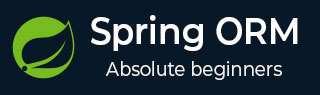
- Spring ORM Tutorial
- Spring ORM - Home
- Spring ORM - Overview
- Spring ORM - Environment Setup
- Spring ORM & Hibernate
- Spring ORM - Create Project
- Spring ORM - Maven Hibernate
- Spring ORM - Persistence Hibernate
- Spring ORM - Update Project
- Spring ORM - Run & Test Hibernate
- Spring ORM & EclipseLink
- Spring ORM - Maven EclipseLink
- Spring ORM - Persistence EclipseLink
- Spring ORM - Update Project EclipseLink
- Spring ORM - Run & Test EclipseLink
- Spring ORM Useful Resources
- Spring ORM - Quick Guide
- Spring ORM - Useful Resources
- Spring ORM - Discussion
Spring ORM - Quick Guide
Spring ORM - Overview
The Spring Framework integrates well with ORM frameworks like Hibernate, Java Persistence API (JPA), Java Data Objects (JDO) and iBATIS SQL Maps. Spring provides resource management, data access object (DAO) implementations, and transaction strategies. Spring allows to configure ORM library features through dependency management. Spring maintains a uniform DAO Exception hiearchies and a generic transaction management for all the ORM libraries it supports.
Spring IoC container facilitates ORM configurations and easy deployment. Following are the key benefits of using Spring framework to create ORM DAO.
Easy to Test − Using spring IoC, an ORM implementation can be easily configured. Each piece of persistence unit can be tested in isolation.
Common Data Access Exception − Spring wraps ORM Tools exceptions to a common runtime exception as DataAccessException. This approach helps to handle most persistence exception (non-recoverable) in appropriate layers. No need to handle ORM specific boilerplate catch/throws/exception declarations.
General Resource Management − Spring application contexts manages persistence objects, their configurations easily. For example, Hibernate SessionFactory instances, JPA EntityManagerFactory instances, JDBC DataSource instances, iBatis SQL Maps configuration objects and other related objects. Spring handles the local as well JTA transaction management by itself.
Integrated transaction management − Spring AOP can be used to wrap an ORM code with a declarative AOP styled interceptor either using @Transaction annotation or by specifying transaction AOP advice in XML configuration file. Spring handles transaction semantics, exception handling, rollback and so on. Spring allows to swap transaction managers without affecting the ORM code.
Spring ORM - Environment Setup
This chapter will guide you on how to prepare a development environment to start your work with Spring Framework. It will also teach you how to set up JDK, Maven and Eclipse on your machine before you set up Spring Framework −
Setup Java Development Kit (JDK)
You can download the latest version of SDK from Oracle's Java site − Java SE Downloads. You will find instructions for installing JDK in downloaded files, follow the given instructions to install and configure the setup. Finally set PATH and JAVA_HOME environment variables to refer to the directory that contains java and javac, typically java_install_dir/bin and java_install_dir respectively.
If you are running Windows and have installed the JDK in C:\jdk-11.0.11, you would have to put the following line in your C:\autoexec.bat file.
set PATH=C:\jdk-11.0.11;%PATH% set JAVA_HOME=C:\jdk-11.0.11
Alternatively, on Windows NT/2000/XP, you will have to right-click on My Computer, select Properties → Advanced → Environment Variables. Then, you will have to update the PATH value and click the OK button.
On Unix (Solaris, Linux, etc.), if the SDK is installed in /usr/local/jdk-11.0.11 and you use the C shell, you will have to put the following into your .cshrc file.
setenv PATH /usr/local/jdk-11.0.11/bin:$PATH setenv JAVA_HOME /usr/local/jdk-11.0.11
Alternatively, if you use an Integrated Development Environment (IDE) like Borland JBuilder, Eclipse, IntelliJ IDEA, or Sun ONE Studio, you will have to compile and run a simple program to confirm that the IDE knows where you have installed Java. Otherwise, you will have to carry out a proper setup as given in the document of the IDE.
