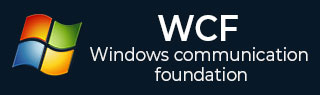
- WCF Tutorial
- WCF - Home
- WCF - Overview
- WCF - Versus Web Service
- WCF - Developers Tools
- WCF - Architecture
- WCF - Creating WCF Service
- WCF - Hosting WCF Service
- WCS - IIS Hosting
- WCF - Self-Hosting
- WCF - WAS Hosting
- WCF - Windows Service Hosting
- WCF - Consuming WCF Service
- WCF - Service Binding
- WCF - Instance Management
- WCF - Transactions
- WCF - RIA Services
- WCF - Security
- WCF - Exception Handling
- WCF Resources
- WCF - Quick Guide
- WCF - Useful Resources
- WCF - Discussion
WCF - Exception Handling
A WCF service developer may encounter some unforeseen errors which require reporting to the client in a suitable manner. Such errors, known as exceptions, are normally handled by using try/catch blocks, but again, this is very technology specific.
Since a client's concern area is not about how an error occurred or the factors contributing to an error, SOAP Fault contract is used to communicate the error message from the service to the client in WCF.
A Fault contract enables the client to have a documented view of the errors occurred in a service. The following example gives a better understanding.
Step 1 − An easy calculator service is created with divide operation which will generate general exceptions.
using System; usingSystem.Collections.Generic; usingSystem.Linq; usingSystem.Runtime.Serialization; usingSystem.ServiceModel; usingSystem.Text; namespace Calculator { // NOTE: You can use the "Rename" command on the "Refactor" menu to change // the interface name "IService1" in both code and config file together. [ServiceContract] public interface IService1 { [OperationContract] int divide(int num1, int num2); // TODO: Add your service operations here } }
The coding for the class file is show below −
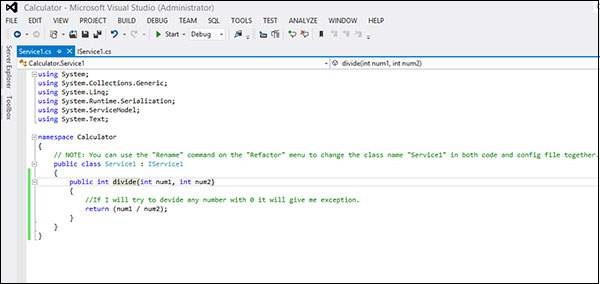
Now, when we try to divide the number 10 by zero, the calculator service will throw an exception.
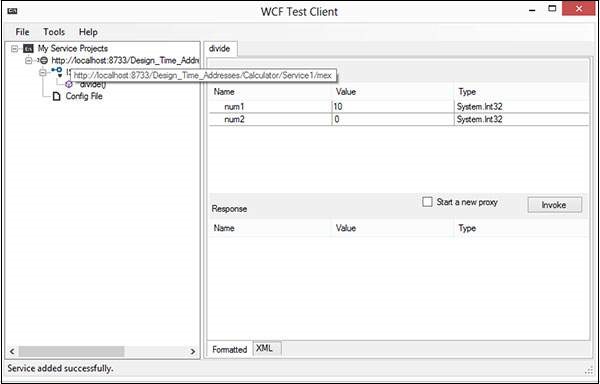
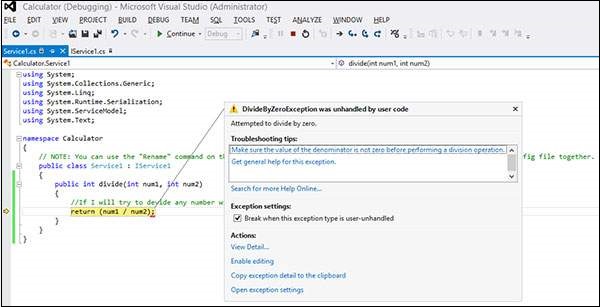
The exception can be handled by try/catch block.
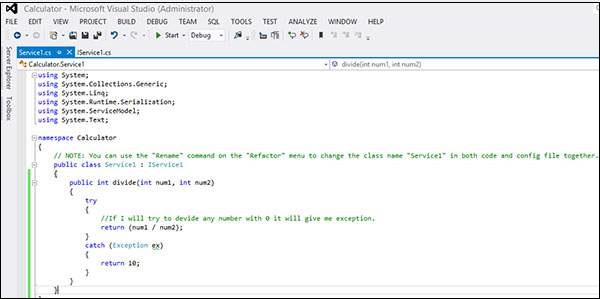
Now, when we try to divide any integer number by 0, it will return the value 10 because we have handled it in the catch block.
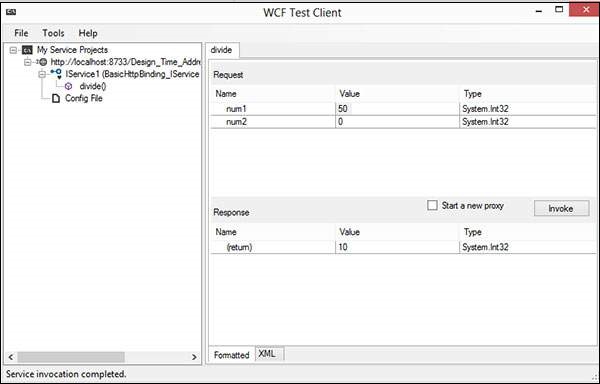
Step 2 − FaultException is used in this step to communicate the exception information to the client from the service.
public int Divide(int num1, int num2) { //Do something throw new FaultException("Error while dividing number"); }
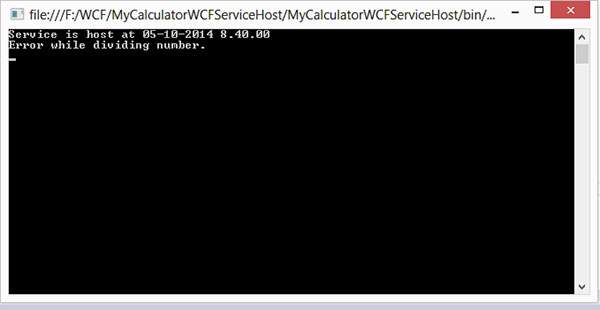
Step 3 − It is also possible to create a custom type to send the error message using FaultContract. The steps essential to create a custom type are mentioned below −
A type is defined by the use of data contract and the fields intended to get returned are specified.
The service operation is decorated by the FaultContract attribute. The type name is also specified.
A service instance is created to raise exceptions and custom exception properties are assigned.