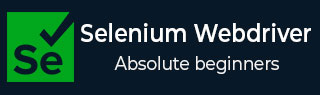
- Selenium Webdriver Tutorial
- Home
- Introduction
- Installation
- Browser Navigation
- Identify Single Element
- Identify Multiple Elements
- Explicit and Implicit Wait
- Pop-ups
- Backward and Forward Navigation
- Cookies
- Exceptions
- Action Class
- Create a Basic Test
- Forms
- Drag and Drop
- Windows
- Alerts
- Handling Links
- Handling Edit Boxes
- Color Support
- Generating HTML Test Reports in Python
- Read/Write data from Excel
- Handling Checkboxes
- Executing Tests in Multiple Browsers
- Headless Execution
- Wait Support
- Select Support
- JavaScript Executor
- Chrome WebDriver Options
- Scroll Operations
- Capture Screenshots
- Right Click
- Double Click
- Selenium Webdriver Useful Resources
- Selenium WebDriver - Quick Guide
- Selenium WebDriver - Useful Resources
- Selenium WebDriver - Discussion
Selenium Webdriver - Identify Multiple Elements
In this chapter, we will learn how to identify multiple elements by various options. Let us begin by understanding identifying multiple elements by Id.
By id
It is not recommended to identify multiple elements by the locator id, since the value of an id attribute is unique to an element and is applicable to a single element on the page.
By Class name
Once we navigate to a webpage, we have to interact with the webelements available on the page like clicking a link/button, entering text within an edit box, and so on to complete our automation test case.
For this, our first job is to identify the elements. We can use the class attribute for elements for their identification and utilise the method find_elements_by_class_name. With this, all the elements with the matching value of the attribute class are returned in the form of list.
In case there are no elements with the matching value of the class attribute, an empty list shall be returned.
The syntax for identifying multiple elements by Classname is as follows −
driver.find_elements_by_class_name("value of class attribute")
Let us see the html code of webelements having class attribute as given below −

The value of the class attribute highlighted in the above image is toc chapters. Let us try to count the number of such webelements.
Code Implementation
The code implementation for identifying multiple elements by Classname is as follows −
from selenium import webdriver driver = webdriver.Chrome(executable_path='../drivers/chromedriver') #implicit wait time driver.implicitly_wait(5) #url launch driver.get("https://www.tutorialspoint.com/about/about_careers.htm") #identify elements with class attribute l = driver.find_elements_by_class_name("chapters") #count elements s = len(l) print('Count is:') print(s) #driver quit driver.quit()
Output
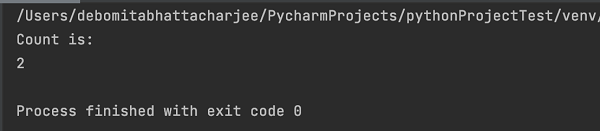
The output shows the message - Process with exit code 0 meaning that the above Python code executed successfully. Also, the total count of webelements having the class attributes value chapters (obtained from the len method) - 2 gets printed in the console.
By Tagname
Once we navigate to a webpage, we have to interact with the webelements available on the page like clicking a link/button, entering text within an edit box, and so on to complete our automation test case.
For this, our first job is to identify the elements. We can use the tagname for elements for their identification and utilise the method find_elements_by_tag_name. With this, all the elements with the matching value of the tagname are returned in the form of list.
In case there are no elements with the matching value of the tagname, an empty list shall be returned.
The syntax for identifying multiple elements by Tagname is as follows −
driver.find_elements_by_tag_name("value of tagname")
Let us see the html code of a webelement, which is as follows −
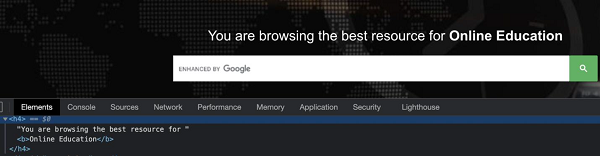
The value of the tagname highlighted in the above image is h4. Let us try to count the number of webelements having tagname as h4.
