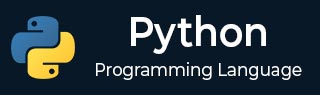
- Python Basics
- Python - Home
- Python - Overview
- Python - History
- Python - Features
- Python vs C++
- Python - Hello World Program
- Python - Application Areas
- Python - Interpreter
- Python - Environment Setup
- Python - Virtual Environment
- Python - Basic Syntax
- Python - Variables
- Python - Data Types
- Python - Type Casting
- Python - Unicode System
- Python - Literals
- Python - Operators
- Python - Arithmetic Operators
- Python - Comparison Operators
- Python - Assignment Operators
- Python - Logical Operators
- Python - Bitwise Operators
- Python - Membership Operators
- Python - Identity Operators
- Python - Operator Precedence
- Python - Comments
- Python - User Input
- Python - Numbers
- Python - Booleans
- Python Control Statements
- Python - Control Flow
- Python - Decision Making
- Python - If Statement
- Python - If else
- Python - Nested If
- Python - Match-Case Statement
- Python - Loops
- Python - for Loops
- Python - for-else Loops
- Python - While Loops
- Python - break Statement
- Python - continue Statement
- Python - pass Statement
- Python - Nested Loops
- Python Functions & Modules
- Python - Functions
- Python - Default Arguments
- Python - Keyword Arguments
- Python - Keyword-Only Arguments
- Python - Positional Arguments
- Python - Positional-Only Arguments
- Python - Arbitrary Arguments
- Python - Variables Scope
- Python - Function Annotations
- Python - Modules
- Python - Built in Functions
- Python Strings
- Python - Strings
- Python - Slicing Strings
- Python - Modify Strings
- Python - String Concatenation
- Python - String Formatting
- Python - Escape Characters
- Python - String Methods
- Python - String Exercises
- Python Lists
- Python - Lists
- Python - Access List Items
- Python - Change List Items
- Python - Add List Items
- Python - Remove List Items
- Python - Loop Lists
- Python - List Comprehension
- Python - Sort Lists
- Python - Copy Lists
- Python - Join Lists
- Python - List Methods
- Python - List Exercises
- Python Tuples
- Python - Tuples
- Python - Access Tuple Items
- Python - Update Tuples
- Python - Unpack Tuples
- Python - Loop Tuples
- Python - Join Tuples
- Python - Tuple Methods
- Python - Tuple Exercises
- Python Sets
- Python - Sets
- Python - Access Set Items
- Python - Add Set Items
- Python - Remove Set Items
- Python - Loop Sets
- Python - Join Sets
- Python - Copy Sets
- Python - Set Operators
- Python - Set Methods
- Python - Set Exercises
- Python Dictionaries
- Python - Dictionaries
- Python - Access Dictionary Items
- Python - Change Dictionary Items
- Python - Add Dictionary Items
- Python - Remove Dictionary Items
- Python - Dictionary View Objects
- Python - Loop Dictionaries
- Python - Copy Dictionaries
- Python - Nested Dictionaries
- Python - Dictionary Methods
- Python - Dictionary Exercises
- Python Arrays
- Python - Arrays
- Python - Access Array Items
- Python - Add Array Items
- Python - Remove Array Items
- Python - Loop Arrays
- Python - Copy Arrays
- Python - Reverse Arrays
- Python - Sort Arrays
- Python - Join Arrays
- Python - Array Methods
- Python - Array Exercises
- Python File Handling
- Python - File Handling
- Python - Write to File
- Python - Read Files
- Python - Renaming and Deleting Files
- Python - Directories
- Python - File Methods
- Python - OS File/Directory Methods
- Object Oriented Programming
- Python - OOPs Concepts
- Python - Object & Classes
- Python - Class Attributes
- Python - Class Methods
- Python - Static Methods
- Python - Constructors
- Python - Access Modifiers
- Python - Inheritance
- Python - Polymorphism
- Python - Method Overriding
- Python - Method Overloading
- Python - Dynamic Binding
- Python - Dynamic Typing
- Python - Abstraction
- Python - Encapsulation
- Python - Interfaces
- Python - Packages