Setup Eclipse IDE
All the examples in this tutorial have been written using Eclipse IDE. So we would suggest you should have the latest version of Eclipse installed on your machine.
To install Eclipse IDE, download the latest Eclipse binaries from www.eclipse.org/downloads/. Once you download the installation, unpack the binary distribution into a convenient location. For example, in C:\eclipse on Windows, or /usr/local/eclipse on Linux/Unix and finally set PATH variable appropriately.
Eclipse can be started by executing the following commands on Windows machine, or you can simply double-click on eclipse.exe
%C:\eclipse\eclipse.exe
Eclipse can be started by executing the following commands on Unix (Solaris, Linux, etc.) machine −
$/usr/local/eclipse/eclipse
After a successful startup, if everything is fine then it should display the following result −
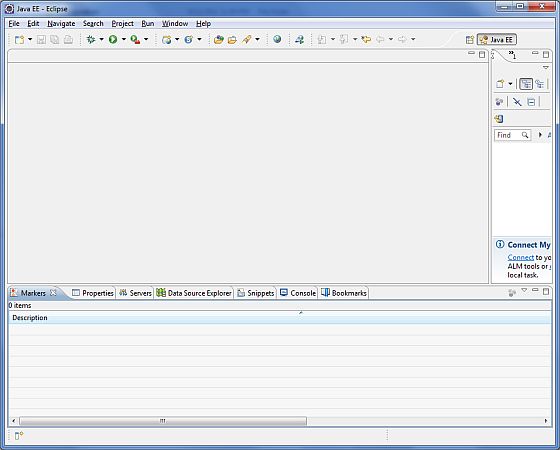
Install MySQL Database
The most important thing you will need, of course is an actual running database with a table that you can query and modify.
MySQL DB − MySQL is an open source database. You can download it from MySQL Official Site. We recommend downloading the full Windows installation.
In addition, download and install MySQL Administrator as well as MySQL Query Browser. These are GUI based tools that will make your development much easier.
Finally, download and unzip MySQL Connector/J (the MySQL JDBC driver) in a convenient directory. For the purpose of this tutorial we will assume that you have installed the driver at C:\Program Files\MySQL\mysql-connector-java-5.1.8.
Accordingly, set CLASSPATH variable to C:\Program Files\MySQL\mysql-connector-java-5.1.8\mysql-connector-java-5.1.8-bin.jar. Your driver version may vary based on your installation.
Set Database Credential
When we install MySQL database, its administrator ID is set to root and it gives provision to set a password of your choice.
Using root ID and password you can either create another user ID and password, or you can use root ID and password for your JDBC application.
There are various database operations like database creation and deletion, which would need administrator ID and password.
If you do not have sufficient privilege to create new users, then you can ask your Database Administrator (DBA) to create a user ID and password for you.
Create Database
To create the TUTORIALSPOINT database, use the following steps −
Step 1
Open a Command Prompt and change to the installation directory as follows −
C:\> C:\>cd Program Files\MySQL\bin C:\Program Files\MySQL\bin>
Note − The path to mysqld.exe may vary depending on the install location of MySQL on your system. You can also check documentation on how to start and stop your database server.
Step 2
Start the database server by executing the following command, if it is already not running.
C:\Program Files\MySQL\bin>mysqld C:\Program Files\MySQL\bin>
Step 3
Create the TUTORIALSPOINT database by executing the following command −
C:\Program Files\MySQL\bin> create database TUTORIALSPOINT;
Note − The path to mysqld.exe may vary depending on the install location of MySQL on your system. You can also check documentation on how to start and stop your database server.
For a complete understanding on MySQL database, study the MySQL Tutorial.
Set Maven
In this tutorial, we are using maven to run and build the spring based examples. Follow the Maven - Environment Setup to install maven.