Code Implementation
The code implementation for identifying multiple elements by Tagname is as follows −
from selenium import webdriver driver = webdriver.Chrome(executable_path='../drivers/chromedriver') #implicit wait time driver.implicitly_wait(5) #url launch driver.get("https://www.tutorialspoint.com/index.htm") #identify elements with tagname l = driver.find_elements_by_tag_name("h4") #count elements s = len(l) print('Count is:') print(s) #driver quit driver.quit()
Output
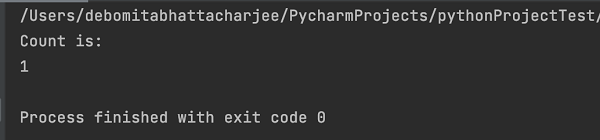
The output shows the message - Process with exit code 0 meaning that the above Python code executed successfully. Also, the total count of webelement having the tagname as h4 (obtained from the len method) - 1 gets printed in the console.
By Partial Link Text
Once we navigate to a webpage, we may have to interact with the webelements by clicking a link to complete our automation test case. The partial link text is used for elements having the anchor tag.
For this, our first job is to identify the elements. We can use the partial link text attribute for elements for their identification and utlize the method find_elements_by_partial_link_text. With this, all the elements with the matching value of the given partial link text are returned in the form of a list.
In case there are no elements with the matching value of the partial link text, an empty list shall be returned.
The syntax for identifying multiple elements by Partial Link Text is as follows −
driver.find_elements_by_partial_link_text("value of partial link text")
Let us see the html code of link, which is as follows −
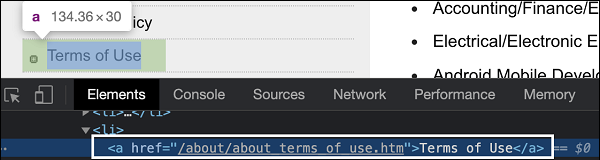
The link highlighted - Terms of Use in the above image has a tagname - a and the partial link text - Terms. Let us try to identify the text after identifying it.
Code Implementation
The code implementation for identifying multiple elements by Partial Link Text is as follows −
from selenium import webdriver driver = webdriver.Chrome(executable_path='../drivers/chromedriver') #implicit wait time driver.implicitly_wait(5) #url launch driver.get("https://www.tutorialspoint.com/about/about_careers.htm") #identify elements with partial link text l = driver.find_elements_by_partial_link_text('Terms') #count elements s = len(l) #iterate through list for i in l: #obtain text t = i.text print('Text is: ' + t) #driver quit driver.quit()
Output

The output shows the message - Process with exit code 0 meaning that the above Python code executed successfully. Also, the text of the link identified with the partial link text locator (obtained from the text method) - Terms of use gets printed in the console.
By Link Text
Once we navigate to a webpage, we may have to interact with the webelements by clicking a link to complete our automation test case. The link text is used for elements having the anchor tag.
For this, our first job is to identify the elements. We can use the link text attribute for elements for their identification and utilize the method find_elements_by_link_text. With this, all the elements with the matching value of the given link text are returned in the form of a list.
In case there are no elements with the matching value of the link text, an empty list shall be returned.
The syntax for identifying multiple elements by Link Text is as follows −
driver.find_elements_by_link_text("value of link text")
Let us see the html code of link, which is as follows −
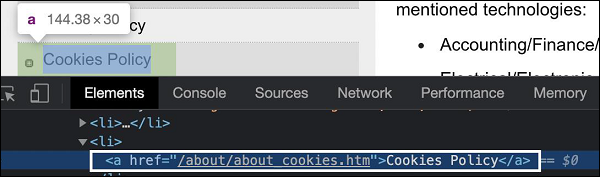
The link highlighted - Cookies Policy in the above image has a tagname - a and the link text - Cookies Policy. Let us try to identify the text after identifying it.
Code Implementation
The code implementation for identifying multiple elements by Link Text is as follows −
from selenium import webdriver driver = webdriver.Chrome(executable_path='../drivers/chromedriver') #implicit wait time driver.implicitly_wait(5) #url launch driver.get("https://www.tutorialspoint.com/about/about_careers.htm") #identify elements with link text l = driver.find_elements_by_link_text('Cookies Policy') #count elements s = len(l) #iterate through list for i in l: #obtain text t = i.text print('Text is: ' + t) #driver quit driver.quit()
Output

The output shows the message - Process with exit code 0 meaning that the above Python code executed successfully. Also, the text of the link identified with the link text locator (obtained from the text method) - Cookies Policy gets printed in the console.
