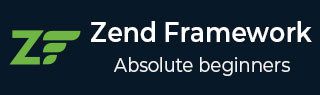
- Zend Framework Tutorial
- Zend Framework - Home
- Zend Framework - Introduction
- Zend Framework - Installation
- Skeleton Application
- Zend Framework - MVC Architecture
- Zend Framework - Concepts
- Zend Framework - Service Manager
- Zend Framework - Event Manager
- Zend Framework - Module System
- Application Structure
- Zend Framework - Creating Module
- Zend Framework - Controllers
- Zend Framework - Routing
- Zend Framework - View Layer
- Zend Framework - Layout
- Models & Database
- Different Databases
- Forms & Validation
- Zend Framework - File Uploading
- Zend Framework - Ajax
- Cookie Management
- Session Management
- Zend Framework - Authentication
- Email Management
- Zend Framework - Unit Testing
- Zend Framework - Error Handling
- Zend Framework - Working Example
- Zend Framework Useful Resources
- Zend Framework - Quick Guide
- Zend Framework - Useful Resources
- Zend Framework - Discussion
Zend Framework - Email Management
The Zend Framework provides a separate component called as zend-mail to send email messages. The zend-mail component also provides an option to read and write email messages with attachments both in text and html format. Sending an email in Zend is much easier and simple to configure.
Let us go through the email concepts, basic settings, advanced settings such as SMTP transport, etc., in this chapter.
Install Mail Component
The mail component can be installed using the following Composer command.
composer require zendframework/zend-mail
Basic Email Configuration
A basic email consists of one or more recipients, a subject, a body and a sender. Zend provides Zend\Mail\Message class to create a new email message. To send an email using the zend-mail, you must specify at least one recipient as well as a message body.
The partial code to create a new mail message is as follows −
use Zend\Mail; $mail = new Mail\Message(); $mail->setSubject('Zend email sample'); $mail->setBody('This is content of the mail message'); $mail->setFrom('sender@example.com', "sender-name"); $mail->addTo('recipient@test.com', "recipient-name");
Zend provides Zend\Mail\Sendmail class to send the mail message. Sendmail uses the php native mail function, mail to send the mail message and we can configure the transport layer using php configuration file.
The partial coding using Sendmail is as follow −
$transport = new Mail\Transport\Sendmail(); $transport->send($mail);
The zend-mail provides many transport layer and each may require many additional parameters such as username, password, etc
Email Management Methods
Some of the notable email management methods are as follows −
isValid − Messages without a ‘From’ address is invalid.
isValid() : bool
setEncoding − Set the message encoding.
setEncoding(string $encoding) : void
getEncoding − Get the message encoding.
getEncoding() : string
setHeaders − Compose headers.
setHeaders(Zend\Mail\Headers $headers) : void
getHeaders − Access headers collection.
getHeaders() : Zend\Mail\Headers
setFrom − Set (overwrite) From addresses. It contains a key/value pairs where the key is the human readable name and the value is the email address.
setFrom( string|AddressInterface|array|AddressList|Traversable $emailOrAddressList, string|null $name ) : void
addFrom − Add a ‘From’ address.
addFrom( string|AddressInterface|array|AddressList|Traversable $emailOrAddressOrList, string|null $name ) : void
getFrom − Retrieve list of ‘From’ senders.
getFrom() : AddressList setTo - Overwrite the address list in the To recipients. setTo( string|AddressInterface|array|AddressList|Traversable $emailOrAddressList, null|string $name ) : void
setSubject − Set the message subject header value.
setSubject(string $subject) :void
setBody − Set the message body.
setBody(null|string|Zend\Mime\Message|object $body) : void
SMTP Transport Layer
The zend-mail provides options to send an email using the SMTP server through the Zend\Mail\Transport\Smtpclass. It is like Sendmail except that it has a few additional options to configure the SMTP host, port, username, password, etc.
The partial code is as follows −
use Zend\Mail\Transport\Smtp as SmtpTransport; use Zend\Mail\Transport\SmtpOptions; $transport = new SmtpTransport(); $options = new SmtpOptions([ 'name' => 'localhost', 'host' =>'smtp.gmail.com', 'port' => 465, ]); $transport->setOptions($options);
Here,
name − Name of the SMTP host.
host − Remote hostname or IP address.
port − Port on which the remote host is listening.
Mail Concept – Example
Let us follow the following points to write a simple php console application to understand the mail concept.
Create a folder “mailapp”.
Install zend-mail using the composer tool.
Create a php file Mail.php inside the “mailapp” folder.
Create the message using the Zend\Mail\Message.
$message = new Message(); $message->addTo('user1@gmail.com'); $message->addFrom('user2@gmail.com'); $message->setSubject('Hello!'); $message->setBody("My first Zend-mail application!");
Create the SMTP transport layer and add the necessary configuration.
// Setup SMTP transport using LOGIN authentication $transport = new SmtpTransport(); $options = new SmtpOptions([ 'name' => 'localhost', 'host' => 'smtp.gmail.com', // or any SMTP server 'port' => 465, // port on which the SMTP server is listening 'connection_class' => 'login', 'connection_config' => [ username' => '<your username>', 'password' => '<your password>', 'ssl' => 'ssl'], ]); $transport->setOptions($options);
Send the email using the send method.
$transport->send($message);
The complete listing, Mail.php is as follows −
<?php require __DIR__ . '/vendor/autoload.php'; use Zend\Mail\Message; use Zend\Mail\Transport\Smtp as SmtpTransport; use Zend\Mail\Transport\SmtpOptions; $message = new Message(); $message->addTo('user1@gmail.com'); $message->addFrom('user2@gmail.com'); $message->setSubject('Hello!'); $message->setBody("My first Zend-mail application!"); // Setup SMTP transport using LOGIN authentication $transport = new SmtpTransport(); $options = new SmtpOptions([ 'name' => 'localhost', 'host' => 'smtp.gmail.com', // or any SMTP server 'port' => 465, // port on which the SMTP server is listening 'connection_class' => 'login', 'connection_config' => [ 'username' => '<your username>', 'password' => '<your password>', 'ssl' => 'ssl'], ]); $transport->setOptions($options); $transport->send($message);
Now, run the application in the command prompt php Mail.php. This will send the mail as configured in the application.
To Continue Learning Please Login