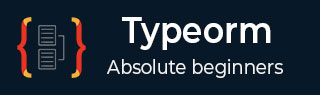
- TypeORM Tutorial
- TypeORM - Home
- TypeORM - Introduction
- TypeORM - Installation
- TypeORM - Creating a Simple Project
- TypeORM - Connection API
- TypeORM - Entity
- TypeORM - Relations
- TypeORM - Working with Repository
- TypeORM - Working with Entity Manager
- TypeORM - Query Builder
- TypeORM - Query Operations
- TypeORM - Transactions
- TypeORM - Indices
- TypeORM - Entity Listener and Logging
- TypeORM with JavaScript
- TypeORM - Working with MongoDB
- TypeORM with Express
- TypeORM - Migrations
- TypeORM - Working with CLI
- TypeORM Useful Resources
- TypeORM - Quick Guide
- TypeORM - Useful Resources
- TypeORM - Discussion
TypeORM - Creating a Simple Project
This chapter explains about how to create simple TypeORM application. Let us create a new directory named ‘TypeORM’ and move into the directory.
cd /path/to/TypeORM/
Syntax
Use below command to create new project −
typeorm init --name <project-name> --database <database-name>
Example
typeorm init --name FirstProject --database mysql
Here,
FirstProject is your project name and sqlite3 is database name. After executing the above command, you could see the following response,
Project created inside /path/to/TypeORM/FirstProject directory
Now, move in to our project directory and install project dependencies using npm module,
$ cd FirstProject $ npm install
Project structure
Let us understand project structure of our newly created project, FirstProject.
FirstProject ├──> src │ ├──> entity │ │ └──> User.ts │ ├──> migration │ └──> index.ts ├──> node_modules ├──> ormconfig.json ├──> package.json ├──> package-lock.json └──> tsconfig.json
Here,
- src − contains source code of your application in TypeScript language. It has a file index.ts and two sub directories entity and migration.
- index.ts − Your entry point to the application.
- entity − Contains database models.
- migration − contains database migration code.
- node_modules − locally saved npm modules.
- ormconfig.json − Main configuration file of your application. It contains database configuration details and entities configuration.
- package.json − Contains node module dependencies.
- package-lock.json − Auto generated file and related to package.json.
- tsconfig.json − Contains TypeScript specific compiler options.
ormconfig.json file
Let us check the configuration option available for our application. Open ormconfig.json file and it looks similar to this −
{ "type": "mysql", "host": "localhost", "port": 3306, "username": "test", "password": "test", "database": "test", "synchronize": true, "logging": false, "entities": [ "src/entity/**/*.ts" ], "migrations": [ "src/migration/**/*.ts" ], "subscribers": [ "src/subscriber/**/*.ts" ], "cli": { "entitiesDir":"src/entity", "migrationsDir":"src/migration", "subscribersDir":"src/subscriber } }
Here,
type, host, username, password, database and port options are related to database setting. mysql can be configured using below configuration −
{ "type": "mysql", "host": "localhost", "port": 3306, "username": "db_username", "password": "db_password", "database": "db_name" }
- entities − refers the location of your entity classes.
- migrations − refers the location of your migration classes.
- subscribers − refers the location of your subscriber classes.
- cli − refers the option used by TypeORM CLI to auto generate the code
Start MySql server
Before starting the application, start your MySQL server or any database server used by you and make sure it is running properly.
Run application
Once everything is configured, we can execute the application using the below command −
npm start
You could see the following response −
> FirstProject@0.0.1 start /Users/../../TypeORM/FirstProject > ts-node src/index.ts Inserting a new user into the database... Saved a new user with id: 1 Loading users from the database... Loaded users: [ User { id: 1, firstName: 'Timber', lastName: 'Saw', age: 25 }] Here you can setup and run express/koa/any other framework.
The application inserted a new user into the database and then reverse load it from the database and finally show the loaded user in the console. We have successfully created a new TypeORM application, configured it and run the application.
We will discuss about how the data is executed elaborately in upcoming chapters.