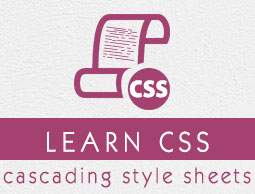
- CSS Tutorial
- CSS - Home
- CSS - Introduction
- CSS - Syntax
- CSS - Selectors
- CSS - Inclusion
- CSS - Measurement Units
- CSS - Colors
- CSS - Backgrounds
- CSS - Fonts
- CSS - Text
- CSS - Images
- CSS - Links
- CSS - Tables
- CSS - Borders
- CSS - Border Block
- CSS - Border Inline
- CSS - Margins
- CSS - Lists
- CSS - Padding
- CSS - Cursor
- CSS - Outlines
- CSS - Dimension
- CSS - Scrollbars
- CSS - Inline Block
- CSS - Dropdowns
- CSS - Visibility
- CSS - Overflow
- CSS - Clearfix
- CSS - Float
- CSS - Arrows
- CSS - Resize
- CSS - Quotes
- CSS - Order
- CSS - Position
- CSS - Hyphens
- CSS - Hover
- CSS - Display
- CSS - Focus
- CSS - Zoom
- CSS - Translate
- CSS - Height
- CSS - Hyphenate Character
- CSS - Width
- CSS - Opacity
- CSS - Z-Index
- CSS - Bottom
- CSS - Navbar
- CSS - Overlay
- CSS - Forms
- CSS - Align
- CSS - Icons
- CSS - Image Gallery
- CSS - Comments
- CSS - Loaders
- CSS - Attr Selectors
- CSS - Combinators
- CSS - Root
- CSS - Box Model
- CSS - Counters
- CSS - Clip
- CSS - Writing Mode
- CSS - Unicode-bidi
- CSS - min-content
- CSS - All
- CSS - Inset
- CSS - Isolation
- CSS - Overscroll
- CSS - Justify Items
- CSS - Justify Self
- CSS - Tab Size
- CSS - Pointer Events
- CSS - Place Content
- CSS - Place Items
- CSS - Place Self
- CSS - Max Block Size
- CSS - Min Block Size
- CSS - Mix Blend Mode
- CSS - Max Inline Size
- CSS - Min Inline Size
- CSS - Offset
- CSS - Accent Color
- CSS - User Select
- CSS Advanced
- CSS - Grid
- CSS - Grid Layout
- CSS - Flexbox
- CSS - Visibility
- CSS - Positioning
- CSS - Layers
- CSS - Pseudo Classes
- CSS - Pseudo Elements
- CSS - @ Rules
- CSS - Text Effects
- CSS - Paged Media
- CSS - Printing
- CSS - Layouts
- CSS - Validations
- CSS - Image Sprites
- CSS - Important
- CSS - Data Types
- CSS3 Tutorial
- CSS3 - Tutorial
- CSS - Rounded Corner
- CSS - Border Images
- CSS - Multi Background
- CSS - Color
- CSS - Gradients
- CSS - Box Shadow
- CSS - Box Decoration Break
- CSS - Caret Color
- CSS - Text Shadow
- CSS - Text
- CSS - 2d transform
- CSS - 3d transform
- CSS - Transition
- CSS - Animation
- CSS - Multi columns
- CSS - Box Sizing
- CSS - Tooltips
- CSS - Buttons
- CSS - Pagination
- CSS - Variables
- CSS - Media Queries
- CSS - Functions
- CSS - Math Functions
- CSS - Masking
- CSS - Shapes
- CSS - Style Images
- CSS - Specificity
- CSS - Custom Properties
- CSS Responsive
- CSS RWD - Introduction
- CSS RWD - Viewport
- CSS RWD - Grid View
- CSS RWD - Media Queries
- CSS RWD - Images
- CSS RWD - Videos
- CSS RWD - Frameworks
- CSS References
- CSS - Questions and Answers
- CSS - Quick Guide
- CSS - References
- CSS - Color References
- CSS - Web browser References
- CSS - Web safe fonts
- CSS - Units
- CSS - Animation
- CSS Resources
- CSS - Useful Resources
- CSS - Discussion
CSS Flexbox - flex-flow Property
CSS flex-flow is a shorthand property that determines the direction of a flex container and the wrapping behavior of its content.
This property enables flex items to wrap onto multiple lines in a horizontal row when they exceed the container's width.
The flex-flow property is a shorthand for the following CSS properties:
Possible Values
-
row − The flex items are displayed in a horizontal direction from left to right.
-
row-reverse − The flex items are displayed in a horizontal direction but in reverse order from right to left.
-
column − The flex items are displayed in a vertical direction from top to bottom.
-
column-reverse − The flex items are displayed in a vertical direction but in reverse order from bottom to top.
-
nowrap − The flex items should not wrap or break into the next line.
-
wrap − The flex item should wrap to the next line.
-
wrap-reverse − The flex items should wrap to the next line in reverse order.
Applies to
Flex containers.
