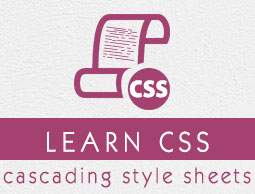
- CSS Tutorial
- CSS - Home
- CSS - Introduction
- CSS - Syntax
- CSS - Selectors
- CSS - Inclusion
- CSS - Measurement Units
- CSS - Colors
- CSS - Backgrounds
- CSS - Fonts
- CSS - Text
- CSS - Images
- CSS - Links
- CSS - Tables
- CSS - Borders
- CSS - Border Block
- CSS - Border Inline
- CSS - Margins
- CSS - Lists
- CSS - Padding
- CSS - Cursor
- CSS - Outlines
- CSS - Dimension
- CSS - Scrollbars
- CSS - Inline Block
- CSS - Dropdowns
- CSS - Visibility
- CSS - Overflow
- CSS - Clearfix
- CSS - Float
- CSS - Arrows
- CSS - Resize
- CSS - Quotes
- CSS - Order
- CSS - Position
- CSS - Hyphens
- CSS - Hover
- CSS - Display
- CSS - Focus
- CSS - Zoom
- CSS - Translate
- CSS - Height
- CSS - Hyphenate Character
- CSS - Width
- CSS - Opacity
- CSS - Z-Index
- CSS - Bottom
- CSS - Navbar
- CSS - Overlay
- CSS - Forms
- CSS - Align
- CSS - Icons
- CSS - Image Gallery
- CSS - Comments
- CSS - Loaders
- CSS - Attr Selectors
- CSS - Combinators
- CSS - Root
- CSS - Box Model
- CSS - Counters
- CSS - Clip
- CSS - Writing Mode
- CSS - Unicode-bidi
- CSS - min-content
- CSS - All
- CSS - Inset
- CSS - Isolation
- CSS - Overscroll
- CSS - Justify Items
- CSS - Justify Self
- CSS - Tab Size
- CSS - Pointer Events
- CSS - Place Content
- CSS - Place Items
- CSS - Place Self
- CSS - Max Block Size
- CSS - Min Block Size
- CSS - Mix Blend Mode
- CSS - Max Inline Size
- CSS - Min Inline Size
- CSS - Offset
- CSS - Accent Color
- CSS - User Select
- CSS Advanced
- CSS - Grid
- CSS - Grid Layout
- CSS - Flexbox
- CSS - Visibility
- CSS - Positioning
- CSS - Layers
- CSS - Pseudo Classes
- CSS - Pseudo Elements
- CSS - @ Rules
- CSS - Text Effects
- CSS - Paged Media
- CSS - Printing
- CSS - Layouts
- CSS - Validations
- CSS - Image Sprites
- CSS - Important
- CSS - Data Types
- CSS3 Tutorial
- CSS3 - Tutorial
- CSS - Rounded Corner
- CSS - Border Images
- CSS - Multi Background
- CSS - Color
- CSS - Gradients
- CSS - Box Shadow
- CSS - Box Decoration Break
- CSS - Caret Color
- CSS - Text Shadow
- CSS - Text
- CSS - 2d transform
- CSS - 3d transform
- CSS - Transition
- CSS - Animation
- CSS - Multi columns
- CSS - Box Sizing
- CSS - Tooltips
- CSS - Buttons
- CSS - Pagination
- CSS - Variables
- CSS - Media Queries
- CSS - Functions
- CSS - Math Functions
- CSS - Masking
- CSS - Shapes
- CSS - Style Images
- CSS - Specificity
- CSS - Custom Properties
- CSS Responsive
- CSS RWD - Introduction
- CSS RWD - Viewport
- CSS RWD - Grid View
- CSS RWD - Media Queries
- CSS RWD - Images
- CSS RWD - Videos
- CSS RWD - Frameworks
- CSS References
- CSS - Questions and Answers
- CSS - Quick Guide
- CSS - References
- CSS - Color References
- CSS - Web browser References
- CSS - Web safe fonts
- CSS - Units
- CSS - Animation
- CSS Resources
- CSS - Useful Resources
- CSS - Discussion
CSS grid - grid-auto-columns Property
The CSS property grid-auto-columns defines the width of grid columns that are created implicitly or in a pattern without explicit declaration.
If a grid item is placed in a column that isn't explicitly dimensioned by grid-template-columns, the grid system creates implicit grid columns to accommodate it.
