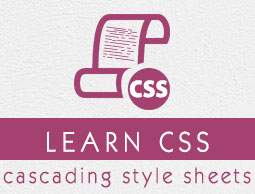
- CSS Tutorial
- CSS - Home
- CSS - Introduction
- CSS - Syntax
- CSS - Selectors
- CSS - Inclusion
- CSS - Measurement Units
- CSS - Colors
- CSS - Backgrounds
- CSS - Fonts
- CSS - Text
- CSS - Images
- CSS - Links
- CSS - Tables
- CSS - Borders
- CSS - Border Block
- CSS - Border Inline
- CSS - Margins
- CSS - Lists
- CSS - Padding
- CSS - Cursor
- CSS - Outlines
- CSS - Dimension
- CSS - Scrollbars
- CSS - Inline Block
- CSS - Dropdowns
- CSS - Visibility
- CSS - Overflow
- CSS - Clearfix
- CSS - Float
- CSS - Arrows
- CSS - Resize
- CSS - Quotes
- CSS - Order
- CSS - Position
- CSS - Hyphens
- CSS - Hover
- CSS - Display
- CSS - Focus
- CSS - Zoom
- CSS - Translate
- CSS - Height
- CSS - Hyphenate Character
- CSS - Width
- CSS - Opacity
- CSS - Z-Index
- CSS - Bottom
- CSS - Navbar
- CSS - Overlay
- CSS - Forms
- CSS - Align
- CSS - Icons
- CSS - Image Gallery
- CSS - Comments
- CSS - Loaders
- CSS - Attr Selectors
- CSS - Combinators
- CSS - Root
- CSS - Box Model
- CSS - Counters
- CSS - Clip
- CSS - Writing Mode
- CSS - Unicode-bidi
- CSS - min-content
- CSS - All
- CSS - Inset
- CSS - Isolation
- CSS - Overscroll
- CSS - Justify Items
- CSS - Justify Self
- CSS - Tab Size
- CSS - Pointer Events
- CSS - Place Content
- CSS - Place Items
- CSS - Place Self
- CSS - Max Block Size
- CSS - Min Block Size
- CSS - Mix Blend Mode
- CSS - Max Inline Size
- CSS - Min Inline Size
- CSS - Offset
- CSS - Accent Color
- CSS - User Select
- CSS Advanced
- CSS - Grid
- CSS - Grid Layout
- CSS - Flexbox
- CSS - Visibility
- CSS - Positioning
- CSS - Layers
- CSS - Pseudo Classes
- CSS - Pseudo Elements
- CSS - @ Rules
- CSS - Text Effects
- CSS - Paged Media
- CSS - Printing
- CSS - Layouts
- CSS - Validations
- CSS - Image Sprites
- CSS - Important
- CSS - Data Types
- CSS3 Tutorial
- CSS3 - Tutorial
- CSS - Rounded Corner
- CSS - Border Images
- CSS - Multi Background
- CSS - Color
- CSS - Gradients
- CSS - Box Shadow
- CSS - Box Decoration Break
- CSS - Caret Color
- CSS - Text Shadow
- CSS - Text
- CSS - 2d transform
- CSS - 3d transform
- CSS - Transition
- CSS - Animation
- CSS - Multi columns
- CSS - Box Sizing
- CSS - Tooltips
- CSS - Buttons
- CSS - Pagination
- CSS - Variables
- CSS - Media Queries
- CSS - Functions
- CSS - Math Functions
- CSS - Masking
- CSS - Shapes
- CSS - Style Images
- CSS - Specificity
- CSS - Custom Properties
- CSS Responsive
- CSS RWD - Introduction
- CSS RWD - Viewport
- CSS RWD - Grid View
- CSS RWD - Media Queries
- CSS RWD - Images
- CSS RWD - Videos
- CSS RWD - Frameworks
- CSS References
- CSS - Questions and Answers
- CSS - Quick Guide
- CSS - References
- CSS - Color References
- CSS - Web browser References
- CSS - Web safe fonts
- CSS - Units
- CSS - Animation
- CSS Resources
- CSS - Useful Resources
- CSS - Discussion
CSS Flexbox - justify-content Property
CSS justify-content property determines the alignment and allocation of space between content items along the main axis of a flex container or the inline axis of a grid container.