Syntax
<flex-direction>
flex-flow: row; flex-flow: row-reverse; flex-flow: column; flex-flow: column-reverse;
<flex-wrap>
flex-flow: nowrap; flex-flow: wrap; flex-flow: wrap-reverse;
<flex-direction> and <flex-wrap>
flex-flow: row nowrap; flex-flow: column wrap; flex-flow: column-reverse wrap-reverse;
CSS flex-flow - row Value
The following example demonstrates use of flex-flow: row property. The flex items display in a horizontal direction −
<html> <head> <style> .flex-container { display: flex; flex-flow: row; background-color: green; } .flex-container div { background-color: yellow; padding: 10px; margin: 5px; width: 75px; height: 50px; } </style> </head> <body> <div class="flex-container"> <div>Flex item 1</div> <div>Flex item 2</div> <div>Flex item 3</div> <div>Flex item 4</div> <div>Flex item 5</div> <div>Flex item 6</div> <div>Flex item 7</div> <div>Flex item 8</div> </div> </body> </html>
CSS flex-flow - row-reverse Value
The following example demonstrates that the flex-flow: row property arranges flex items in a horizontal direction from right to left −
<html> <head> <style> .flex-container { display: flex; flex-flow: row-reverse; background-color: green; } .flex-container div { background-color: yellow; padding: 10px; margin: 5px; width: 75px; height: 50px; } </style> </head> <body> <div class="flex-container"> <div>Flex item 1</div> <div>Flex item 2</div> <div>Flex item 3</div> <div>Flex item 4</div> <div>Flex item 5</div> <div>Flex item 6</div> <div>Flex item 7</div> <div>Flex item 8</div> </div> </body> </html>
CSS flex-flow - column Value
The following example demonstrates that the flex-flow: column property arranges flex items in a vertical direction −
<html> <head> <style> .flex-container { display: flex; flex-flow: column; background-color: green; width: 100%; } .flex-container div { background-color: yellow; padding: 10px; margin: 5px; width: 75px; height: 50px; } </style> </head> <body> <div class="flex-container"> <div>Flex item 1</div> <div>Flex item 2</div> <div>Flex item 3</div> <div>Flex item 4</div> <div>Flex item 5</div> </div> </body> </html>
CSS flex-flow - column-reverse Value
The following example demonstrates that the flex-flow: column property arranges flex items in a vertical direction but displays in a reverse order from bottom to top −
<html> <head> <style> .flex-container { display: flex; flex-flow: column-reverse; background-color: green; width: 100%; } .flex-container div { background-color: yellow; padding: 10px; margin: 5px; width: 75px; height: 50px; } </style> </head> <body> <div class="flex-container"> <div>Flex item 1</div> <div>Flex item 2</div> <div>Flex item 3</div> <div>Flex item 4</div> <div>Flex item 5</div> </div> </body> </html>
CSS flex-flow - nowrap Value
The following example demonstrates that flex-flow: nowrap property prevents the flex items from wrapping to the next line −
<html> <head> <style> .flex-container { display: flex; flex-flow: nowrap; background-color: green; } .flex-container div { background-color: yellow; padding: 10px; margin: 5px; width: 75px; height: 50px; } </style> </head> <body> <h4>As you resize the browser window the flex items are displayed in a single row without wrapping.</h4> <div class="flex-container"> <div>Flex item 1</div> <div>Flex item 2</div> <div>Flex item 3</div> <div>Flex item 4</div> <div>Flex item 5</div> </div> </body> </html>
CSS flex-flow - wrap Value
The following example demonstrates use of flex-flow: wrap property. Here the flex items wraps to the next line −
<html> <head> <style> .flex-container { display: flex; flex-flow: wrap; background-color: green; width: 100%; } .flex-container div { background-color: yellow; padding: 10px; margin: 5px; width: 75px; height: 50px; } </style> </head> <body> <h4>As you rsize the browser window the flex items should be wrap to the next line.</h4> <div class="flex-container"> <div>Flex item 1</div> <div>Flex item 2</div> <div>Flex item 3</div> <div>Flex item 4</div> <div>Flex item 5</div> <div>Flex item 6</div> <div>Flex item 7</div> </div> </body> </html>
CSS flex-flow - wrap-reverse Value
The following example demonstrates use of flex-flow: wrap-reverse property. Here the flex items wraps to the next line in reverse order −
<html> <head> <style> .flex-container { display: flex; flex-flow: wrap-reverse; background-color: green; width: 100%; } .flex-container div { background-color: yellow; padding: 10px; margin: 5px; width: 75px; height: 50px; } </style> </head> <body> <h4>As you rsize the browser window the flex items should be wrap to the next line in reverse order.</h4> <div class="flex-container"> <div>Flex item 1</div> <div>Flex item 2</div> <div>Flex item 3</div> <div>Flex item 4</div> <div>Flex item 5</div> <div>Flex item 6</div> <div>Flex item 7</div> </div> </body> </html>
CSS flex-flow - row wrap Value
The following example demonstrates that flex-flow: row wrap property arranges the flex items in a row and wraps to the next line −
<html> <head> <style> .flex-container { display: flex; flex-flow: row wrap; background-color: green; width: 100%; } .flex-container div { background-color: yellow; padding: 10px; margin: 5px; width: 75px; height: 50px; } </style> </head> <body> <h4>As you rsize the browser window the flex items should be wrap to the next line.</h4> <div class="flex-container"> <div>Flex item 1</div> <div>Flex item 2</div> <div>Flex item 3</div> <div>Flex item 4</div> <div>Flex item 5</div> <div>Flex item 6</div> <div>Flex item 7</div> </div> </body> </html>