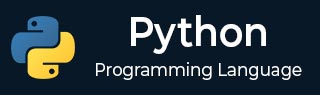
- Python Basics
- Python - Home
- Python - Overview
- Python - History
- Python - Features
- Python vs C++
- Python - Hello World Program
- Python - Application Areas
- Python - Interpreter
- Python - Environment Setup
- Python - Virtual Environment
- Python - Basic Syntax
- Python - Variables
- Python - Data Types
- Python - Type Casting
- Python - Unicode System
- Python - Literals
- Python - Operators
- Python - Arithmetic Operators
- Python - Comparison Operators
- Python - Assignment Operators
- Python - Logical Operators
- Python - Bitwise Operators
- Python - Membership Operators
- Python - Identity Operators
- Python - Operator Precedence
- Python - Comments
- Python - User Input
- Python - Numbers
- Python - Booleans
- Python Control Statements
- Python - Control Flow
- Python - Decision Making
- Python - If Statement
- Python - If else
- Python - Nested If
- Python - Match-Case Statement
- Python - Loops
- Python - for Loops
- Python - for-else Loops
- Python - While Loops
- Python - break Statement
- Python - continue Statement
- Python - pass Statement
- Python - Nested Loops
- Python Functions & Modules
- Python - Functions
- Python - Default Arguments
- Python - Keyword Arguments
- Python - Keyword-Only Arguments
- Python - Positional Arguments
- Python - Positional-Only Arguments
- Python - Arbitrary Arguments
- Python - Variables Scope
- Python - Function Annotations
- Python - Modules
- Python - Built in Functions
- Python Strings
- Python - Strings
- Python - Slicing Strings
- Python - Modify Strings
- Python - String Concatenation
- Python - String Formatting
- Python - Escape Characters
- Python - String Methods
- Python - String Exercises
- Python Lists
- Python - Lists
- Python - Access List Items
- Python - Change List Items
- Python - Add List Items
- Python - Remove List Items
- Python - Loop Lists
- Python - List Comprehension
- Python - Sort Lists
- Python - Copy Lists
- Python - Join Lists
- Python - List Methods
- Python - List Exercises
- Python Tuples
- Python - Tuples
- Python - Access Tuple Items
- Python - Update Tuples
- Python - Unpack Tuples
- Python - Loop Tuples
- Python - Join Tuples
- Python - Tuple Methods
- Python - Tuple Exercises
- Python Sets
- Python - Sets
- Python - Access Set Items
- Python - Add Set Items
- Python - Remove Set Items
- Python - Loop Sets
- Python - Join Sets
- Python - Copy Sets
- Python - Set Operators
- Python - Set Methods
- Python - Set Exercises
- Python Dictionaries
- Python - Dictionaries
- Python - Access Dictionary Items
- Python - Change Dictionary Items
- Python - Add Dictionary Items
- Python - Remove Dictionary Items
- Python - Dictionary View Objects
- Python - Loop Dictionaries
- Python - Copy Dictionaries
- Python - Nested Dictionaries
- Python - Dictionary Methods
- Python - Dictionary Exercises
- Python Arrays
- Python - Arrays
- Python - Access Array Items
- Python - Add Array Items
- Python - Remove Array Items
- Python - Loop Arrays
- Python - Copy Arrays
- Python - Reverse Arrays
- Python - Sort Arrays
- Python - Join Arrays
- Python - Array Methods
- Python - Array Exercises
- Python File Handling
- Python - File Handling
- Python - Write to File
- Python - Read Files
- Python - Renaming and Deleting Files
- Python - Directories
- Python - File Methods
- Python - OS File/Directory Methods
- Object Oriented Programming
- Python - OOPs Concepts
- Python - Object & Classes
- Python - Class Attributes
- Python - Class Methods
- Python - Static Methods
- Python - Constructors
- Python - Access Modifiers
- Python - Inheritance
- Python - Polymorphism
- Python - Method Overriding
- Python - Method Overloading
- Python - Dynamic Binding
- Python - Dynamic Typing
- Python - Abstraction
- Python - Encapsulation
- Python - Interfaces
- Python - Packages
- Python - Inner Classes
- Python - Anonymous Class and Objects
- Python - Singleton Class
- Python - Wrapper Classes
- Python - Enums
- Python - Reflection
- Python Errors & Exceptions
- Python - Syntax Errors
- Python - Exceptions
- Python - try-except Block
- Python - try-finally Block
- Python - Raising Exceptions
- Python - Exception Chaining
- Python - Nested try Block
- Python - User-defined Exception
- Python - Logging
- Python - Assertions
