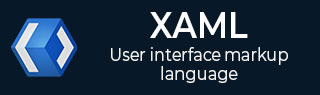
- XAML Tutorial
- XAML - Home
- XAML - Overview
- XAML - Environment Setup
- Writing XAML Aplication On MAC OS
- XAML Vs C# Code
- XAML Vs.VB.NET
- XAML - Building Blocks
- XAML - Controls
- XAML - Layouts
- XAML - Event Handling
- XAML - Data Binding
- XAML - Markup Extensions
- XAML - Dependency Properties
- XAML - Resources
- XAML - Templates
- XAML - Styles
- XAML - Triggers
- XAML - Debugging
- XAML - Custom Controls
- XAML Useful Resources
- XAML - Quick Guide
- XAML - Useful Resources
- XAML - Discussion
XAML - TimePicker
A TimePicker is a control that allows a user to pick a time value. The hierarchical inheritance of TimePicker class is as follows −
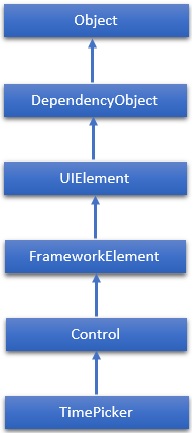
Properties
Sr.No. | Property & Description |
---|---|
1 | ClockIdentifier Gets or sets the clock system to use. |
2 | ClockIdentifierProperty Gets the identifier for the ClockIdentifier dependency property. |
3 | Header Gets or sets the content for the control's header. |
4 | HeaderProperty Identifies the Header dependency property. |
5 | HeaderTemplate Gets or sets the DataTemplate used to display the content of the control's header. |
6 | HeaderTemplateProperty Identifies the HeaderTemplate dependency property. |
7 | MinuteIncrement Gets or sets a value that indicates the time increments shown in the minute picker. For example, 15 specifies that the TimePicker minute control displays only the choices 00, 15, 30, 45. |
8 | MinuteIncrementProperty Gets the identifier for the MinuteIncrement dependency property. |
9 | Time Gets or sets the time currently set in the time picker. |
10 | TimeProperty Gets the identifier for the Time dependency property. |
Events
Sr.No. | Event & Description |
---|---|
1 | ManipulationCompleted Occurs when a manipulation on the UIElement is complete. (Inherited from UIElement) |
2 | ManipulationDelta Occurs when the input device changes position during a manipulation. (Inherited from UIElement) |
3 | ManipulationInertiaStarting Occurs when the input device loses contact with the UIElement object during a manipulation and inertia begins. (Inherited from UIElement) |
4 | ManipulationStarted Occurs when an input device begins a manipulation on the UIElement. (Inherited from UIElement) |
5 | ManipulationStarting Occurs when the manipulation processor is first created. (Inherited from UIElement) |
6 | TimeChanged Occurs when the time value is changed. |
Methods
Sr.No. | Method & Description |
---|---|
1 | OnManipulationCompleted Called before the ManipulationCompleted event occurs. (Inherited from Control) |
2 | OnManipulationDelta Called before the ManipulationDelta event occurs. (Inherited from Control) |
3 | OnManipulationInertiaStarting Called before the ManipulationInertiaStarting event occurs. (Inherited from Control) |
4 | OnManipulationStarted Called before the ManipulationStarted event occurs. (Inherited from Control) |
5 | OnManipulationStarting Called before the ManipulationStarting event occurs. (Inherited from Control) |
Example
The following example shows the usage of TimePicker in an XAML application. Here is the XAML code to create and initialize a TimePicker with some properties.
<Page x:Class = "XAMLTimePicker.MainPage" xmlns = "http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x = "http://schemas.microsoft.com/winfx/2006/xaml" xmlns:local = "using:XAMLTimePicker" xmlns:d = "http://schemas.microsoft.com/expression/blend/2008" xmlns:mc = "http://schemas.openxmlformats.org/markup-compatibility/2006" mc:Ignorable = "d"> <Grid Background = "{ThemeResource ApplicationPageBackgroundThemeBrush}"> <StackPanel Orientation = "Horizontal" Height = "60" Margin = "46,67,-46,641"> <TimePicker x:Name = "arrivalTimePicker" Header = "Arrival Time" Margin = "0,1"/> <Button Content = "Submit" Click = "SubmitButton_Click" Margin = "5,0,0,-2" VerticalAlignment = "Bottom"/> <TextBlock x:Name = "Control1Output" FontSize = "24"/> </StackPanel> </Grid> </Page>
Here is the click event implementation in C# −
using System; using Windows.UI.Xaml; using Windows.UI.Xaml.Controls; namespace XAMLTimePicker { public sealed partial class MainPage : Page { public MainPage() { this.InitializeComponent(); } private void SubmitButton_Click(object sender, RoutedEventArgs e) { if (VerifyTimeIsAvailable(arrivalTimePicker.Time) == true) { Control1Output.Text = string.Format("Thank you. Your appointment is set for {0}.", arrivalTimePicker.Time.ToString()); } else { Control1Output.Text = "Sorry, we're only open from 8AM to 5PM."; } } private bool VerifyTimeIsAvailable(TimeSpan time) { // Set open (8AM) and close (5PM) times. TimeSpan openTime = new TimeSpan(8, 0, 0); TimeSpan closeTime = new TimeSpan(17, 0, 0); if (time >= openTime && time < closeTime) { return true; // Open } return false; // Closed } } }
When you compile and execute the above code, it will display the following output. When time is selected between 8 am to 5 pm, it will display the following message −
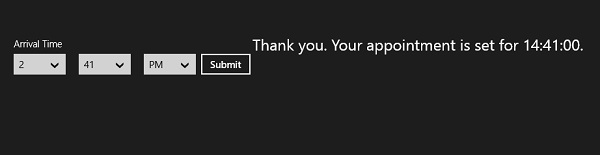
Otherwise, the following message will be displayed −
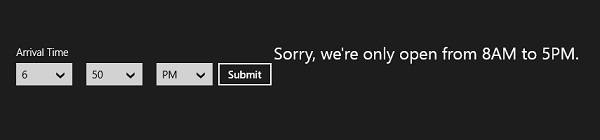
We recommend you to execute the above example code and experiment with some other properties and events.
To Continue Learning Please Login