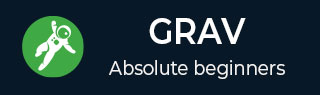
- Grav Basics Tutorial
- Grav - Home
- Grav - Overview
- Grav - Installation
- Grav Content
- Grav - Pages
- Grav - Markdown Syntax
- Grav - Page Linking
- Grav - Image Linking
- Grav - Media
- Grav - Modular Pages
- Grav - Multi Language
- Grav Themes
- Grav - Themes Basics
- Grav - Theme Tutorial
- Grav - Twig Filters & Functions
- Grav - Theme Variables
- Grav - Asset Manager
- Grav - Theme Customization
- Grav Administration Panel
- Grav - Admin Introduction
- Grav - Admin Dashboard
- Grav - Configuration System
- Grav - Configuration Site
- Grav - Administration Panel Pages
- Grav - Page Editor Options
- Grav - Page Editor Advanced
- Grav Advanced
- Grav - Blueprints
- Grav - Performance & Caching
- Grav - Debugging & Logging
- Grav - CLI
- Grav - GPM
- Grav - Development
- Grav - Lifecycle
- Grav - YAML Syntax
- Grav - Forms
- Grav Hosting
- Grav - Web Hostings
- Grav Troubleshooting
- Grav - Server Error
- Grav - Permission
- Grav Useful Resources
- Grav - Interview Questions
- Grav - Quick Guide
- Grav - Useful Resources
- Grav - Discussion
Grav - Plugin Tutorials
In this chapter, we will delve into how a plugin can be set up and configured. In addition, we will also understand the structure of a plugin and how to display a random page. Plugin is a piece of software that provides additional functionality which was not originally completed by Grav's core functionality.
In this article, we are going to display random page using the random plugin. Before using this plugin, we will see some important points of the random plugin.
You can use this plugin to display the random page by using URI as /random.
Create the filter to make use of taxonomy specified in the pages. You can create as category : blog.
You can display a random page by using the filter option; this informs Grav to use the same content that is to be displayed in the random page.
Setup Plugin
Follow these steps to create a basic setup for plugin before using the actual plugin.
Create folder called random under the user/plugins folder.
Under the user/plugins/random folder, create two files namely −
random.php used for plugin code
random.yaml used for the configuration
Plugin Configuration
To use the random plugin, we need to have some configuration options. We will write the following lines under the random.yaml file.
enabled:true route:/random filters: category:blog
Random creates a route that you define. Based on taxonomy filters, it picks a random item. The default value of the filter is 'category: blog' which is used for random selection.
Plugin Structure
The following code can be used in the plugin structure.
<?php namespace Grav\Plugin; use Grav\Common\Page\Collection; use Grav\Common\Plugin; use Grav\Common\Uri; use Grav\Common\Taxonomy; class RandomPlugin extends Plugin { } ?>
We are using a bunch of classes in the plugin using the use statements which makes it more readable and saves on space too. The namespace Grav\Plugin must be written at the top of the PHP file. The plugin name should be written in titlecase and should be extended using Plugin.
You can subscribe the function getSubscribedEvents() to the onPluginsInitialized event; this determines which events the plugin is subscribed to. Like this, you can use the event to subscribe to other events.
public static function getSubscribedEvents() { return [ 'onPluginsInitialized' => ['onPluginsInitialized', 0], ]; }
Let us now use the onPluginInitialized event under the RandomPlugin class used for routing the page which is configured in the random.yaml file.
The method onPluginInitialized() contains the following code −
public function onPluginsInitialized() { $uri = $this->grav['uri']; $route = $this->config->get('plugins.random.route'); if ($route && $route == $uri->path()) { $this->enable([ 'onPageInitialized' => ['onPageInitialized', 0] ]); } }
The Uri object includes the current uri, information about route. The config object specifies the configuration value for routing the random plugin and store it in the route object.
We will now compare the configured route with the current URI path which informs the plugin to listen to the onPageInitialized event.
Displaying Random Page
You can display the random page by using the code with the following method −
public function onPageInitialized() { $taxonomy_map = $this->grav['taxonomy']; $filters = (array) $this->config->get('plugins.random.filters'); $operator = $this->config->get('plugins.random.filter_combinator', 'and'); if (count($filters)) { $collection = new Collection(); $collection->append($taxonomy_map->findTaxonomy($filters, $operator)->toArray()); if (count($collection)) { unset($this->grav['page']); $this->grav['page'] = $collection->random()->current(); } } }
As shown in the code,
Assign the taxonomy object to the variable $taxonomy_map.
Get the array of filter which uses configured taxonomy from the plugin configuration using config object. We are using the item as category : blog.
We are using collection to store the random page in the $collection. Append the page which matches the filter to $collection variable.
Unset the current page object and set the current page to display as random page in the collection.
Finally, we will see the complete code of plugin to display a random page as shown below −
<?php namespace Grav\Plugin; use Grav\Common\Page\Collection; use Grav\Common\Plugin; use Grav\Common\Uri; use Grav\Common\Taxonomy; class RandomPlugin extends Plugin { public static function getSubscribedEvents() { return [ 'onPluginsInitialized' => ['onPluginsInitialized', 0], ]; } public function onPluginsInitialized() { $uri = $this->grav['uri']; $route = $this->config->get('plugins.random.route'); if ($route && $route == $uri->path()) { $this->enable([ 'onPageInitialized' => ['onPageInitialized', 0] ]); } } public function onPageInitialized() { $taxonomy_map = $this->grav['taxonomy']; $filters = (array) $this->config->get('plugins.random.filters'); $operator = $this->config->get('plugins.random.filter_combinator', 'and'); if (count($filters)) { $collection = new Collection(); $collection->append($taxonomy_map->findTaxonomy($filters, $operator)->toArray()); if (count($collection)) { unset($this->grav['page']); $this->grav['page'] = $collection->random()->current(); } } } }
Open your browser and type localhost/folder_name/random to see the random page as shown in the following screenshot −
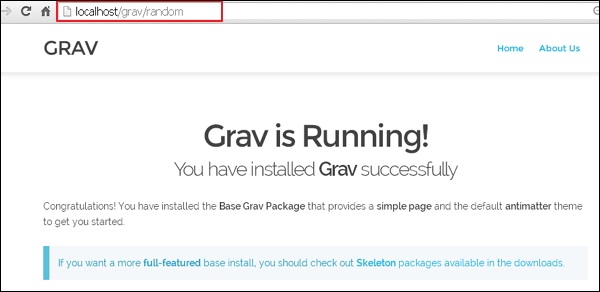