- Python - Inner Classes
- Python - Anonymous Class and Objects
- Python - Singleton Class
- Python - Wrapper Classes
- Python - Enums
- Python - Reflection
- Python Errors & Exceptions
- Python - Syntax Errors
- Python - Exceptions
- Python - try-except Block
- Python - try-finally Block
- Python - Raising Exceptions
- Python - Exception Chaining
- Python - Nested try Block
- Python - User-defined Exception
- Python - Logging
- Python - Assertions
- Python - Built-in Exceptions
- Python Multithreading
- Python - Multithreading
- Python - Thread Life Cycle
- Python - Creating a Thread
- Python - Starting a Thread
- Python - Joining Threads
- Python - Naming Thread
- Python - Thread Scheduling
- Python - Thread Pools
- Python - Main Thread
- Python - Thread Priority
- Python - Daemon Threads
- Python - Synchronizing Threads
- Python Synchronization
- Python - Inter-thread Communication
- Python - Thread Deadlock
- Python - Interrupting a Thread
- Python Networking
- Python - Networking
- Python - Socket Programming
- Python - URL Processing
- Python - Generics
- Python Libraries
- NumPy Tutorial
- Pandas Tutorial
- SciPy Tutorial
- Matplotlib Tutorial
- Django Tutorial
- OpenCV Tutorial
- Python Miscellenous
- Python - Date & Time
- Python - Maths
- Python - Iterators
- Python - Generators
- Python - Closures
- Python - Decorators
- Python - Recursion
- Python - Reg Expressions
- Python - PIP
- Python - Database Access
- Python - Weak References
- Python - Serialization
- Python - Templating
- Python - Output Formatting
- Python - Performance Measurement
- Python - Data Compression
- Python - CGI Programming
- Python - XML Processing
- Python - GUI Programming
- Python - Command-Line Arguments
- Python - Docstrings
- Python - JSON
- Python - Sending Email
- Python - Further Extensions
- Python - Tools/Utilities
- Python - GUIs
- Python Useful Resources
- Python Compiler
- NumPy Compiler
- Matplotlib Compiler
- SciPy Compiler
- Python - Programming Examples
- Python - Quick Guide
- Python - Useful Resources
- Python - Discussion
Python Mock Test
This section presents you various set of Mock Tests related to Python. You can download these sample mock tests at your local machine and solve offline at your convenience. Every mock test is supplied with a mock test key to let you verify the final score and grade yourself.
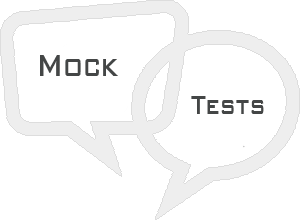
Python Mock Test III
Q 1 - Which of the following operator in python evaluates to true if it does not finds a variable in the specified sequence and false otherwise?
Answer : D
Explanation
not in − Evaluates to true if it does not finds a variable in the specified sequence and false otherwise. x not in y, here not in results in a 1 if x is not a member of sequence y.
Q 2 - Which of the following statement terminates the loop statement and transfers execution to the statement immediately following the loop?
Answer : A
Explanation
break statement − Terminates the loop statement and transfers execution to the statement immediately following the loop.
Q 3 - Which of the following statement causes the loop to skip the remainder of its body and immediately retest its condition prior to reiterating?
Answer : B
Explanation
continue statement − Causes the loop to skip the remainder of its body and immediately retest its condition prior to reiterating.
Q 4 - Which of the following statement is used when a statement is required syntactically but you do not want any command or code to execute?
Answer : C
Explanation
pass statement − The pass statement in Python is used when a statement is required syntactically but you do not want any command or code to execute.
Q 5 - Which of the following function returns a random item from a list, tuple, or string?
Answer : A
Explanation
choice(seq) − Returns a random item from a list, tuple, or string.