Spring ORM - Create Project
Using eclipse, select File → New → Maven Project. Tick the Create a simple project(skip archetype selection) and click Next.
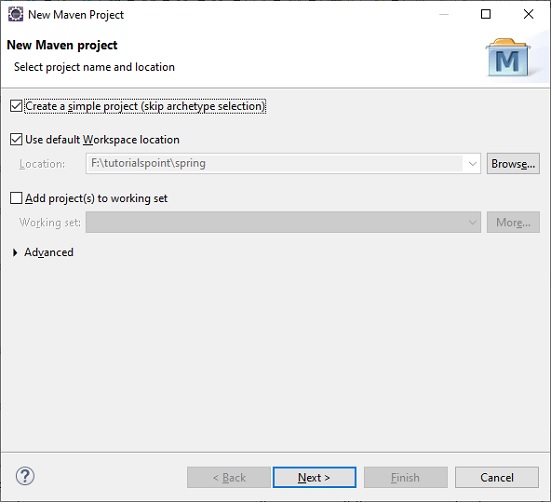
Enter the details, as shown below:
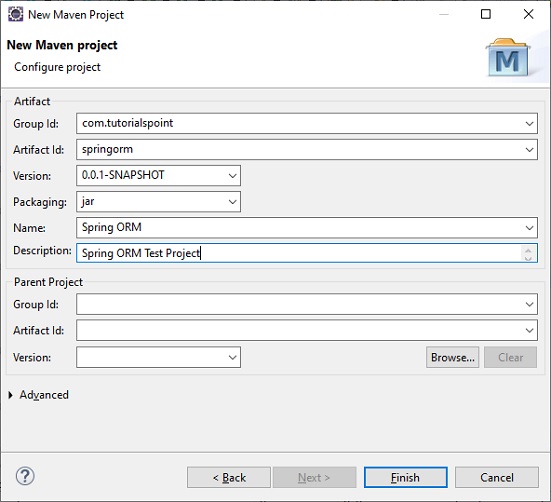
Click on Finish button and an new project will be created.
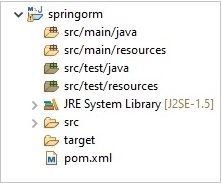
Now as we've our project ready, let add following dependencies in pom.xml in next chapter.
Spring Framework
Hibernate
MySQL Connector
Other related dependencies.
Spring ORM - Maven Hibernate
Let's add following dependencies in pom.xml.
Spring Framework
Hibernate
MySQL Connector
Other related dependencies.
Update the pom.xml as per below content.
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.tutorialspoint</groupId> <artifactId>springorm</artifactId> <version>0.0.1-SNAPSHOT</version> <name>Spring ORM</name> <description>Spring ORM Test Project</description> <properties> <org.springframework.version>4.3.7.RELEASE</org.springframework.version> <org.hibernate.version>5.2.9.Final</org.hibernate.version> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <java.version>1.8</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>${org.springframework.version}</version> <scope>compile</scope> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-orm</artifactId> <version>${org.springframework.version}</version> </dependency> <dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-core</artifactId> <version>${org.hibernate.version}</version> </dependency> <dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-entitymanager</artifactId> <version>${org.hibernate.version}</version> </dependency> <dependency> <groupId>org.apache.commons</groupId> <artifactId>commons-dbcp2</artifactId> <version>2.1.1</version> </dependency> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <scope>runtime</scope> <version>8.0.13</version> </dependency> <dependency> <groupId>com.sun.activation</groupId> <artifactId>javax.activation</artifactId> <version>1.2.0</version> </dependency> <dependency> <groupId>javax.xml.bind</groupId> <artifactId>jaxb-api</artifactId> <version>2.3.1</version> </dependency> <dependency> <groupId>org.glassfish.jaxb</groupId> <artifactId>jaxb-runtime</artifactId> <version>2.3.1</version> <scope>runtime</scope> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.1</version> <configuration> <source>${java.version}</source> <target>${java.version}</target> </configuration> </plugin> </plugins> </build> </project>
Now right click on the project name. Select Maven → Update Project to download the required dependencies.
