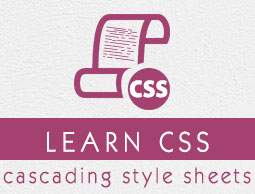
- CSS Tutorial
- CSS - Home
- CSS - Introduction
- CSS - Syntax
- CSS - Selectors
- CSS - Inclusion
- CSS - Measurement Units
- CSS - Colors
- CSS - Backgrounds
- CSS - Fonts
- CSS - Text
- CSS - Images
- CSS - Links
- CSS - Tables
- CSS - Borders
- CSS - Border Block
- CSS - Border Inline
- CSS - Margins
- CSS - Lists
- CSS - Padding
- CSS - Cursor
- CSS - Outlines
- CSS - Dimension
- CSS - Scrollbars
- CSS - Inline Block
- CSS - Dropdowns
- CSS - Visibility
- CSS - Overflow
- CSS - Clearfix
- CSS - Float
- CSS - Arrows
- CSS - Resize
- CSS - Quotes
- CSS - Order
- CSS - Position
- CSS - Hyphens
- CSS - Hover
- CSS - Display
- CSS - Focus
- CSS - Zoom
- CSS - Translate
- CSS - Height
- CSS - Hyphenate Character
- CSS - Width
- CSS - Opacity
- CSS - Z-Index
- CSS - Bottom
- CSS - Navbar
- CSS - Overlay
- CSS - Forms
- CSS - Align
- CSS - Icons
- CSS - Image Gallery
- CSS - Comments
- CSS - Loaders
- CSS - Attr Selectors
- CSS - Combinators
- CSS - Root
- CSS - Box Model
- CSS - Counters
- CSS - Clip
- CSS - Writing Mode
- CSS - Unicode-bidi
- CSS - min-content
- CSS - All
- CSS - Inset
- CSS - Isolation
- CSS - Overscroll
- CSS - Justify Items
- CSS - Justify Self
- CSS - Tab Size
- CSS - Pointer Events
- CSS - Place Content
- CSS - Place Items
- CSS - Place Self
- CSS - Max Block Size
- CSS - Min Block Size
- CSS - Mix Blend Mode
- CSS - Max Inline Size
- CSS - Min Inline Size
- CSS - Offset
- CSS - Accent Color
- CSS - User Select
- CSS Advanced
- CSS - Grid
- CSS - Grid Layout
- CSS - Flexbox
- CSS - Visibility
- CSS - Positioning
- CSS - Layers
- CSS - Pseudo Classes
- CSS - Pseudo Elements
- CSS - @ Rules
- CSS - Text Effects
- CSS - Paged Media
- CSS - Printing
- CSS - Layouts
- CSS - Validations
- CSS - Image Sprites
- CSS - Important
- CSS - Data Types
- CSS3 Tutorial
- CSS3 - Tutorial
- CSS - Rounded Corner
- CSS - Border Images
- CSS - Multi Background
- CSS - Color
- CSS - Gradients
- CSS - Box Shadow
- CSS - Box Decoration Break
- CSS - Caret Color
- CSS - Text Shadow
- CSS - Text
- CSS - 2d transform
- CSS - 3d transform
- CSS - Transition
- CSS - Animation
- CSS - Multi columns
- CSS - Box Sizing
- CSS - Tooltips
- CSS - Buttons
- CSS - Pagination
- CSS - Variables
- CSS - Media Queries
- CSS - Functions
- CSS - Math Functions
- CSS - Masking
- CSS - Shapes
- CSS - Style Images
- CSS - Specificity
- CSS - Custom Properties
- CSS Responsive
- CSS RWD - Introduction
- CSS RWD - Viewport
- CSS RWD - Grid View
- CSS RWD - Media Queries
- CSS RWD - Images
- CSS RWD - Videos
- CSS RWD - Frameworks
- CSS References
- CSS - Questions and Answers
- CSS - Quick Guide
- CSS - References
- CSS - Color References
- CSS - Web browser References
- CSS - Web safe fonts
- CSS - Units
- CSS - Animation
- CSS Resources
- CSS - Useful Resources
- CSS - Discussion
CSS - Media Queries
CSS media queries allow to apply CSS styles to a webpage based on the characteristics of the device or browser viewing the page. With media queries, we can create designs that adapt to different screen sizes, resolutions, and orientations.
Media queries are defined using the @media rule.
Media queries are used for the following:
To apply CSS styles conditionally using CSS @media and @import at-rules.
To target specific media for the <style>, <link>, <source> and other HTML components using media= attribute.
To test media states and monitor them using Window.matchMedia() and EventTarget.addEventListener() methods.
