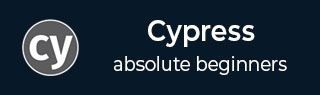
- Cypress Tutorial
- Cypress - Home
- Cypress - Introduction
- Cypress - Architecture and Environment Setup
- Cypress - Test Runner
- Cypress - Build First Test
- Cypress - Supported Browsers
- Cypress - Basic Commands
- Cypress - Variables
- Cypress - Aliases
- Cypress - Locators
- Cypress - Assertions
- Cypress - Text Verification
- Cypress - Asynchronous Behavior
- Cypress - Working with XHR
- Cypress - jQuery
- Cypress - Checkbox
- Cypress - Tabs
- Cypress - Dropdown
- Cypress - Alerts
- Cypress - Child Windows
- Cypress - Hidden Elements
- Cypress - Frames
- Cypress - Web Tables
- Cypress - Mouse Actions
- Cypress - Cookies
- Cypress - Get and Post
- Cypress - File Upload
- Cypress - Data Driven Testing
- Cypress - Prompt Pop-up Window
- Cypress - Dashboards
- Cypress - Screenshots and Videos
- Cypress - Debugging
- Cypress - Custom Commands
- Cypress - Fixtures
- Cypress - Environment Variables
- Cypress - Hooks
- Cypress - Configuration of JSON File
- Cypress - Reports
- Cypress - Plugins
- Cypress - GitHub
- Cypress Useful Resources
- Cypress - Quick Guide
- Cypress - Useful Resources
- Cypress - Discussion
Cypress - Data Driven Testing
Cypress data driven testing is achieved with the help of fixtures. Cypress fixtures are added to maintain and hold the test data for automation.
The fixtures are kept inside the fixtures folder (example.json file) in the Cypress project.Basically, it helps us to get data input from external files.

Cypress fixtures folder can have files in JavaScript Object Notation (JSON) or other formats and the data is maintained in "key:value" pairs.
All the test data can be utilised by more than one test. All fixture data has to be declared within the before hook block.
Syntax
The syntax for Cypress data driven testing is as follows −
cy.fixture(path of test data) cy.fixture(path of test data, encoding type) cy.fixture(path of test data, opts) cy.fixture(path of test data, encoding type, options)
Here,
path of test data is the path of test data file within fixtures folder.
encoding type − Encoding type (utf-8, asci, and so on) is used to read the file.
Opts − Modifies the timeout for response. The default value is 30000ms. The wait time for cy.fixture(), prior throws an exception.
Implementation in example.json
Given below is the implementation of data driven testing with example.json in Cypress −
{ "email": "abctest@gmail.com", "password": "Test@123" }
Implementation of Actual Test
The implementation of actual data driven testing in Cypress is as follows −
describe('Tutorialspoint Test', function () { //part of before hook before(function(){ //access fixture data cy.fixture('example').then(function(signInData){ this.signInData = signInData }) }) // test case it('Test Case1', function (){ // launch URL cy.visit("https://www.linkedin.com/") //data driven from fixture cy.get('#session_key ') .type(this.signInData.email) cy.get('# session_password').type(this.signInData.password) }); });
Execution Results
The output is as follows
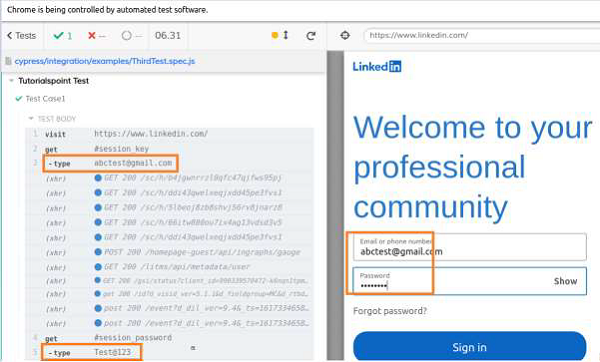
The output logs show the values abctest@gmail.com and Test@123 being fed to the Email and Password fields respectively. These data have been passed to the test from the fixtures.