Spring ORM - Persistence Hibernate
persistence.xml defines the various aspects related to hibernate like following.
Persistence Unit − A persistence unit containing all the details. It's name is used to get reference in spring context.
provider − org.hibernate.jpa.HibernatePersistenceProvider class will be used as provider.
Database url and credentials − In properties section, we pass the database related values.
show_sql − to show the generated sql queries
hbm2ddl − to allow hibernate to create tables.
Create persistence.xml in src -> main > resources > META-INF folder.
persistence.xml
<persistence xmlns="http://xmlns.jcp.org/xml/ns/persistence" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/persistence http://xmlns.jcp.org/xml/ns/persistence/persistence_2_1.xsd" version="2.1"> <persistence-unit name="Hibernate_JPA"> <description> Spring Hibernate JPA Configuration Example</description> <provider>org.hibernate.jpa.HibernatePersistenceProvider</provider> <properties> <property name="javax.persistence.jdbc.driver" value="com.mysql.cj.jdbc.Driver" /> <property name="javax.persistence.jdbc.url" value="jdbc:mysql://localhost:3306/tutorialspoint?useSSL=false" /> <property name="javax.persistence.jdbc.user" value="root" /> <property name="javax.persistence.jdbc.password" value="root@123" /> <property name="hibernate.show_sql" value="true" /> <property name="hibernate.hbm2ddl.auto" value="create" /> </properties> </persistence-unit> </persistence>
Spring ORM - Update Project
Let's add the following classes in the project in the relevant packages.
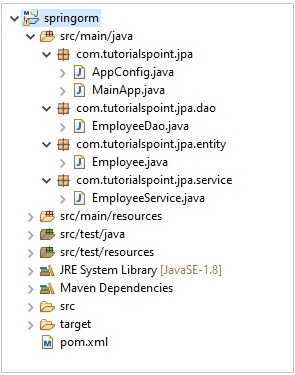
Employee class to be persisted in database.
Employee.java
package com.tutorialspoint.jpa.entity; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; import javax.persistence.Table; @Entity @Table(name = "Employees") public class Employee { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private int id; @Column(name = "NAME") private String name; @Column(name = "SALARY") private double salary; @Column(name = "DESIGNATION") private String designation; public Employee( ) { } public Employee(String name, double salary, String designation) { this.name = name; this.salary = salary; this.designation = designation; } public int getId() { return id; } public void setId(int id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public double getSalary() { return salary; } public void setSalary(double salary) { this.salary = salary; } public String getDesignation() { return designation; } public void setDesignation(String designation) { this.designation = designation; } }
Employee DAO class to do operations on database.
EmployeeDao.java
package com.tutorialspoint.jpa.dao; import java.util.List; import javax.persistence.EntityManager; import javax.persistence.PersistenceContext; import javax.persistence.criteria.CriteriaQuery; import javax.persistence.criteria.Root; import org.springframework.stereotype.Repository; import com.tutorialspoint.jpa.entity.Employee; @Repository public class EmployeeDao { @PersistenceContext private EntityManager em; public void add(Employee employee) { em.persist(employee); } public List<Employee> listEmployees() { CriteriaQuery<Employee> criteriaQuery = em.getCriteriaBuilder().createQuery(Employee.class); @SuppressWarnings("unused") Root<Employee> root = criteriaQuery.from(Employee.class); return em.createQuery(criteriaQuery).getResultList(); } }
Employee Service class to use Employee DAO class.
EmployeeService.java
package com.tutorialspoint.jpa.service; import java.util.List; import javax.transaction.Transactional; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; import com.tutorialspoint.jpa.dao.EmployeeDao; import com.tutorialspoint.jpa.entity.Employee; @Service public class EmployeeService { @Autowired private EmployeeDao employeeDao; @Transactional public void add(Employee employee) { employeeDao.add(employee); } @Transactional() public List<Employee> listEmployees() { return employeeDao.listEmployees(); } }
Application Configuration to be used by Spring framework.
