Resources
- Selenium WebDriver - Quick Guide
- Selenium WebDriver - Useful Resources
- Selenium WebDriver - Discussion
Selenium Webdriver - Alerts
Selenium webdriver is capable of handling Alerts. The class selenium.webdriver.common.alert.Alert(driver) is used to work with Alerts. It has methods to accept, dismiss, enter and obtain the Alert text.
Methods
The methods under the Alert class are listed below −
accept() − For accepting an Alert.
dismiss() − For dismissing an Alert.
text() − For obtaining Alert text.
send_keys(keysToSend) − For entering text in Alert.
Code Implementation
The code implementation for alerts is as follows −
from selenium import webdriver #import Alert class from selenium.webdriver.common.alert import Alert driver = webdriver.Chrome(executable_path='../drivers/chromedriver') #implicit wait time driver.implicitly_wait(0.8) #url launch driver.get("https://the-internet.herokuapp.com/javascript_alerts") #identify element l = driver.find_element_by_xpath("//*[text()='Click for JS Prompt']") l.click() # instance of Alert class a = Alert(driver) # get alert text print(a.text) #input text in Alert a.send_keys('Tutorialspoint') #dismiss alert a.dismiss() l.click() #accept alert a.accept() #driver quit driver.quit()
Output

The output shows the message - Process with exit code 0 meaning that the above Python code executed successfully. Also, the Alert text - I am a JS prompt gets printed in the console.
Advertisements