Syntax
@media [media-type] and ([media-feature]) { /* CSS rules to apply when the media query conditions are met */ }
Here, media-type can be things like screen, print, speech, etc., and media-feature can be characteristics such as width, height, aspect ratio, orientation, and more.
Media Types
Media types are used in CSS media queries to apply different styles based on device. The most common media types are all, print, and screen. If you don't specify a media type, it matches all devices. But, if you use the not or only logical operators, you must specify a media type.
all − Default Value. This value applies to all media types.
print − This value is only valid when printing the document.
screen − This value is only valid for screens, such as computers, tablets, and smartphones.
JavaScript provides a CSS object model interface called CSSMediaRule, which can be used to access the rules created using the @media CSS at-rule.
Logical Operators
Media queries can be combined using logical operators such as listed in the below table:
Logical Operators | Explanation |
---|---|
and | It combines multiple media features together into a single media query, where each connected feature must be true for the entire query to be true. |
comma | It combines two or more media queries into a single rule. If any of the media queries in a comma-separated list is true, the entire media statement will also be true. |
or | This is similar to the comma , operator. |
not | It can be used to reverse the logic of a condition. The not operator is true only when all media features are false. |
only | Applies a style only if the entire media query matches. This can be helpful to prevent older browsers from applying styles. |
You can't use the not keyword to negate a single condition in a media query, it can only be applied to an entire media query.
CSS Media Type - All
The following example demonstrates the use of a CSS media query with the media type all −
<html> <head> <style> @media all { h3 { color: red; } p { background-color: yellow; color: blue; } } </style> </head> <body> <h3>Tutorialspoint</h3> <p>CSS Media Type - All</p> </body> </html>
CSS Media Type - Print
Following example demonstrates use of a CSS media query with the media type print. When webpage viewed on a screen the text color will be red. When you print it, the text will be blue.
<html> <head> <style> h3,p { color: red; } @media print { h3,p { color: blue; } } button { background-color: violet; padding: 5px; } </style> </head> <body> <h3>Tutorialspoint</h3> <p>CSS Media Type - Print</p> <p>Click on below button to see the effect when you print the page.</p> <button onclick="printPage()">Print Page</button> <script> function printPage() { window.print(); } </script> </body> </html>
CSS Media Type - Screen
The following example demonstrates that if the screen size is greater than 500px, the background color changes to pink and text color changes to blue −
<html> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <style> h2,h4 { background-color: red; } @media screen and (min-width: 500px) { h2,h4 { background-color: pink; color: blue; } } </style> </head> <body> <p>Resize the browser window to see the effect.</p> <h2>Tutorialspoint</h2> <h4>CSS Media Type - Screen</h4> </body> </html>
CSS Media Type - With Comma
The following example demonstrates using media types with comma separation. The background color changes to yellow and text color changes to blue when in print mode and when screen size is > 500px −
<html> <head> <style> body { color: red } @media screen and (min-width: 500px), print { body { background-color: yellow; color: blue; } } button { background-color: violet; padding: 5px; } </style> </head> <body> <h1>CSS Media Type - Screen and Print</h1> <p>Resize the window to less than 500px to see the background and font color changes to default.</p> <p>Click on below button to see the effect when you print the page.</p> <button onclick="printPage()">Print Page</button> <script> function printPage() { window.print(); } </script> </body> </html>
CSS Media Type - With Only
The following example demonstrates that when the screen width is between 500px and 700px, the background color changes to pink and text color changes to blue −
<html> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <style> body { color: red; } @media only screen and (min-width: 500px) and (max-width: 700px) { body { color: blue; background-color: pink; } } </style> </head> <body> <h1>Tutorialspoint</h1> <p>CSS Media Type - Screen</p> <p>Resize the browser window to see the effect.</p> </body> </html>
CSS Media Queries - Range
The short and easy new range syntax for media query for testing various features that involve a range.
@media (width > 700px) { /* Styles for screens that are at least 700px wide */ }
The following example demonstrates that when the screen width is between 600px and 800px, the background color changes to yellow and text color changes to red −
<html> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <style> p { color: blue; } @media (600px < width < 800px) { p { background-color: yellow; color: red; } } </style> </head> <body> <h1>Tutorialspoint</h1> <p>CSS Media Type - Screen</p> <p>Resize the browser size to see the effect.</p> </body> </html>
Media Features
CSS media feature is used to apply different styles to the webpage based on the a specific characteristic, output device, or environment.