AppConfig.java
package com.tutorialspoint.jpa; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.ComponentScan; import org.springframework.context.annotation.ComponentScans; import org.springframework.context.annotation.Configuration; import org.springframework.orm.jpa.JpaTransactionManager; import org.springframework.orm.jpa.LocalEntityManagerFactoryBean; import org.springframework.transaction.annotation.EnableTransactionManagement; @Configuration @EnableTransactionManagement @ComponentScans(value = { @ComponentScan("com.tutorialspoint.jpa.dao"), @ComponentScan("com.tutorialspoint.jpa.service") }) public class AppConfig { @Bean public LocalEntityManagerFactoryBean geEntityManagerFactoryBean() { LocalEntityManagerFactoryBean factoryBean = new LocalEntityManagerFactoryBean(); factoryBean.setPersistenceUnitName("Hibernate_JPA"); return factoryBean; } @Bean public JpaTransactionManager geJpaTransactionManager() { JpaTransactionManager transactionManager = new JpaTransactionManager(); transactionManager.setEntityManagerFactory(geEntityManagerFactoryBean().getObject()); return transactionManager; } }
Main Application to run and test the functionalities.
MainApp.java
package com.tutorialspoint.jpa; import java.sql.SQLException; import java.util.List; import org.springframework.context.annotation.AnnotationConfigApplicationContext; import com.tutorialspoint.jpa.entity.Employee; import com.tutorialspoint.jpa.service.EmployeeService; public class MainApp { public static void main(String[] args) throws SQLException { AnnotationConfigApplicationContext context = new AnnotationConfigApplicationContext(AppConfig.class); EmployeeService employeeService = context.getBean(EmployeeService.class); employeeService.add(new Employee("Julie", 10000, "Technical Manager")); employeeService.add(new Employee("Robert", 20000, "Senior Manager")); employeeService.add(new Employee("Anil", 5000, "Software Engineer")); // Get Persons List<Employee> employees = employeeService.listEmployees(); for (Employee employee : employees) { System.out.println("Id : "+employee.getId()); System.out.println("Name : "+employee.getName()); System.out.println("Salary = "+employee.getSalary()); System.out.println("Designation = "+employee.getDesignation()); System.out.println(); } context.close(); } }
Spring ORM - Run & Test Hibernate
Now in eclipse, right click on the MainApp.java, select Run As context menu, and select Java Application. Check the console logs in the eclipse. You can see the below logs −
... Sep 27, 2021 9:16:52 AM org.springframework.orm.jpa.LocalEntityManagerFactoryBean createNativeEntityManagerFactory INFO: Building JPA EntityManagerFactory for persistence unit 'Hibernate_JPA' Sep 27, 2021 9:16:52 AM org.hibernate.jpa.internal.util.LogHelper logPersistenceUnitInformation INFO: HHH000204: Processing PersistenceUnitInfo [name: Hibernate_JPA...] ... INFO: HHH000400: Using dialect: org.hibernate.dialect.MySQL5Dialect Hibernate: drop table if exists Employees Sep 27, 2021 9:16:54 AM org.hibernate.resource.transaction.backend.jdbc.internal.DdlTransactionIsolatorNonJtaImpl getIsolatedConnection INFO: HHH10001501: Connection obtained from JdbcConnectionAccess [org.hibernate.engine.jdbc.env.internal.JdbcEnvironmentInitiator$ConnectionProviderJdbcConnectionAccess@2264ea32] for (non-JTA) DDL execution was not in auto-commit mode; the Connection 'local transaction' will be committed and the Connection will be set into auto-commit mode. Hibernate: create table Employees (id integer not null auto_increment, DESIGNATION