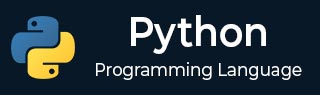
- Python Basics
- Python - Home
- Python - Overview
- Python - History
- Python - Features
- Python vs C++
- Python - Hello World Program
- Python - Application Areas
- Python - Interpreter
- Python - Environment Setup
- Python - Virtual Environment
- Python - Basic Syntax
- Python - Variables
- Python - Data Types
- Python - Type Casting
- Python - Unicode System
- Python - Literals
- Python - Operators
- Python - Arithmetic Operators
- Python - Comparison Operators
- Python - Assignment Operators
- Python - Logical Operators
- Python - Bitwise Operators
- Python - Membership Operators
- Python - Identity Operators
- Python - Operator Precedence
- Python - Comments
- Python - User Input
- Python - Numbers
- Python - Booleans
- Python Control Statements
- Python - Control Flow
- Python - Decision Making
- Python - If Statement
- Python - If else
- Python - Nested If
- Python - Match-Case Statement
- Python - Loops
- Python - for Loops
- Python - for-else Loops
- Python - While Loops
- Python - break Statement
- Python - continue Statement
- Python - pass Statement
- Python - Nested Loops
- Python Functions & Modules
- Python - Functions
- Python - Default Arguments
- Python - Keyword Arguments
- Python - Keyword-Only Arguments
- Python - Positional Arguments
- Python - Positional-Only Arguments
- Python - Arbitrary Arguments
- Python - Variables Scope
- Python - Function Annotations
- Python - Modules
- Python - Built in Functions
- Python Strings
- Python - Strings
- Python - Slicing Strings
- Python - Modify Strings
- Python - String Concatenation
- Python - String Formatting
- Python - Escape Characters
- Python - String Methods
- Python - String Exercises
- Python Lists
- Python - Lists
- Python - Access List Items
- Python - Change List Items
- Python - Add List Items
- Python - Remove List Items
- Python - Loop Lists
- Python - List Comprehension
- Python - Sort Lists
- Python - Copy Lists
- Python - Join Lists
- Python - List Methods
- Python - List Exercises
- Python Tuples
- Python - Tuples
- Python - Access Tuple Items
- Python - Update Tuples
- Python - Unpack Tuples
- Python - Loop Tuples
- Python - Join Tuples
- Python - Tuple Methods
- Python - Tuple Exercises
- Python Sets
- Python - Sets
- Python - Access Set Items
- Python - Add Set Items
- Python - Remove Set Items
- Python - Loop Sets
- Python - Join Sets
- Python - Copy Sets
- Python - Set Operators
- Python - Set Methods
- Python - Set Exercises
- Python Dictionaries
- Python - Dictionaries
- Python - Access Dictionary Items
- Python - Change Dictionary Items
- Python - Add Dictionary Items
- Python - Remove Dictionary Items
- Python - Dictionary View Objects
- Python - Loop Dictionaries
- Python - Copy Dictionaries
- Python - Nested Dictionaries
- Python - Dictionary Methods
- Python - Dictionary Exercises
- Python Arrays
- Python - Arrays
- Python - Access Array Items
- Python - Add Array Items
- Python - Remove Array Items
- Python - Loop Arrays
- Python - Copy Arrays
- Python - Reverse Arrays
- Python - Sort Arrays
- Python - Join Arrays
- Python - Array Methods
- Python - Array Exercises
- Python File Handling
- Python - File Handling
- Python - Write to File
- Python - Read Files
- Python - Renaming and Deleting Files
- Python - Directories
- Python - File Methods
- Python - OS File/Directory Methods
- Object Oriented Programming
- Python - OOPs Concepts
- Python - Object & Classes
- Python - Class Attributes
- Python - Class Methods
- Python - Static Methods
- Python - Constructors
- Python - Access Modifiers
- Python - Inheritance
- Python - Polymorphism
- Python - Method Overriding
- Python - Method Overloading
- Python - Dynamic Binding
- Python - Dynamic Typing
- Python - Abstraction
- Python - Encapsulation
- Python - Interfaces