Possible Values
-
start − The items are placed closely together at the starting edge of the container.
-
end − The items are placed closely together, starting from the end of the container.
-
center − The items are placed closely together from the center of the container.
-
flex-start − Aligns the flex items at the start of the flex container. This only applies to flex layout items.
-
flex-end − Aligns the flex items at the end of the flex container. This only applies to flex layout items
-
left − Aligns the flex items to the left side of the main axis of a flex container. When flex-direction: column; is used, when the property's horizontal axis is not parallel with the inline axis, this value functions similarly to start.
-
right − Aligns the flex items to the right side of the main axis of a flex container. This value functions like start, when the property's axis is not parallel with the inline axis (in a grid container) or the main-axis (in a flexbox container).
-
normal − This behaves like a stretch but in multi-column containers with a specified column-width, columns maintain their width instead of stretching to fill the container.
-
space-between − It evenly distributes space between the flex items along the main axis of a flex container. Each pair of adjacent elements has the same spacing. The first and last items are flush with the main-start edge and the main-end edge, respectively.
-
space-around − It evenly distributes space around the flex items along the main axis of a flex container. Each pair of adjacent elements has the same spacing. The spacing before the first and after the last item is equal to half the space between each pair of adjacent items.
-
space-evenly − It evenly distributes the space around and between the flex items along the main axis of a flex container. The adjacent items have the same spacing, the main-start edge, and the first item, as well as the main-end edge and the last item.
-
stretch − If the total size of items along the main axis is smaller than the alignment container, auto-sized items increase their size equally to fill the container, respecting max-height/max-width constraints.
Applies to
Flex containers
Syntax
Positional Alignment
justify-content: start; justify-content: end; justify-content: center; justify-content: flex-start; justify-content: flex-end; justify-content: left; justify-content: right;
Normal Alignment
justify-content: normal;
Distributed Alignment
justify-content: space-between; justify-content: space-around; justify-content: space-evenly; justify-content: stretch;
CSS justify-content - start Value
The following example demonstrates property justify-content: start, aligns the flex items at the start of the flex container −
<html> <head> <style> .flex-container { display: flex; justify-content: start; background-color: green; } .flex-container div { background-color: yellow; padding: 10px; margin: 5px; width: 75px; height: 50px; } </style> </head> <body> <div class="flex-container"> <div>Flex item 1</div> <div>Flex item 2</div> <div>Flex item 3</div> </div> </body> </html>
CSS justify-content - end Value
The following example demonstrates property justify-content: end aligns the flex items at the end of the flex container −
<html> <head> <style> .flex-container { display: flex; justify-content: end; background-color: green; } .flex-container div { background-color: yellow; padding: 10px; margin: 5px; width: 75px; height: 50px; } </style> </head> <body> <div class="flex-container"> <div>Flex item 1</div> <div>Flex item 2</div> <div>Flex item 3</div> </div> </body> </html>
CSS justify-content - center Value
The following example demonstrates property justify-content: center aligns the flex items at the center of the flex container −
<html> <head> <style> .flex-container { display: flex; justify-content: center; background-color: green; } .flex-container div { background-color: yellow; padding: 10px; margin: 5px; width: 75px; height: 50px; } </style> </head> <body> <div class="flex-container"> <div>Flex item 1</div> <div>Flex item 2</div> <div>Flex item 3</div> </div> </body> </html>
CSS justify-content - flex-start Value
The following example demonstrates property justify-content: flex-start aligns the flex items at the start of the flex container −