varchar(255), NAME varchar(255), SALARY double precision, primary key (id)) engine=MyISAM Sep 27, 2021 9:16:54 AM org.hibernate.resource.transaction.backend.jdbc.internal.DdlTransactionIsolatorNonJtaImpl getIsolatedConnection INFO: HHH10001501: Connection obtained from JdbcConnectionAccess [org.hibernate.engine.jdbc.env.internal.JdbcEnvironmentInitiator$ConnectionProviderJdbcConnectionAccess@58740366] for (non-JTA) DDL execution was not in auto-commit mode; the Connection 'local transaction' will be committed and the Connection will be set into auto-commit mode. Sep 27, 2021 9:16:54 AM org.hibernate.tool.schema.internal.SchemaCreatorImpl applyImportSources INFO: HHH000476: Executing import script 'org.hibernate.tool.schema.internal.exec.ScriptSourceInputNonExistentImpl@4b74b35' Sep 27, 2021 9:16:54 AM org.springframework.orm.jpa.LocalEntityManagerFactoryBean buildNativeEntityManagerFactory INFO: Initialized JPA EntityManagerFactory for persistence unit 'Hibernate_JPA' Hibernate: insert into Employees (DESIGNATION, NAME, SALARY) values (?, ?, ?) Hibernate: insert into Employees (DESIGNATION, NAME, SALARY) values (?, ?, ?) Hibernate: insert into Employees (DESIGNATION, NAME, SALARY) values (?, ?, ?) Sep 27, 2021 9:16:55 AM org.hibernate.hql.internal.QueryTranslatorFactoryInitiator initiateService INFO: HHH000397: Using ASTQueryTranslatorFactory Hibernate: select employee0_.id as id1_0_, employee0_.DESIGNATION as DESIGNAT2_0_, employee0_.NAME as NAME3_0_, employee0_.SALARY as SALARY4_0_ from Employees employee0_ Id : 1 Name : Julie Salary = 10000.0 Designation = Technical Manager Id : 2 Name : Robert Salary = 20000.0 Designation = Senior Manager Id : 3 Name : Anil Salary = 5000.0 Designation = Software Engineer Sep 27, 2021 9:16:55 AM org.springframework.context.annotation.AnnotationConfigApplicationContext doClose INFO: Closing org.springframework.context.annotation.AnnotationConfigApplicationContext@18eed359: startup date [Mon Sep 27 09:16:51 IST 2021]; root of context hierarchy Sep 27, 2021 9:16:55 AM org.springframework.orm.jpa.LocalEntityManagerFactoryBean destroy INFO: Closing JPA EntityManagerFactory for persistence unit 'Hibernate_JPA' Sep 27, 2021 9:16:55 AM org.hibernate.engine.jdbc.connections.internal.DriverManagerConnectionProviderImpl stop INFO: HHH10001008: Cleaning up connection pool [jdbc:mysql://localhost:3306/tutorialspoint?useSSL=false]
Here project is built and run using spring configurations. A table Employee is created and have three records. You can verify the same using MySQL console.
Enter password: ******** Welcome to the MySQL monitor. Commands end with ; or \g. Your MySQL connection id is 41 Server version: 8.0.23 MySQL Community Server - GPL Copyright (c) 2000, 2021, Oracle and/or its affiliates. Oracle is a registered trademark of Oracle Corporation and/or its affiliates. Other names may be trademarks of their respective owners. Type 'help;' or '\h' for help. Type '\c' to clear the current input statement. mysql> use tutorialspoint; Database changed mysql> select * from employees; +----+-------------------+--------+--------+ | id | DESIGNATION | NAME | SALARY | +----+-------------------+--------+--------+ | 1 | Technical Manager | Julie | 10000 | | 2 | Senior Manager | Robert | 20000 | | 3 | Software Engineer | Anil | 5000 | +----+-------------------+--------+--------+ 3 rows in set (0.00 sec) mysql>
Spring ORM - Maven EclipseLink
Let's add following dependencies in pom.xml.
Spring Framework
EclipseLink
MySQL Connector
Other related dependencies.
