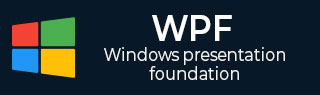
- WPF Tutorial
- WPF - Home
- WPF - Overview
- WPF - Environment Setup
- WPF - Hello World
- WPF - XAML Overview
- WPF - Elements Tree
- WPF - Dependency Properties
- WPF - Routed Events
- WPF - Controls
- WPF - Layouts
- WPF - Nesting Of Layout
- WPF - Input
- WPF - Command Line
- WPF - Data Binding
- WPF - Resources
- WPF - Templates
- WPF - Styles
- WPF - Triggers
- WPF - Debugging
- WPF - Custom Controls
- WPF - Exception Handling
- WPF - Localization
- WPF - Interaction
- WPF - 2D Graphics
- WPF - 3D Graphics
- WPF - Multimedia
- WPF Useful Resources
- WPF - Quick Guide
- WPF - Useful Resources
- WPF - Discussion
WPF - Window
Window is the root window of XAML applications which provides minimize/maximize option, title bar, border, and close button. It also provides the ability to create, configure, show, and manage the lifetime of windows and dialog boxes. The hierarchical inheritance of Window class is as follows −

Commonly Used Properties of Window Class
Sr. No. | Property & Description |
---|---|
1 | AllowsTransparency Gets or sets a value that indicates whether a window's client area supports transparency. |
2 | DialogResult Gets or sets the dialog result value, which is the value that is returned from the ShowDialog method. |
3 | Icon Gets or sets a window's icon. |
4 | IsActive Gets a value that indicates whether the window is active. |
5 | Left Gets or sets the position of the window's left edge, in relation to the desktop. |
6 | OwnedWindows Gets a collection of windows for which this window is the owner. |
7 | Owner Gets or sets the Window that owns this Window. |
8 | ResizeMode Gets or sets the resize mode. |
9 | RestoreBounds Gets the size and location of a window before being either minimized or maximized. |
10 | ShowActivated Gets or sets a value that indicates whether a window is activated when first shown. |
11 | ShowInTaskbar Gets or sets a value that indicates whether the window has a task bar button. |
12 | SizeToContent Gets or sets a value that indicates whether a window will automatically size itself to fit the size of its content. |
13 | TaskbarItemInfo Gets or sets the Windows 7 taskbar thumbnail for the Window. |
14 | Title Gets or sets a window's title. |
15 | Top Gets or sets the position of the window's top edge, in relation to the desktop. |
16 | Topmost Gets or sets a value that indicates whether a window appears in the topmost z-order. |
17 | WindowStartupLocation Gets or sets the position of the window when first shown. |
18 | WindowState Gets or sets a value that indicates whether a window is restored, minimized, or maximized. |
19 | WindowStyle Gets or sets a window's border style. |
Commonly Used Events of Window Class
Sr. No. | Events & Description |
---|---|
1 | Activated Occurs when a window becomes the foreground window. |
2 | Closed Occurs when the window is about to close. |
3 | Closing Occurs directly after Close is called, and can be handled to cancel window closure. |
4 | ContentRendered Occurs after a window's content has been rendered. |
5 | Deactivated Occurs when a window becomes a background window. |
6 | LocationChanged Occurs when the window's location changes. |
7 | SourceInitialized This event is raised to support interoperation with Win32. See HwndSource. |
8 | StateChanged Occurs when the window's WindowState property changes. |
Commonly Used Methods of Window Class
Sr. No. | Methods & Description |
---|---|
1 | Activate Attempts to bring the window to the foreground and activates it. |
2 | Close Manually closes a Window. |
3 | DragMove Allows a window to be dragged by a mouse with its left button down over an exposed area of the window's client area. |
4 | GetWindow Returns a reference to the Window object that hosts the content tree within which the dependency object is located. |
5 | Hide Makes a window invisible. |
6 | Show Opens a window and returns without waiting for the newly opened window to close. |
7 | ShowDialog Opens a window and returns only when the newly opened window is closed. |
Example
When you create a new WPF project, then by default, the Window control is present. Let’s have a look at the following example.
The following XAML code starts with a <Window> Tag and ends with a </Window> tag. The code sets some properties for the window and creates some other controls like text blocks, button, etc.
<Window x:Class = "WPFToolTipControl.MainWindow" xmlns = "http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x = "http://schemas.microsoft.com/winfx/2006/xaml" xmlns:d = "http://schemas.microsoft.com/expression/blend/2008" xmlns:mc = "http://schemas.openxmlformats.org/markup-compatibility/2006" xmlns:local = "clr-namespace:WPFToolTipControl" mc:Ignorable = "d" Title = "MainWindow" Height = "350" Width = "604"> <Grid> <TextBlock x:Name = "textBlock" HorizontalAlignment = "Left" Margin = "101,75,0,0" TextWrapping = "Wrap" Text = "User Name" VerticalAlignment = "Top" /> <TextBlock x:Name = "textBlock1" HorizontalAlignment = "Left" Margin = "101,125,0,0" TextWrapping = "Wrap" Text = "Password" VerticalAlignment = "Top" /> <TextBox x:Name = "textBox" HorizontalAlignment = "Left" Height = "24" Margin = "199,75,0,0" TextWrapping = "Wrap" VerticalAlignment = "Top" Width = "219" ToolTipService.ToolTip = "Enter User Name" /> <PasswordBox x:Name = "passwordBox" HorizontalAlignment = "Left" Margin = "199,125,0,0" VerticalAlignment = "Top" Width = "219" Height = "24" ToolTipService.ToolTip = "Enter Password" /> <Button x:Name = "button" Content = "Log in" HorizontalAlignment = "Left" Margin = "199,189,0,0" VerticalAlignment = "Top" Width = "75" ToolTipService.ToolTip = "Log in" /> </Grid> </Window>
When you compile and execute the above code, it will display the following output. When the mouse enters the region of the Button or the Textboxes, it will show a tooltip.

We recommend that you execute the above example code and try some other properties and events of this class.