This happens if the item is explicitly positioned in a column outside the defined area or if the auto- placement algorithm generates additional columns to accommodate the content.
Possible values
<length> - Is a non-negative length.
<percentage> - Is a <percentage> value that is non-negative in relation to the grid container's block size. The percentage value is set as auto if the grid container's block size is indefinite.
<flex> - This value is a non-negative dimension with the unit fr, which indicates the flex factor of the track. Each track that has a certain size takes up a portion of the remaining space based on its flex factor.
max-content - It's a keyword that specifies the maximum content contribution of the grid elements that occupy the grid track.
min-content - It's a keyword that specifies the largest minimum content contribution of the grid elements within the grid track.
minmax(min, max) - This function defines a size range, from min to max values. If the maximum value is smaller than the minimum value, the maximum value isn't taken into account and the function only uses the minimum value.
fit-content( [ <length> | <percentage> ] ) - Represents the expression min(max-content, max(auto, argument)), which is calculated similarly to auto (i.e. minmax(auto, max-content)), but the track size is limited to the argument if it exceeds the auto minimum.
auto - The maximum and minimum values in a track indicate the maximum and minimum content sizes (specified by min-width/min-height), respectively, and auto covers the range between them when used alone outside minmax() notation.
Syntax
grid-auto-columns = <track-size>+ <track-size> = <track-breadth> minmax(<inflexible-breadth> , <track-breadth>) | fit-content(<length-percentage [0,∞]>)
Applies to
Grid containers.
CSS grid-auto-columns - <length> Value
The following example demonstrates the usage of grid-auto-columns using length.
<html> <head> <style> body { font-family: Arial, sans-serif; margin: 0; padding: 0; } .grid-container { display: grid; grid-auto-columns: 250px; /* Change this value to see the effect */ gap: 10px; padding: 20px; background-color: #f5dbc1; } .item { background-color: #b05b05; color: white; display: flex; align-items: center; justify-content: center; font-size: 24px; border: 2px solid #333; } </style> </head> <body> <div class="grid-container"> <div class="item">Box 1</div> <div class="item">BOx 2</div> <div class="item">Box 3</div> <div class="item">Box 4</div> <div class="item">Box 5</div> <div class="item">Box 6</div> <div class="item">Box 7</div> <div class="item">Box 8</div> </div> </body> </html>
CSS grid-auto-columns - <percentage> Value
The following example demonstrates the usage of grid-auto-columns using percentage values.
<html> <head> <style> body { font-family: 'Roboto', sans-serif; margin: 0; padding: 0; height: 100vh; display: flex; align-items: center; justify-content: center; } .custom-grid { display: grid; grid-auto-columns: 65%; gap: 15px; padding: 20px; background-color: #a6e3e9; align-content: center; justify-content: center; } .custom-item { background-color: #f36886; color: #1e1e1e; display: flex; align-items: center; justify-content: center; font-size: 20px; border: 2px solid #635353; border-radius: 8px; } </style> </head> <body> <div class="custom-grid"> <div class="custom-item">Custom Box 1</div> <div class="custom-item">Custom Box 2</div> <div class="custom-item">Custom Box 3</div> <div class="custom-item">Custom Box 4</div> <div class="custom-item">Custom Box 5</div> <div class="custom-item">Custom Box 6</div> </div> </body> </html>
CSS grid-auto-columns - <flex> Value
The following example demonstrates the usage of grid-auto-columns using flex value that is fr.
<html> <head> <style> body { background-color: #F8ECE5; font-family: 'Segoe UI', Tahoma, Geneva, Verdana, sans-serif; margin: 0; padding: 0; } #unique-grid { display: grid; grid-template-rows: 150px 150px; grid-template-columns: fit-content(120px); grid-auto-columns: 1fr 0.5fr; grid-auto-flow: column; grid-gap: 20px; margin: 20px; } #unique-grid > div { background-color: #FF6F61; text-align: center; color: white; font-size: 22px; padding: 20px; border-radius: 10px; box-shadow: 0 4px 8px rgba(0, 0, 0, 0.2); } </style> </head> <body> <h1>Grid Layout using flex</h1> <div id="unique-grid"> <div>Item X</div> <div>Item Y</div> <div>Item Z</div> <div>Item W</div> <div>Item V</div> <div>Item U</div> </div> </body> </html>
CSS grid-auto-columns - max-content Value
In the following example, the CSS grid-auto-columns: max-content; allows the grid to dynamically size additional columns based on the maximum content width within each column, ensuring a layout that adjusts according to the content of each grid element.