By Name
Once we navigate to a webpage, we have to interact with the webelements available on the page like clicking a link/button, entering text within an edit box, and so on to complete our automation test case.
For this, our first job is to identify the elements. We can use the name attribute of elements for their identification and utilize the method find_elements_by_name. With this, the elements with the matching value of the attribute name are returned in the form of a list.
In case there is no element with the matching value of the name attribute, an empty list shall be returned.
The syntax for identifying multiple elements by Name is as follows −
driver.find_elements_by_name("value of name attribute")
Let us see the html code of an webelement, which is as follows −
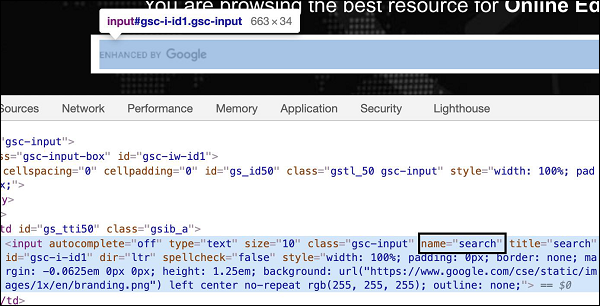
The edit box highlighted in the above image has a name attribute with value search. Let us try to input some text into this edit box after identifying it.
Code Implementation
The code implementation for identifying multiple elements by Name is as follows −
from selenium import webdriver driver = webdriver.Chrome(executable_path='../drivers/chromedriver') #implicit wait time driver.implicitly_wait(5) #url launch driver.get("https://www.tutorialspoint.com/index.htm") #identify elements with name attribute l = driver.find_elements_by_name('search') #count elements s = len(l) #iterate through list for i in l: #obtain text t = i.send_keys('Selenium Python') v = i.get_attribute('value') print('Value entered is: ' + v) #driver quit driver.quit()
Output

The output shows the message - Process with exit code 0 meaning that the above Python code executed successfully. Also, the value entered within the edit box (obtained from the get_attribute method) - Selenium Python gets printed in the console.
By CSS Selector
Once we navigate to a webpage, we have to interact with the webelements available on the page like clicking a link/button, entering text within an edit box, and so on to complete our automation test case.
For this, our first job is to identify the elements. We can create a css selector for their identification and utilize the method find_elements_by_css_selector. With this, the elements with the matching value of the given css are returned in the form of list.
In case there is no element with the matching value of the css, an empty list shall be returned.
The syntax for identifying multiple elements by CSS Selector is as follows −
driver.find_elements_by_css_selector("value of css")
Rules for CSS Expression
The rules to create a css expression are discussed below −
To identify the element with css, the expression should be tagname[attribute='value']. We can also specifically use the id attribute to create a css expression.
With id, the format of a css expression should be tagname#id. For example, input#txt [here input is the tagname and the txt is the value of the id attribute].
With class, the format of css expression should be tagname.class . For example, input.cls-txt [here input is the tagname and the cls-txt is the value of the class attribute].
If there are n children of a parent element, and we want to identify the nth child, the css expression should have nth-of –type(n).
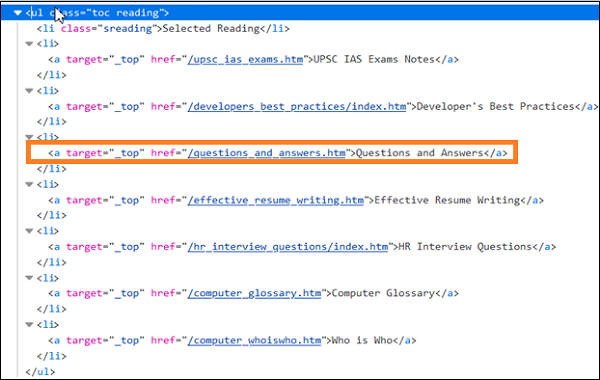
In the above code, if we want to identify the fourth li child of ul[Questions and Answers], the css expression should be ul.reading li:nth-of-type(4). Similarly, to identify the last child, the css expression should be ul.reading li:last-child.
For attributes whose values are dynamically changing, we can use ^= to locate an element whose attribute value starts with a particular text. For example, input[name^='qa'] [here input is the tagname and the value of the name attribute starts with qa].