- Python - Packages
- Python - Inner Classes
- Python - Anonymous Class and Objects
- Python - Singleton Class
- Python - Wrapper Classes
- Python - Enums
- Python - Reflection
- Python Errors & Exceptions
- Python - Syntax Errors
- Python - Exceptions
- Python - try-except Block
- Python - try-finally Block
- Python - Raising Exceptions
- Python - Exception Chaining
- Python - Nested try Block
- Python - User-defined Exception
- Python - Logging
- Python - Assertions
- Python - Built-in Exceptions
- Python Multithreading
- Python - Multithreading
- Python - Thread Life Cycle
- Python - Creating a Thread
- Python - Starting a Thread
- Python - Joining Threads
- Python - Naming Thread
- Python - Thread Scheduling
- Python - Thread Pools
- Python - Main Thread
- Python - Thread Priority
- Python - Daemon Threads
- Python - Synchronizing Threads
- Python Synchronization
- Python - Inter-thread Communication
- Python - Thread Deadlock
- Python - Interrupting a Thread
- Python Networking
- Python - Networking
- Python - Socket Programming
- Python - URL Processing
- Python - Generics
- Python Libraries
- NumPy Tutorial
- Pandas Tutorial
- SciPy Tutorial
- Matplotlib Tutorial
- Django Tutorial
- OpenCV Tutorial
- Python Miscellenous
- Python - Date & Time
- Python - Maths
- Python - Iterators
- Python - Generators
- Python - Closures
- Python - Decorators
- Python - Recursion
- Python - Reg Expressions
- Python - PIP
- Python - Database Access
- Python - Weak References
- Python - Serialization
- Python - Templating
- Python - Output Formatting
- Python - Performance Measurement
- Python - Data Compression
- Python - CGI Programming
- Python - XML Processing
- Python - GUI Programming
- Python - Command-Line Arguments
- Python - Docstrings
- Python - JSON
- Python - Sending Email
- Python - Further Extensions
- Python - Tools/Utilities
- Python - GUIs
- Python Useful Resources
- Python Compiler
- NumPy Compiler
- Matplotlib Compiler
- SciPy Compiler
- Python - Programming Examples
- Python - Quick Guide
- Python - Useful Resources
- Python - Discussion
Python - Access Modifiers
The languages such as C++ and Java, use access modifiers to restrict access to class members (i.e., variables and methods). These languages have keywords public, protected, and private to specify the type of access.
A class member is said to be public if it can be accessed from anywhere in the program. Private members are allowed to be accessed from within the class only.
Usually, methods are defined as public and instance variable are private. This arrangement of private instance variables and public methods ensures implementation of principle of encapsulation.
Protected members are accessible from within the class as well as by classes derived from that class.
Unlike these languages, Python has no provision to specify the type of access that a class member may have. By default, all the variables and methods in a class are public.
Example
Here, we have Employee class with instance variables name and age. An object of this class has these two attributes. They can be directly accessed from outside the class, because they are public.
class Employee: 'Common base class for all employees' def __init__(self, name="Bhavana", age=24): self.name = name self.age = age e1 = Employee() e2 = Employee("Bharat", 25) print ("Name: {}".format(e1.name)) print ("age: {}".format(e1.age)) print ("Name: {}".format(e2.name)) print ("age: {}".format(e2.age))
It will produce the following output −
Name: Bhavana age: 24 Name: Bharat age: 25
Python doesn't enforce restrictions on accessing any instance variable or method. However, Python prescribes a convention of prefixing name of variable/method with single or double underscore to emulate behavior of protected and private access modifiers.