- Python - Built-in Exceptions
- Python Multithreading
- Python - Multithreading
- Python - Thread Life Cycle
- Python - Creating a Thread
- Python - Starting a Thread
- Python - Joining Threads
- Python - Naming Thread
- Python - Thread Scheduling
- Python - Thread Pools
- Python - Main Thread
- Python - Thread Priority
- Python - Daemon Threads
- Python - Synchronizing Threads
- Python Synchronization
- Python - Inter-thread Communication
- Python - Thread Deadlock
- Python - Interrupting a Thread
- Python Networking
- Python - Networking
- Python - Socket Programming
- Python - URL Processing
- Python - Generics
- Python Libraries
- NumPy Tutorial
- Pandas Tutorial
- SciPy Tutorial
- Matplotlib Tutorial
- Django Tutorial
- OpenCV Tutorial
- Python Miscellenous
- Python - Date & Time
- Python - Maths
- Python - Iterators
- Python - Generators
- Python - Closures
- Python - Decorators
- Python - Recursion
- Python - Reg Expressions
- Python - PIP
- Python - Database Access
- Python - Weak References
- Python - Serialization
- Python - Templating
- Python - Output Formatting
- Python - Performance Measurement
- Python - Data Compression
- Python - CGI Programming
- Python - XML Processing
- Python - GUI Programming
- Python - Command-Line Arguments
- Python - Docstrings
- Python - JSON
- Python - Sending Email
- Python - Further Extensions
- Python - Tools/Utilities
- Python - GUIs
- Python Useful Resources
- Python Compiler
- NumPy Compiler
- Matplotlib Compiler
- SciPy Compiler
- Python - Programming Examples
- Python - Quick Guide
- Python - Useful Resources
- Python - Discussion
Python - math Module
Python's standard library provides math module. This module includes many pre-defined functions for performing different mathematical operations. These functions do not work with complex numbers. There is a cmath module contains mathematical functions for complex numbers.
Following are various functions available in the Python math module −
Theoretic and Representation Functions
Python includes following theoretic and representation Functions in the math module −
Sr.No. | Function & Description |
---|---|
1 |
The ceiling of x: the smallest integer not less than x |
2 |
math.comb(n,k)
This function is used to find the returns the number of ways to choose "x" items from "y" items without repetition and without order. |
3 |
This function returns a float with the magnitude (absolute value) of x but the sign of y. |
4 |
This function is used to compare the values of to objects. This function is deprecated in Python3. |
5 |
This function is used to calculate the absolute value of a given integer. |
6 |
This function is used to find the factorial of a given integer. |
7 |
This function calculates the floor value of a given integer. |
8 |
The fmod() function in math module returns same result as the "%" operator. However fmod() gives more accurate result of modulo division than modulo operator. |
9 |
This function is used to calculate the mantissa and exponent of a given number. |
10 |
This function returns the floating point sum of all numeric items in an iterable i.e. list, tuple, array. |
11 |
This function is used to calculate the greatest common divisor of all the given integers. |
12 |
This function is used to determine whether two given numeric values are close to each other. |
13 |
This function is used to determine whether the given number is a finite number. |
14 |
This function is used to determine whether the given value is infinity (+ve or, -ve). |
15 |
This function is used to determine whether the given number is "NaN". |
16 |
This function calculates the integer square-root of the given non negative integer. |
17 |
This function is used to calculate the least common factor of the given integer arguments. |
18 |
This function returns product of first number with exponent of second number. So, ldexp(x,y) returns x*2**y. This is inverse of frexp() function. |
19 |
This returns the fractional and integer parts of x in a two-item tuple. |
20 |
This function returns the next floating-point value after x towards y. |
21 |
This function is used to calculate the permutation. It returns the number of ways to choose x items from y items without repetition and with order. |
22 |