For attributes whose values are dynamically changing, we can use $= to locate an element whose attribute value ends with a particular text. For example, input[class$='txt'] Here, input is the tagname and the value of the class attribute ends with txt.
For attributes whose values are dynamically changing, we can use *= to locate an element whose attribute value contains a specific sub-text. For example, input[name*='nam'] Here, input is the tagname and the value of the name attribute contains the sub-text nam.
Let us see the html code of a webelement −
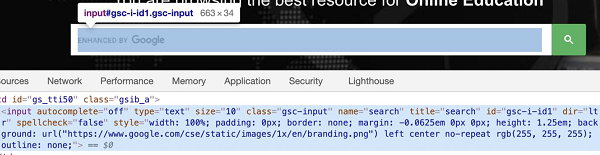
The edit box highlighted in the above image has a name attribute with value search, the css expression should be input[name='search']. Let us try to input some text into this edit box after identifying it.
Code Implementation
The code implementation for identifying multiple elements by CSS Selector is as follows −
from selenium import webdriver driver = webdriver.Chrome(executable_path='../drivers/chromedriver') #implicit wait time driver.implicitly_wait(5) #url launch driver.get("https://www.tutorialspoint.com/index.htm") #identify elements with css l = driver.find_elements_by_css_selector("input[name='search']") #count elements s = len(l) #iterate through list for i in l: #obtain text t = i.send_keys('Tutorialspoint') v = i.get_attribute('value') print('Value entered is: ' + v) #driver quit driver.quit()
Output

The output shows the message - Process with exit code 0 meaning that the above Python code executed successfully. Also, the value entered within the edit box (obtained from the get_attribute method) - Tutorialspoint gets printed in the console.
By Xpath
Once we navigate to a webpage, we have to interact with the webelements available on the page like clicking a link/button, entering text within an edit box, and so on to complete our automation test case.
For this, our first job is to identify the elements. We can create an xpath for their identification and utilize the method find_elements_by_xpath. With this, the elements with the matching value of the given xpath are returned in the form of a list.
In case there is no element with the matching value of the xpath, an empty list shall be returned.
The syntax for identifying multiple elements by Xpath is as follows:
driver.find_elements_by_xpath("value of xpath")
Rules for Xpath Expression
The rules to create a xpath expression are discussed below −
To identify the element with xpath, the expression should be //tagname[@attribute='value']. There can be two types of xpath – relative and absolute. The absolute xpath begins with / symbol and starts from the root node upto the element that we want to identify.
For example,
/html/body/div[1]/div/div[1]/a
The relative xpath begins with // symbol and does not start from the root node.
For example,
//img[@alt='tutorialspoint']
Let us see the html code of the highlighted link - Home starting from the root.
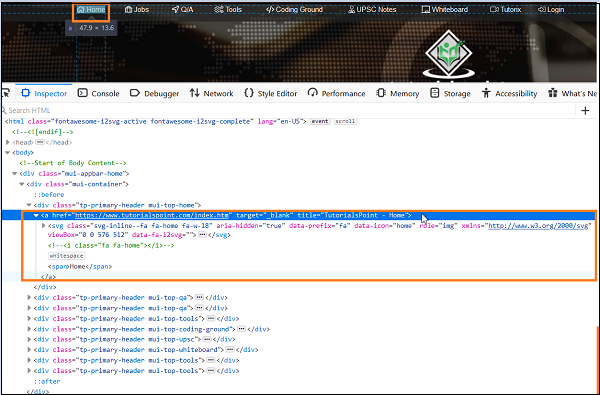
The absolute xpath for the element Home can be as follows −
/html/body/div[1]/div/div[1]/a.
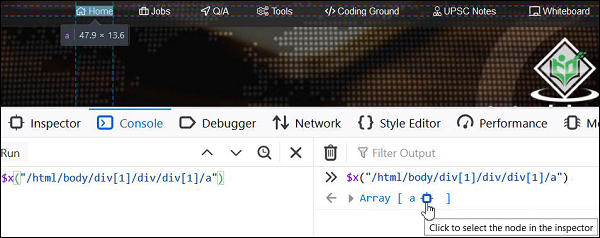
The relative xpath for element Home can be as follows −
//a[@title='TutorialsPoint - Home'].