Q 6 - Which of the following function returns a randomly selected element from range?
Answer : B
Explanation
randrange ([start,] stop [,step]) − returns a randomly selected element from range(start, stop, step).
Q 7 - Which of the following function returns a random float r, such that 0 is less than or equal to r and r is less than 1?
Answer : C
Explanation
random() − returns a random float r, such that 0 is less than or equal to r and r is less than 1.
Q 8 - Which of the following function sets the integer starting value used in generating random numbers?
Answer : D
Explanation
seed([x]) − Sets the integer starting value used in generating random numbers. Call this function before calling any other random module function. Returns None.
Q 9 - Which of the following function randomizes the items of a list in place?
Answer : A
Explanation
shuffle(lst) − Randomizes the items of a list in place. Returns None.
Q 10 - Which of the following function capitalizes first letter of string?
Answer : B
Explanation
capitalize() − Capitalizes first letter of string.
Q 11 - Which of the following function checks in a string that all characters are alphanumeric?
Answer : C
Explanation
isalnum() − Returns true if string has at least 1 character and all characters are alphanumeric and false otherwise.
Q 12 - Which of the following function checks in a string that all characters are digits?
Answer : D
Explanation
isdigit() − Returns true if string has at least 1 character and all characters are digits and false otherwise.
Q 13 - Which of the following function checks in a string that all characters are in lowercase?
Answer : A
Explanation
islower() − Returns true if string has at least 1 character and all characters are in lowercase.
Q 14 - Which of the following function checks in a string that all characters are numeric?
Answer : B
Explanation
isnumeric() − Returns true if string has at least 1 character and all characters are numeric.
Q 15 - Which of the following function checks in a string that all characters are whitespaces?
Answer : C
Explanation
isspace() − Returns true if string has at least 1 character and all characters are whitespaces.
Q 16 - Which of the following function checks in a string that all characters are titlecased?
Answer : D
Explanation
istitle() − Returns true if string has at least 1 character and all characters are titlecased.
Q 17 - Which of the following function checks in a string that all characters are in uppercase?
Answer : A
Explanation
isupper() − Returns true if string has at least 1 character and all characters are in uppercase.
Q 18 - Which of the following function merges elements in a sequence?
Answer : B
Explanation
join(seq) − Merges (concatenates) the string representations of elements in sequence seq into a string, with separator string.
Q 19 - Which of the following function gets the length of the string?
Answer : C
Explanation
len(string) − Returns the length of the string.
Q 20 - Which of the following function gets a space-padded string with the original string left-justified to a total of width columns?
Answer : D
Explanation
ljust(width[, fillchar]) − Returns a space-padded string with the original string left-justified to a total of width columns.
Q 21 - Which of the following function converts a string to all lowercase?
Answer : A
Explanation
lower() − Converts all uppercase letters in string to lowercase.
Q 22 - Which of the following function removes all leading whitespace in string?
Answer : B
Explanation
lstrip() − Removes all leading whitespace in string.
Q 23 - Which of the following function returns the max alphabetical character from the string str?
Answer : C
Explanation
max(str) − Returns the max alphabetical character from the string str.
Q 24 - Which of the following function returns the min alphabetical character from the string str?
Answer : D
Explanation
min(str) − Returns the min alphabetical character from the string str.
Q 25 - Which of the following function replaces all occurrences of old substring in string with new string?
Answer : A
Explanation
replace(old, new [, max]) − Replaces all occurrences of old in string with new or at most max occurrences if max given.
Answer Sheet
Question Number | Answer Key |
---|---|
1 | D |
2 | A |
3 | B |
4 | C |
5 | A |
6 | B |
7 | C |
8 | D |
9 | A |
10 | B |
11 | C |
12 | D |
13 | A |
14 | B |
15 | C |
16 | D |
17 | A |
18 | B |
19 | C |
20 | D |
21 | A |
22 | B |
23 | C |
24 | D |
25 | A |