This function is used to calculate the product of all numeric items in the iterable (list, tuple) given as argument. |
23 |
This function returns the remainder of x with respect to y. This is the difference x − n*y, where n is the integer closest to the quotient x / y. |
24 |
This function returns integral part of the number, removing the fractional part. trunc() is equivalent to floor() for positive x, and equivalent to ceil() for negative x. |
25 |
This function returns the value of the least significant bit of the float x. trunc() is equivalent to floor() for positive x, and equivalent to ceil() for negative x. |
Power and Logarithmic Functions
Sr.No. | Function & Description |
---|---|
1 |
This function is used to calculate the cube root of a number. |
2 |
This function calculate the exponential of x: ex |
3 |
This function returns 2 raised to power x. It is equivalent to 2**x. |
4 |
This function returns e raised to the power x, minus 1. Here e is the base of natural logarithms. |
5 |
This function calculates the natural logarithm of x, for x> 0. |
6 |
This function returns the natural logarithm of 1+x (base e). The result is calculated in a way which is accurate for x near zero. |
7 |
This function returns the base-2 logarithm of x. This is usually more accurate than log(x, 2). |
8 |
The base-10 logarithm of x for x> 0. |
9 |
The value of x**y. |
10 |
The square root of x for x > 0 |
Trigonometric Functions
Python includes following functions that perform trigonometric calculations in the math module −
Sr.No. | Function & Description |
---|---|
1 |
This function returns the arc cosine of x, in radians. |
2 |
This function returns the arc sine of x, in radians. |
3 |
This function returns the arc tangent of x, in radians. |
4 |
This function returns atan(y / x), in radians. |
5 |
This function returns the cosine of x radians. |
6 |
This function returns the sine of x radians. |
7 |
This function returns the tangent of x radians. |
8 |
This function returns the Euclidean norm, sqrt(x*x + y*y). |
Angular conversion Functions
Following are the angular conversion function provided by Python math module −
Sr.No. | Function & Description |
---|---|
1 |
This function converts the given angle from radians to degrees. |
2 |
This function converts the given angle from degrees to radians. |
Mathematical Constants
The Python math module defines the following mathematical constants −
Sr.No. | Constants & Description |
---|---|
1 |
This represents the mathematical constant pi, which equals to "3.141592..." to available precision. |
2 |
This represents the mathematical constant e, which is equal to "2.718281..." to available precision. |
3 |
This represents the mathematical constant Tau (denoted by τ ). It is equivalent to the ratio of circumference to radius, and is equal to 2Π. |
4 |
This represents positive infinity. For negative infinity use "−math.inf". |
5 |
This constant is a floating-point "not a number" (NaN) value. Its value is equivalent to the output of float('nan'). |
Hyperbolic Functions
Hyperbolic functions are analogs of trigonometric functions that are based on hyperbolas instead of circles. Following are the hyperbolic functions of the Python math module −
Sr.No. | Function & Description |
---|---|
1 |
This function is used to calculate the inverse hyperbolic cosine of the given value. |
2 |
This function is used to calculate the inverse hyperbolic sine of a given number. |
3 |
This function is used to calculate the inverse hyperbolic tangent of a number. |
4 |
This function is used to calculate the hyperbolic cosine of the given value. |
5 |
This function is used to calculate the hyperbolic sine of a given number. |
6 |
This function is used to calculate the hyperbolic tangent of a number. |
Special Functions
Following are the special functions provided by the Python math module −
Sr.No. | Function & Description |
---|---|
1 |
This function returns the value of the Gauss error function for the given parameter. |
2 |
This function is the complementary for the error function. Value of erf(x) is equivalent to 1-erf(x). |
3 |
This is used to calculate the factorial of the complex numbers. It is defined for all the complex numbers except the non-positive integers. |
4 |
This function is used to calculate the natural logarithm of the absolute value of the Gamma function at x. |