
- SWING Tutorial
- SWING - Home
- SWING - Overview
- SWING - Environment
- SWING - Controls
- SWING - Event Handling
- SWING - Event Classes
- SWING - Event Listeners
- SWING - Event Adapters
- SWING - Layouts
- SWING - Menu
- SWING - Containers
- SWING Useful Resources
- SWING - Quick Guide
- SWING - Useful Resources
- SWING - Discussion
SWING - WindowListener Interface
The class which processes the WindowEvent should implement this interface. The object of that class must be registered with a component. The object can be registered using the addWindowListener() method.
Interface Declaration
Following is the declaration for java.awt.event.WindowListener interface −
public interface WindowListener extends EventListener
Interface Methods
Sr.No. | Method & Description |
---|---|
1 |
void windowActivated(WindowEvent e) Invoked when the Window is set to be the active Window. |
2 |
void windowClosed(WindowEvent e) Invoked when a window has been closed as a result of calling dispose on the Window. |
3 |
void windowClosing(WindowEvent e) Invoked when the user attempts to close the Window from the Window's system menu. |
4 |
void windowDeactivated(WindowEvent e) Invoked when a Window is no longer the active Window. |
5 |
void windowDeiconified(WindowEvent e) Invoked when a Window is changed from a minimized to a normal state. |
6 |
void windowIconified(WindowEvent e) Invoked when a Window is changed from a normal to a minimized state. |
7 |
void windowOpened(WindowEvent e) Invoked the first time a Window is made visible. |
Methods Inherited
This interface inherits methods from the following interfaces −
java.awt.EventListener
WindowListener Example
Create the following Java program using any editor of your choice in say D:/ > SWING > com > tutorialspoint > gui >
SwingListenerDemo.java
package com.tutorialspoint.gui; import java.awt.*; import java.awt.event.*; import javax.swing.*; public class SwingListenerDemo { private JFrame mainFrame; private JLabel headerLabel; private JLabel statusLabel; private JPanel controlPanel; private JFrame aboutFrame; public SwingListenerDemo(){ prepareGUI(); } public static void main(String[] args){ SwingListenerDemo swingListenerDemo = new SwingListenerDemo(); swingListenerDemo.showWindowListenerDemo(); } private void prepareGUI(){ mainFrame = new JFrame("Java SWING Examples"); mainFrame.setSize(400,400); mainFrame.setLayout(new GridLayout(3, 1)); headerLabel = new JLabel("",JLabel.CENTER ); statusLabel = new JLabel("",JLabel.CENTER); statusLabel.setSize(350,100); mainFrame.addWindowListener(new WindowAdapter() { public void windowClosing(WindowEvent windowEvent){ System.exit(0); } }); controlPanel = new JPanel(); controlPanel.setLayout(new FlowLayout()); mainFrame.add(headerLabel); mainFrame.add(controlPanel); mainFrame.add(statusLabel); mainFrame.setVisible(true); } private void showWindowListenerDemo(){ headerLabel.setText("Listener in action: WindowListener"); JButton okButton = new JButton("OK"); aboutFrame = new JFrame(); aboutFrame.setSize(300,200);; aboutFrame.setTitle("WindowListener Demo"); aboutFrame.addWindowListener(new CustomWindowListener()); JPanel panel = new JPanel(); panel.setBackground(Color.white); JLabel msglabel = new JLabel("Welcome to TutorialsPoint SWING Tutorial." ,JLabel.CENTER); panel.add(msglabel); aboutFrame.add(panel); aboutFrame.setVisible(true); } class CustomWindowListener implements WindowListener { public void windowOpened(WindowEvent e) { } public void windowClosing(WindowEvent e) { aboutFrame.dispose(); } public void windowClosed(WindowEvent e) { } public void windowIconified(WindowEvent e) { } public void windowDeiconified(WindowEvent e) { } public void windowActivated(WindowEvent e) { } public void windowDeactivated(WindowEvent e) { } } }
Compile the program using the command prompt. Go to D:/ > SWING and type the following command.
D:\SWING>javac com\tutorialspoint\gui\SwingListenerDemo.java
If no error occurs, it means the compilation is successful. Run the program using the following command.
D:\SWING>java com.tutorialspoint.gui.SwingListenerDemo
Verify the following output.
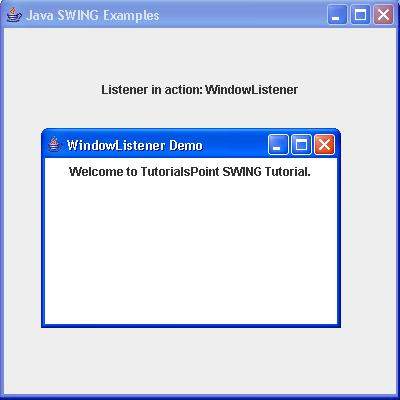