<html> <head> <style> .flex-container { display: flex; justify-content: flex-start; background-color: green; } .flex-container div { background-color: yellow; padding: 10px; margin: 5px; width: 75px; height: 50px; } </style> </head> <body> <div class="flex-container"> <div>Flex item 1</div> <div>Flex item 2</div> <div>Flex item 3</div> </div> </body> </html>
CSS justify-content - flex-end Value
The following example demonstrates property justify-content: flex-end aligns the flex items at the end of the flex container −
<html> <head> <style> .flex-container { display: flex; justify-content: flex-end; background-color: green; } .flex-container div { background-color: yellow; padding: 10px; margin: 5px; width: 75px; height: 50px; } </style> </head> <body> <div class="flex-container"> <div>Flex item 1</div> <div>Flex item 2</div> <div>Flex item 3</div> </div> </body> </html>
CSS justify-content - left Value
The following example demonstrates property justify-content: left aligns the flex items to the left side of the main axis of a flex container −
<html> <head> <style> .flex-container { display: flex; justify-content: left; background-color: green; } .flex-container div { background-color: yellow; padding: 10px; margin: 5px; width: 75px; height: 50px; } </style> </head> <body> <div class="flex-container"> <div>Flex item 1</div> <div>Flex item 2</div> <div>Flex item 3</div> </div> </body> </html>
CSS justify-content - right Value
The following example demonstrates property justify-content: right aligns the flex items to the right side of the main axis of a flex container −
<html> <head> <style> .flex-container { display: flex; justify-content: right; background-color: green; } .flex-container div { background-color: yellow; padding: 10px; margin: 5px; width: 75px; height: 50px; } </style> </head> <body> <div class="flex-container"> <div>Flex item 1</div> <div>Flex item 2</div> <div>Flex item 3</div> </div> </body> </html>
CSS justify-content - space-between Value
The following example demonstrates property justify-content: space-between evenly distributes space between the flex items along the main axis of a flex container −
<html> <head> <style> .my-flex-container { display: flex; justify-content: space-between; background-color: #0ca14a; } .my-flex-container div { background-color: #FBFF22; padding: 10px; margin: 5px; width: 75px; height: 50px; } </style> </head> <body> <div class="my-flex-container"> <div>Flex item 1</div> <div>Flex item 2</div> <div>Flex item 3</div> </div> </body> </html>
CSS justify-content - space-around Value
The following example demonstrates property justify-content: space-around evenly distributes space around the flex items along the main axis of a flex container −
<html> <head> <style> .flex-container { display: flex; justify-content: space-around; background-color: green; } .flex-container div { background-color: yellow; padding: 10px; margin: 5px; width: 75px; height: 50px; } </style> </head> <body> <div class="flex-container"> <div>Flex item 1</div> <div>Flex item 2</div> <div>Flex item 3</div> </div> </body> </html>
CSS justify-content - space-evenly Value
The following example demonstrates property justify-content: space-evenly evenly distributes the space around and between the flex items along the main axis of a flex container −
<html> <head> <style> .flex-container { display: flex; justify-content: space-evenly; background-color: green; } .flex-container div { background-color: yellow; padding: 10px; margin: 5px; width: 75px; height: 50px; } </style> </head> <body> <div class="flex-container"> <div>Flex item 1</div> <div>Flex item 2</div> <div>Flex item 3</div> </div> </body> </html>
CSS justify-content - stretch Value
The following example demonstrates property justify-content: stretch stretches the flex items along the main axis of a flex container −
<html> <head> <style> .flex-container { display: flex; background-color: green; height: 350px; flex-wrap: wrap; justify-content: stretch; } .flex-container div { background-color: yellow; padding: 10px; margin: 5px; width: 90px; } </style> </head> <body> <div class="flex-container"> <div>Flex item 1</div> <div>Flex item 2</div> <div>Flex item 3</div> </div> </body> </html>