Update the pom.xml as per below content.
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.tutorialspoint</groupId> <artifactId>springorm</artifactId> <version>0.0.1-SNAPSHOT</version> <name>Spring ORM</name> <description>Spring ORM Test Project</description> <properties> <org.springframework.version>4.3.7.RELEASE</org.springframework.version> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <java.version>1.8</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>${org.springframework.version}</version> <scope>compile</scope> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-orm</artifactId> <version>${org.springframework.version}</version> </dependency> <dependency> <groupId>org.eclipse.persistence</groupId> <artifactId>eclipselink</artifactId> <version>2.5.1</version> </dependency> <dependency> <groupId>org.eclipse.persistence</groupId> <artifactId>javax.persistence</artifactId> <version>2.0.0</version> </dependency> <dependency> <groupId>javax.transaction</groupId> <artifactId>javax.transaction-api</artifactId> <version>1.3</version> </dependency> <dependency> <groupId>org.apache.commons</groupId> <artifactId>commons-dbcp2</artifactId> <version>2.1.1</version> </dependency> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <scope>runtime</scope> <version>8.0.13</version> </dependency> <dependency> <groupId>com.sun.activation</groupId> <artifactId>javax.activation</artifactId> <version>1.2.0</version> </dependency> <dependency> <groupId>javax.xml.bind</groupId> <artifactId>jaxb-api</artifactId> <version>2.3.1</version> </dependency> <dependency> <groupId>org.glassfish.jaxb</groupId> <artifactId>jaxb-runtime</artifactId> <version>2.3.1</version> <scope>runtime</scope> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.1</version> <configuration> <source>${java.version}</source> <target>${java.version}</target> </configuration> </plugin> </plugins> </build> </project>
Now right click on the project name. Select Maven → Update Project to download the required dependencies.
Spring ORM - Persistence EclipseLink
persistence.xml defines the various aspects related to eclipselink like following.
Persistence Unit − A persistence unit containing all the details. It's name is used to get reference in spring context.
provider − org.eclipse.persistence.jpa.PersistenceProvider class will be used as provider.
Database url and credentials − In properties section, we pass the database related values.
class − to register the class to be persisted.
eclipselink.ddl-generation − to allow eclipselink to create tables.
Create persistence.xml in src -> main > resources > META-INF folder.
persistence.xml
<persistence xmlns="http://xmlns.jcp.org/xml/ns/persistence" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/persistence http://xmlns.jcp.org/xml/ns/persistence/persistence_2_1.xsd" version="2.1"> <persistence-unit name="EclipseLink_JPA"> <description> Spring EclipseLink JPA Configuration Example</description> <provider>org.eclipse.persistence.jpa.PersistenceProvider</provider> <class>com.tutorialspoint.jpa.entity.Employee</class> <properties> <property name="javax.persistence.jdbc.driver" value="com.mysql.cj.jdbc.Driver" /> <property name="javax.persistence.jdbc.url" value="jdbc:mysql://localhost:3306/tutorialspoint?useSSL=false" /> <property name="javax.persistence.jdbc.user" value="root" /> <property name="javax.persistence.jdbc.password" value="root@123" /> <property name="eclipselink.logging.level" value="FINE"/> <property name="eclipselink.ddl-generation" value="create-tables"/> </properties> </persistence-unit> </persistence>
Spring ORM - Update Project EclipseLink
Now update the AppConfig.java to use the persistence unit created for EclipseLink.
