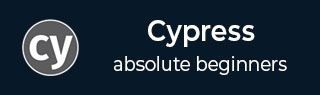
- Cypress Tutorial
- Cypress - Home
- Cypress - Introduction
- Cypress - Architecture and Environment Setup
- Cypress - Test Runner
- Cypress - Build First Test
- Cypress - Supported Browsers
- Cypress - Basic Commands
- Cypress - Variables
- Cypress - Aliases
- Cypress - Locators
- Cypress - Assertions
- Cypress - Text Verification
- Cypress - Asynchronous Behavior
- Cypress - Working with XHR
- Cypress - jQuery
- Cypress - Checkbox
- Cypress - Tabs
- Cypress - Dropdown
- Cypress - Alerts
- Cypress - Child Windows
- Cypress - Hidden Elements
- Cypress - Frames
- Cypress - Web Tables
- Cypress - Mouse Actions
- Cypress - Cookies
- Cypress - Get and Post
- Cypress - File Upload
- Cypress - Data Driven Testing
- Cypress - Prompt Pop-up Window
- Cypress - Dashboards
- Cypress - Screenshots and Videos
- Cypress - Debugging
- Cypress - Custom Commands
- Cypress - Fixtures
- Cypress - Environment Variables
- Cypress - Hooks
- Cypress - Configuration of JSON File
- Cypress - Reports
- Cypress - Plugins
- Cypress - GitHub
- Cypress Useful Resources
- Cypress - Quick Guide
- Cypress - Useful Resources
- Cypress - Discussion
Cypress - Cookies
Cypress handles cookies with the methods Cookies.preserveOnce() and Cookies.defaults(). The method Cookies.debug() produces logs to the console, if there are any changes to the cookies.
By default, Cypress removes all cookies prior to each test execution.We can utilise Cypress.Cookies.preserveOnce() to preserve the cookies with their names to be used for other tests.
Syntax
The syntax for the commands related to the cookies in Cypress are as follows −
This will produce console logs, if cookie values are configured or cleared.
Cypress.Cookies.debug(enable, option)
Here,
enable – if debug of cookie should be enabled.
option – configure default values for cookies, for example, preserve cookies.
Cypress.Cookies.debug(true) // logs will generate if cookies are modified cy.clearCookie('cookie1') cy.setCookie('cookie2', 'val')
To reduce the level of logging.
Cypress.Cookies.debug(true, { verbose: false }) Cypress.Cookies.debug(false) // logs will not generate if cookies are modified
The syntax given below will preserve the cookies and they will not be cleared prior execution of another test.
Cypress.Cookies.preserveOnce(cookie names...)
This syntax is used to modify global configuration and to maintain a group of cookies that are preserved for a test. Any modification will be applicable for that particular test.(maintained in cypress/support/index.js file and are loaded prior to test execution).
Cypress.Cookies.defaults(option) Cypress.Cookies.defaults({ preserve: 'cookie1' })
Here, the cookie named cookie1 will not be cleared before running the test.
Cookie Methods
Some of the cookie methods in Cypress are as follows −
cy.clearCookies() − It removes all the cookies from present domain and subdomain.
cy.clearCookie(name) − It removes a cookie from the browser by name.
cy.getCookie(name) − It is used to obtain a cookie from the browser by name.
cy.getCookies() − It is used to obtain all the cookies.
cy.setCookie(name) − It can configure a cookie.
Implementation
Given below is the implementation of the cookie methods in Cypress −
describe('Tutorialspoint Test', function () { // test case it('Scenario 1', function (){ // launch the application cy.visit("https://accounts.google.com"); // enable cookie logging Cypress.Cookies.debug(true) //set cookie cy.setCookie('cookie1', 'value1') //get cookie by name and verify value cy.getCookie('cookie1').should('have.property', 'value', 'value1') //clear cookie by name cy.clearCookie('cookie') //get all cookies cy.getCookies() //clear all cookies cy.clearCookies() //verify no cookies cy.getCookies().should('be.empty') }); });
Execution Results
The output is mentioned below −
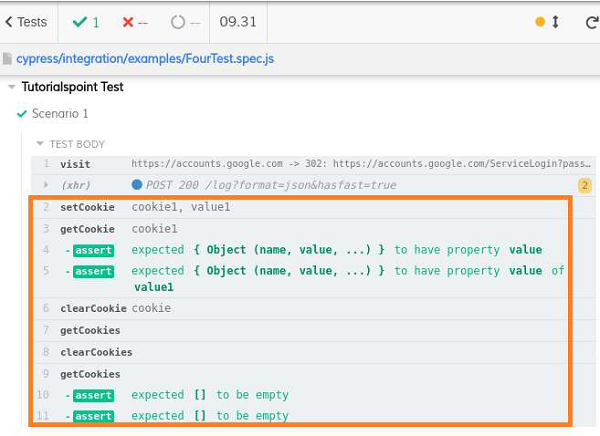