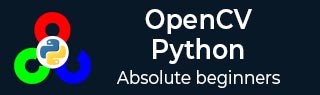
- OpenCV Python Tutorial
- OpenCV Python - Home
- OpenCV Python - Overview
- OpenCV Python - Environment
- OpenCV Python - Reading Image
- OpenCV Python - Write Image
- OpenCV Python - Using Matplotlib
- OpenCV Python - Image Properties
- OpenCV Python - Bitwise Operations
- OpenCV Python - Shapes and Text
- OpenCV Python - Mouse Events
- OpenCV Python - Add Trackbar
- OpenCV Python - Resize and Rotate
- OpenCV Python - Image Threshold
- OpenCV Python - Image Filtering
- OpenCV Python - Edge Detection
- OpenCV Python - Histogram
- OpenCV Python - Color Spaces
- OpenCV Python - Transformations
- OpenCV Python - Image Contours
- OpenCV Python - Template Matching
- OpenCV Python - Image Pyramids
- OpenCV Python - Image Addition
- OpenCV Python - Image Blending
- OpenCV Python - Fourier Transform
- OpenCV Python - Capture Videos
- OpenCV Python - Play Videos
- OpenCV Python - Images From Video
- OpenCV Python - Video from Images
- OpenCV Python - Face Detection
- OpenCV Python - Meanshift/Camshift
- OpenCV Python - Feature Detection
- OpenCV Python - Feature Matching
- OpenCV Python - Digit Recognition
- OpenCV Python Resources
- OpenCV Python - Quick Guide
- OpenCV Python - Resources
- OpenCV Python - Discussion
OpenCV Python - Image Addition
When an image is read by imread() function, the resultant image object is really a two or three dimensional matrix depending upon if the image is grayscale or RGB image.
Hence, cv2.add() functions add two image matrices and returns another image matrix.
Example
Following code reads two images and performs their binary addition −
kalam = cv2.imread('kalam.jpg') einst = cv2.imread('einstein.jpg') img = cv2.add(kalam, einst) cv2.imshow('addition', img)
Result
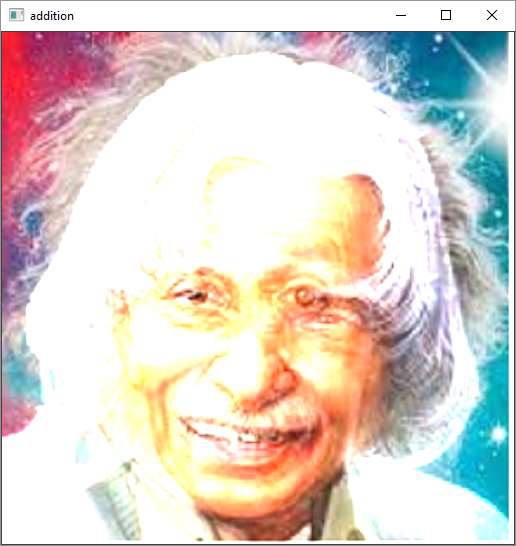
Instead of a linear binary addition, OpenCV has a addWeighted() function that performs weighted sum of two arrays. The command for the same is as follows
Cv2.addWeighted(src1, alpha, src2, beta, gamma)
Parameters
The parameters of the addWeighted() function are as follows −
- src1 − First input array.
- alpha − Weight of the first array elements.
- src2 − Second input array of the same size and channel number as first
- beta − Weight of the second array elements.
- gamma − Scalar added to each sum.
This function adds the images as per following equation −
$$\mathrm{g(x)=(1-\alpha)f_{0}(x)+\alpha f_{1}(x)}$$
The image matrices obtained in the above example are used to perform weighted sum.
By varying a from 0 -> 1, a smooth transition takes place from one image to another, so that they blend together.
First image is given a weight of 0.3 and the second image is given 0.7. The gamma factor is taken as 0.
The command for addWeighted() function is as follows −
img = cv2.addWeighted(kalam, 0.3, einst, 0.7, 0)
It can be seen that the image addition is smoother compared to binary addition.
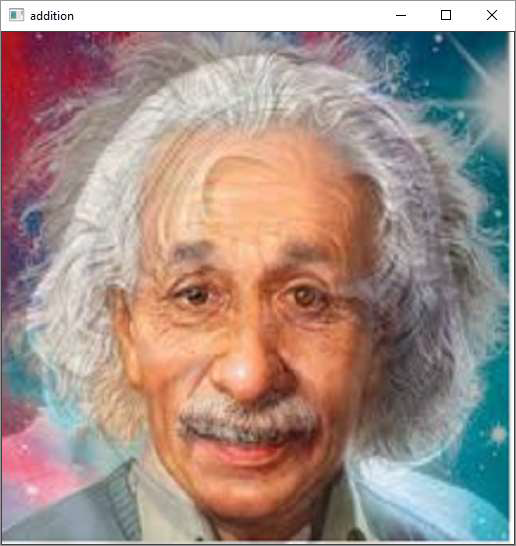