To indicate that an instance variable is private, prefix it with double underscore (such as "__age"). To imply that a certain instance variable is protected, prefix it with single underscore (such as "_salary")
Example
Let us modify the Employee class. Add another instance variable salary. Make age private and salary as protected by prefixing double and single underscores respectively.
class Employee: def __init__(self, name, age, salary): self.name = name # public variable self.__age = age # private variable self._salary = salary # protected variable def displayEmployee(self): print ("Name : ", self.name, ", age: ", self.__age, ", salary: ", self._salary) e1=Employee("Bhavana", 24, 10000) print (e1.name) print (e1._salary) print (e1.__age)
When you run this code, it will produce the following output −
Bhavana 10000 Traceback (most recent call last): File "C:\Users\user\example.py", line 14, in <module> print (e1.__age) ^^^^^^^^ AttributeError: 'Employee' object has no attribute '__age'
Python displays AttributeError because __age is private, and not available for use outside the class.
Name Mangling
Python doesn't block access to private data, it just leaves for the wisdom of the programmer, not to write any code that access it from outside the class. You can still access the private members by Python's name mangling technique.
Name mangling is the process of changing name of a member with double underscore to the form object._class__variable. If so required, it can still be accessed from outside the class, but the practice should be refrained.
In our example, the private instance variable "__name" is mangled by changing it to the format
obj._class__privatevar
So, to access the value of "__age" instance variable of "e1" object, change it to "e1._Employee__age".
Change the print() statement in the above program to −
print (e1._Employee__age)
It now prints 24, the age of e1.
Python Property Object
Python's standard library has a built-in property() function. It returns a property object. It acts as an interface to the instance variables of a Python class.
The encapsulation principle of object-oriented programming requires that the instance variables should have a restricted private access. Python doesn't have efficient mechanism for the purpose. The property() function provides an alternative.
The property() function uses the getter, setter and delete methods defined in a class to define a property object for the class.
Syntax
property(fget=None, fset=None, fdel=None, doc=None)
Parameters
fget − an instance method that retrieves value of an instance variable.
fset − an instance method that assigns value to an instance variable.
fdel − an instance method that removes an instance variable
fdoc − Documentation string for the property.
The function uses getter and setter methods to return the property object.
Getters and Setter Methods
A getter method retrieves the value of an instance variable, usually named as get_varname, whereas the setter method assigns value to an instance variable − named as set_varname.
Let us define getter methods get_name() and get_age(), and setters set_name() and set_age() in the Employee class.
Example
class Employee: def __init__(self, name, age): self.__name = name self.__age = age def get_name(self): return self.__name def get_age(self): return self.__age def set_name(self, name): self.__name = name return def set_age(self, age): self.__age=age e1=Employee("Bhavana", 24) print ("Name:", e1.get_name(), "age:", e1.get_age()) e1.set_name("Archana") e1.set_age(21) print ("Name:", e1.get_name(), "age:", e1.get_age())
It will produce the following output −
Name: Bhavana age: 24 Name: Archana age: 21
The getter and setter methods can retrieve or assign value to instance variables. The property() function uses them to add property objects as class attributes.
The name property is defined as −
name = property(get_name, set_name, "name")
Similarly, you can add the age property −
age = property(get_age, set_age, "age")
The advantage of the property object is that you can use to retrieve the value of its associated instance variable, as well as assign value.
For example,
print (e1.name) displays value of e1.__name e1.name = "Archana" assigns value to e1.__age
Example
The complete program with property objects and their use is given below −
class Employee: def __init__(self, name, age): self.__name = name self.__age = age def get_name(self): return self.__name def get_age(self): return self.__age def set_name(self, name): self.__name = name return def set_age(self, age): self.__age=age return name = property(get_name, set_name, "name") age = property(get_age, set_age, "age") e1=Employee("Bhavana", 24) print ("Name:", e1.name, "age:", e1.age) e1.name = "Archana" e1.age = 23 print ("Name:", e1.name, "age:", e1.age)
It will produce the following output −
Name: Bhavana age: 24 Name: Archana age: 23