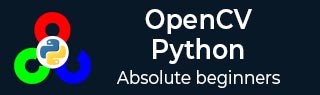
- OpenCV Python Tutorial
- OpenCV Python - Home
- OpenCV Python - Overview
- OpenCV Python - Environment
- OpenCV Python - Reading Image
- OpenCV Python - Write Image
- OpenCV Python - Using Matplotlib
- OpenCV Python - Image Properties
- OpenCV Python - Bitwise Operations
- OpenCV Python - Shapes and Text
- OpenCV Python - Mouse Events
- OpenCV Python - Add Trackbar
- OpenCV Python - Resize and Rotate
- OpenCV Python - Image Threshold
- OpenCV Python - Image Filtering
- OpenCV Python - Edge Detection
- OpenCV Python - Histogram
- OpenCV Python - Color Spaces
- OpenCV Python - Transformations
- OpenCV Python - Image Contours
- OpenCV Python - Template Matching
- OpenCV Python - Image Pyramids
- OpenCV Python - Image Addition
- OpenCV Python - Image Blending
- OpenCV Python - Fourier Transform
- OpenCV Python - Capture Videos
- OpenCV Python - Play Videos
- OpenCV Python - Images From Video
- OpenCV Python - Video from Images
- OpenCV Python - Face Detection
- OpenCV Python - Meanshift/Camshift
- OpenCV Python - Feature Detection
- OpenCV Python - Feature Matching
- OpenCV Python - Digit Recognition
- OpenCV Python Resources
- OpenCV Python - Quick Guide
- OpenCV Python - Resources
- OpenCV Python - Discussion
OpenCV Python - Handling Mouse Events
OpenCV is capable of registering various mouse related events with a callback function. This is done to initiate a certain user defined action depending on the type of mouse event.
Sr.No | Mouse event & Description |
---|---|
1 | cv.EVENT_MOUSEMOVE When the mouse pointer has moved over the window. |
2 | cv.EVENT_LBUTTONDOWN Indicates that the left mouse button is pressed. |
3 | cv.EVENT_RBUTTONDOWN Event of that the right mouse button is pressed. |
4 | cv.EVENT_MBUTTONDOWN Indicates that the middle mouse button is pressed. |
5 | cv.EVENT_LBUTTONUP When the left mouse button is released. |
6 | cv.EVENT_RBUTTONUP When the right mouse button is released. |
7 | cv.EVENT_MBUTTONUP Indicates that the middle mouse button is released. |
8 | cv.EVENT_LBUTTONDBLCLK This event occurs when the left mouse button is double clicked. |
9 | cv.EVENT_RBUTTONDBLCLK Indicates that the right mouse button is double clicked. |
10 | cv.EVENT_MBUTTONDBLCLK Indicates that the middle mouse button is double clicked. |
11 | cv.EVENT_MOUSEWHEEL Positive for forward and negative for backward scrolling. |
To fire a function on a mouse event, it has to be registered with the help of setMouseCallback() function. The command for the same is as follows −
cv2.setMouseCallback(window, callbak_function)
This function passes the type and location of the event to the callback function for further processing.
Example 1
Following code draws a circle whenever left button double click event occurs on the window showing an image as background −
import numpy as np import cv2 as cv # mouse callback function def drawfunction(event,x,y,flags,param): if event == cv.EVENT_LBUTTONDBLCLK: cv.circle(img,(x,y),20,(255,255,255),-1) img = cv.imread('lena.jpg') cv.namedWindow('image') cv.setMouseCallback('image',drawfunction) while(1): cv.imshow('image',img) key=cv.waitKey(1) if key == 27: break cv.destroyAllWindows()
Output
Run the above program and double click at random locations. The similar output will appear −
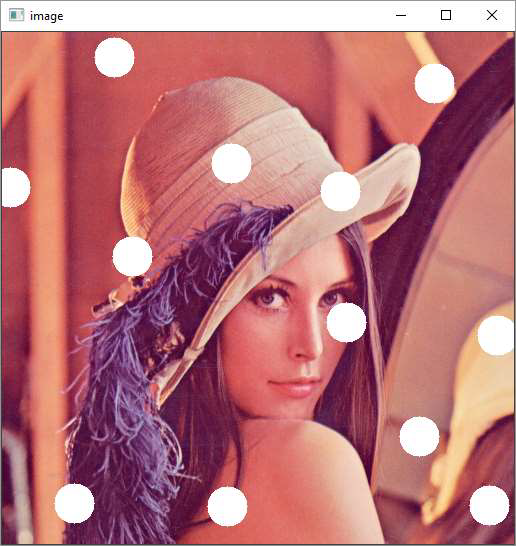
Example 2
Following program interactively draws either rectangle, line or circle depending on user input (1,2 or 3) −
import numpy as np import cv2 as cv # mouse callback function drawing=True shape='r' def draw_circle(event,x,y,flags,param): global x1,x2 if event == cv.EVENT_LBUTTONDOWN: drawing = True x1,x2 = x,y elif event == cv.EVENT_LBUTTONUP: drawing = False if shape == 'r': cv.rectangle(img,(x1,x2),(x,y),(0,255,0),-1) if shape == 'l': cv.line(img,(x1,x2),(x,y),(255,255,255),3) if shape=='c': cv.circle(img,(x,y), 10, (255,255,0), -1) img = cv.imread('lena.jpg') cv.namedWindow('image') cv.setMouseCallback('image',draw_circle) while(1): cv.imshow('image',img) key=cv.waitKey(1) if key==ord('1'): shape='r' if key==ord('2'): shape='l' if key==ord('3'): shape='c' #print (shape) if key == 27: break cv.destroyAllWindows()
On the window surface, a rectangle is drawn between the coordinates of the mouse left button down and up if ‘1’ is pressed.
If user choice is 2, a line is drawn using coordinates as endpoints.
On choosing 3 for the circle, it is drawn at the coordinates of the mouse up event.
Following image will be the output after the successful execution of the above mentioned program −
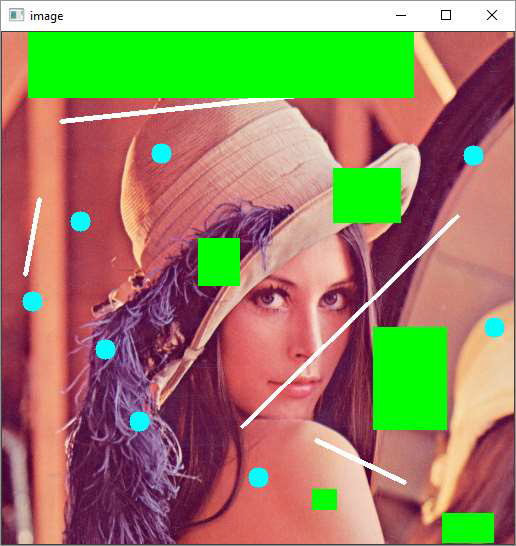