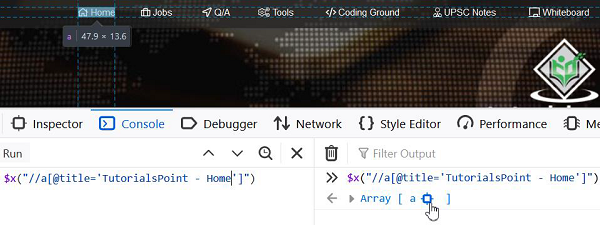
Functions
There are also functions available which help to frame relative xpath expressions −
text()
It is used to identify an element with the help of the visible text on the page. The xpath expression is as follows −
//*[text()='Home'].
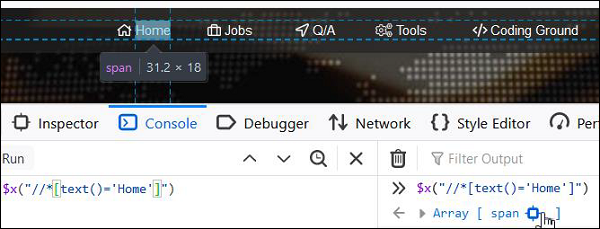
starts-with
It is used to identify an element whose attribute value begins with a specific text. This function is normally used for attributes whose value changes on each page load.
Let us see the html of the element Q/A −
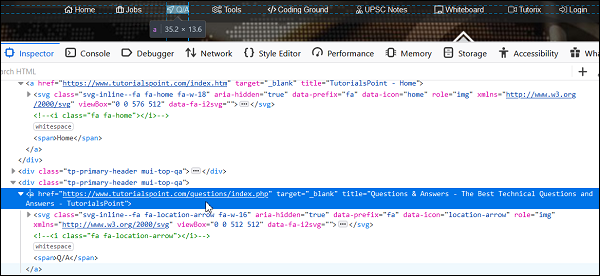
The xpath expression should be as follows −
//a[starts-with(@title, 'Questions &')].
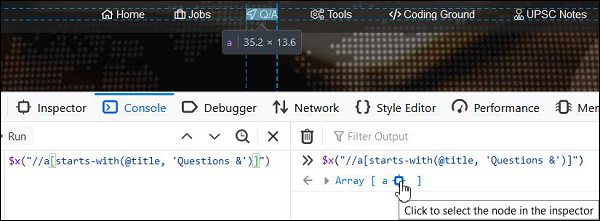
contains()
It identifies an element whose attribute value contains a sub-text. This function is normally used for attributes whose value changes on each page load.
The xpath expression is as follows −
//a[contains(@title, 'Questions & Answers')].
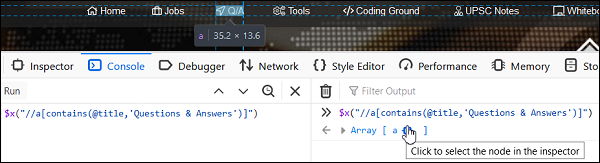
Let us see the html code of a webelement −
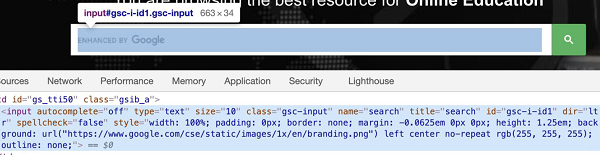
The edit box highlighted in the above image has a name attribute with value search, the xpath expression should be //input[@name='search']. Let us try to input some text into this edit box after identifying it.
Code Implementation
The code implementation for identifying multiple elements by Xpath is as follows −
from selenium import webdriver driver = webdriver.Chrome(executable_path='../drivers/chromedriver') #implicit wait time driver.implicitly_wait(5) #url launch driver.get("https://www.tutorialspoint.com/index.htm") #identify elements with xpath l = driver.find_elements_by_xpath("//input[@name='search']") #count elements s = len(l) #iterate through list for i in l: #obtain text t = i.send_keys('Tutorialspoint - Selenium') v = i.get_attribute('value') print('Value entered is: ' + v) #driver quit driver.quit()
Output

The output shows the message - Process with exit code 0 meaning that the above Python code executed successfully. Also, the value entered within the edit box (obtained from the get_attribute method) - Tutorialspoint - Selenium gets printed in the console.