Following is the list of CSS properties related to media features:
Media Features | Description |
---|---|
any-hover | To check if any of the user's input devices can hover over elements on the page. |
any-pointer | To determine if the user has a pointing device such as mouse and whether that device is accurate or not. |
aspect-ratio | To check the aspect ratio of the viewport or the rendering surface. |
color | To specify the desired number of bits per color component of the output device. |
color-gamut | To apply different styles to your web page depending on the range of colors that the user's device can display. |
color-index | To check how many colors a device can display. |
device-aspect-ratio | To check the aspect ratio between the width and height of an output device.This media feature is obsolete and rarely used. Instead, use the aspect-ratio media feature. |
device-height | To check height of the output device's display area.This media feature is obsolete and rarely used. Instead, use the height media feature. |
device-width | To check width of the output device's display area. This media feature is obsolete and rarely used. Instead, use the width media feature. |
display-mode | To check the display mode of a web-application. |
dynamic-range | To check whether the user agent and output device supports the high brightness, contrast ratio, and color depth. |
forced-colors | To check the user has enabled a forced colors mode on their device. |
grid | To check if the user device or screen supports a grid layout. |
height | To determine the height of the viewport. |
hover | To determine if the user's device is able to hover over elements. |
monochrome | To determine the number of bits are used to represent each pixel in the monochrome frame buffer of the output device. |
orientation | To check whether the device's screen or page is in a portrait or landscape orientation. |
overflow-block | To determine how the user device handles content that goes beyond the initial container's boundaries in a vertical direction. |
overflow-inline | To determine how the user device handles content that goes beyond the initial container's boundaries in a horizontal direction. |
pointer | To check if the user has a pointing device they can use to point and click, such as a mouse or touchscreen. |
prefers-color-scheme | To determine whether a user prefers a website to have a light or dark mode. |
prefers-contrast | To check if the user wants the website to have more or less contrast. |
prefers-reduced-motion | To check if a user has enabled a setting on their device to reduce unnecessary moving animations. |
resolution | To check how many pixels are displayed per inch on a screen. |
width | To determine the width of the viewport. |
update | To check how the content look on the screen after when the user device can change the appearance of content |
Creating Complex Media Query
You can create complex media queries by combining multiple conditions using the and, not, and only operators. You can also combine multiple media queries into a comma-separated list.
The and operator is used to group multiple conditions together. The not operator negates a condition. The only operator is used to prevent older browsers from applying the styles.
If you don't mention a media type, all media type is used by default. However, when you use the not or only operators, you need to specify a media type.
CSS Media Queries - Combining Multiple Types or Features
The and keyword joins a media feature with either a media type or other media features to create more specific media queries.
The following example demonstrates that when the screen width is greater tahn 500px and the device is in landscape orientation, the text color changes to blue −
<html> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <style> body { color: red; } @media (min-width: 500px) and (orientation: landscape) { body { color: blue; } } </style> </head> <body> <h3>Resize the browser window to see the effect.</h3> <p>The text color changed to blue when the viewport width is at least 500px and the device is in landscape orientation.</p> </body> </html>
The following example demonstrates that when the device is a screen media type, the screen width is greater than 600px and the device is in landscape orientation, the background color is changes to yellow −
<html> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <style> body { background-color: red; } @media screen and (min-width: 600px) and (orientation: landscape) { body { background-color: yellow; } } </style> </head> <body> <h3>Resize the browser window to see the effect.</h3> <p>The background color of the body element will change to yellow only for screen media type devices that have at least 600px wide and in landscape orientation.</p> </body> </html>
CSS Media Queries - Testing For Multiple Queries
The following example demonstrates that when the viewport height is greater than 700px and the device is a screen with portrait orientation, the text color changes to red −
<html> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <style> body { color: green; } @media (min-height: 700px), screen and (orientation: portrait) { body { color: red; } } </style> </head> <body> <h3>Resize the browser window to see the effect.</h3> <h1>Testing For Multiple Queries</h1> </body> </html>
CSS Media Queries - Inverting a Query's Meaning
CSS not keyword reverse the logic of a media query, so it only applies when the media query conditions are not met. It can only be used to negate entire media queries, not individual feature queries.
The following example demonstrates that the media query@media not all and (monochrome) reverses its meaning. If the device does not support a monochrome (black and white) display, the text color changes to blue otherwise the default text color is black.
<html> <head> <style> h1 { color: black; } @media not all and (monochrome) { h1 { color: blue; } } </style> </head> <body> <h1>Inverting a Query's Meaning</h1> </body> </html>
The not keyword is evaluated last in a media query, so the above media query would be evaluated as follows:
@media not (all and (monochrome)) { /* … */ }
It's not evaluated like this:
@media (not all) and (monochrome) { /* … */ }
CSS Media Queries - Improving Compatibility With Older Browsers
CSS media queries only keyword is used to make sure that older browsers that do not support media queries with media features to ignore the given styles. It doesn't impact modern browsers:
@media only screen and (color) { /* … */ }