<html> <head> <style> body { font-family: 'Arial', sans-serif; margin: 0; padding: 0; } #customGrid { height: 350px; display: grid; gap: 25px; background-color: #348570; padding: 25px; grid-auto-columns: max-content; } #customGrid div { background-color: rgba(255, 255, 255, 1); text-align: center; padding: 25px 0; font-size: 30px; border-radius: 12px; box-shadow: 0 4px 8px rgba(0, 0, 0, 0.2); } .boxA { grid-area: 1 / 1 / 2 / 2; } .boxB { grid-area: 1 / 2 / 2 / 3; } .boxC { grid-area: 1 / 3 / 2 / 4; } .boxD { grid-area: 2 / 1 / 3 / 2; } .boxE { grid-area: 2 / 2 / 3 / 3; } .boxF { grid-area: 2 / 3 / 3 / 4; } </style> </head> <body> <div id="customGrid"> <div class="boxA">Grid Element A</div> <div class="boxB">Grid Element B</div> <div class="boxC">Grid Element C</div> <div class="boxD">Grid Element D</div> <div class="boxE">Grid Element E</div> <div class="boxF">Grid Element F</div> </div> </body> </html>
CSS grid-auto-columns - min-content Value
In the following example, the CSS grid-auto-columns: min-content; allows the grid to dynamically size columns based on the minimum content width within each column, ensuring a layout that adjusts according to the content of each grid element.
<html> <head> <style> body { font-family: 'Courier New', monospace; margin: 0; padding: 0; background-color: #2C3E50; display: flex; align-items: center; justify-content: center; height: 100vh; } #uniqueGrid { display: grid; gap: 20px; background-color: #3498db; padding: 25px; grid-auto-columns: min-content; border-radius: 10px; box-shadow: 0 6px 12px rgba(0, 0, 0, 0.4); } #uniqueGrid div { background-color: #ECF0F1; text-align: center; padding: 20px; font-size: 24px; border-radius: 8px; } .uniqueBox1 { grid-area: 1 / 1; } .uniqueBox2 { grid-area: 1 / 2; } .uniqueBox3 { grid-area: 1 / 3; } .uniqueBox4 { grid-area: 2 / 1; } .uniqueBox5 { grid-area: 2 / 2; } .uniqueBox6 { grid-area: 2 / 3; } </style> </head> <body> <div id="uniqueGrid"> <div class="uniqueBox1">Custom Box 1</div> <div class="uniqueBox2">Custom Box 2</div> <div class="uniqueBox3">Custom Box 3</div> <div class="uniqueBox4">Custom Box 4</div> <div class="uniqueBox5">Custom Box 5</div> <div class="uniqueBox6">Custom Box 6</div> </div> </body> </html>
CSS grid-auto-columns - fit-content() Value
The following example demonstrates the usage of grid-auto-columns using fit-content() value.
<html> <head> <style> body { background-color: #F0F2F5; } #custom-grid { display: grid; grid-template-rows: 200px 200px; grid-template-columns: fit-content(100px); grid-auto-columns: fit-content(100px); grid-auto-flow: column; grid-gap: 25px; margin: 20px; } #custom-grid > div { background-color: #3E4095; text-align: center; color: white; font-size: 28px; padding: 25px; } </style> </head> <body> <h1>Custom Grid Layout with fit-content()</h1> <div id="custom-grid"> <div>Element 1</div> <div>Element 2</div> <div>Element 3</div> <div>Element 4</div> <div>Element 5</div> <div>Element 6</div> </div> </body> </html>
CSS grid-auto-columns - auto Value
In the following example, the CSS rule grid-auto-columns: auto; ensures that any additional columns created by the grid-auto-flow: column; property will have an automatic width, allowing the grid to dynamically adjust to the content of each column while maintaining a consistent width.
<html> <head> <style> body { background-color:#E7E9EB; } #grid { display: grid; grid-template-rows: 200px 200px; grid-template-columns: auto; grid-auto-columns: auto; grid-auto-flow: column; grid-gap: 25px; } #grid > div { background-color: #f09f26; text-align: center; color: white; font-size: 30px; padding: 25px; } </style> </head> <body> <h1>Grid layout with grid-auto-column</h1> <div id="grid"> <div>BOX A</div> <div>BOX B</div> <div>BOX C</div> <div>BOX D</div> <div>BOX E</div> <div>BOX F</div> <div>BOX G</div> <div>BOX H</div> </div> </body> </html>