AppConfig.java
package com.tutorialspoint.jpa; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.ComponentScan; import org.springframework.context.annotation.ComponentScans; import org.springframework.context.annotation.Configuration; import org.springframework.orm.jpa.JpaTransactionManager; import org.springframework.orm.jpa.LocalEntityManagerFactoryBean; import org.springframework.transaction.annotation.EnableTransactionManagement; @Configuration @EnableTransactionManagement @ComponentScans(value = { @ComponentScan("com.tutorialspoint.jpa.dao"), @ComponentScan("com.tutorialspoint.jpa.service") }) public class AppConfig { @Bean public LocalEntityManagerFactoryBean geEntityManagerFactoryBean() { LocalEntityManagerFactoryBean factoryBean = new LocalEntityManagerFactoryBean(); factoryBean.setPersistenceUnitName("EclipseLink_JPA"); return factoryBean; } @Bean public JpaTransactionManager geJpaTransactionManager() { JpaTransactionManager transactionManager = new JpaTransactionManager(); transactionManager.setEntityManagerFactory(geEntityManagerFactoryBean().getObject()); return transactionManager; } }
Now reset the database.
mysql> use tutorialspoint Database changed mysql> delete from employees; Query OK, 3 rows affected (0.02 sec) mysql> drop table employees; Query OK, 0 rows affected (0.17 sec) mysql>
Spring ORM - Test EclipseLink
Now in eclipse, right click on the MainApp.java, select Run As context menu, and select Java Application. Check the console logs in the eclipse. You can see the below logs −
... Sep 28, 2021 8:56:13 AM org.springframework.orm.jpa.LocalEntityManagerFactoryBean createNativeEntityManagerFactory INFO: Building JPA EntityManagerFactory for persistence unit 'EclipseLink_JPA' [EL Config]: metadata: 2021-09-28 08:56:13.763--ServerSession(712627377)--Thread(Thread[main,5,main])--The access type for the persistent class [class com.tutorialspoint.jpa.entity.Employee] is set to [FIELD]. [EL Config]: metadata: 2021-09-28 08:56:13.787--ServerSession(712627377)--Thread(Thread[main,5,main])--The alias name for the entity class [class com.tutorialspoint.jpa.entity.Employee] is being defaulted to: Employee. [EL Config]: metadata: 2021-09-28 08:56:13.802--ServerSession(712627377)--Thread(Thread[main,5,main])--The column name for element [id] is being defaulted to: ID. Sep 28, 2021 8:56:13 AM org.springframework.orm.jpa.LocalEntityManagerFactoryBean buildNativeEntityManagerFactory INFO: Initialized JPA EntityManagerFactory for persistence unit 'EclipseLink_JPA' [EL Info]: 2021-09-28 08:56:14.102--ServerSession(712627377)--Thread(Thread[main,5,main])--EclipseLink, version: Eclipse Persistence Services - 2.5.1.v20130918-f2b9fc5 [EL Fine]: connection: 2021-09-28 08:56:14.403--Thread(Thread[main,5,main])--Detected database platform: org.eclipse.persistence.platform.database.MySQLPlatform [EL Config]: connection: 2021-09-28 08:56:14.423--ServerSession(712627377)--Connection(741883443)--Thread(Thread[main,5,main])--connecting(DatabaseLogin( platform=>MySQLPlatform user name=> "root" datasource URL=> "jdbc:mysql://localhost:3306/tutorialspoint?useSSL=false" )) [EL Config]: connection: 2021-09-28 08:56:14.469--ServerSession(712627377)--Connection(2134915053)--Thread(Thread[main,5,main])--Connected: jdbc:mysql://localhost:3306/tutorialspoint?useSSL=false User: root@localhost Database: MySQL Version: 8.0.23 Driver: MySQL Connector/J Version: mysql-connector-java-8.0.13 (Revision: 66459e9d39c8fd09767992bc592acd2053279be6) [EL Info]: connection: 2021-09-28 08:56:14.539--ServerSession(712627377)--Thread(Thread[main,5,main])--file:/F:/Workspace/springorm/target/classes/_EclipseLink_JPA login successful [EL Fine]: sql: 2021-09-28 08:56:14.602--ServerSession(712627377)--Connection(2134915053)--Thread(Thread[main,5,main])--CREATE TABLE Employees (ID INTEGER AUTO_INCREMENT NOT NULL, DESIGNATION VARCHAR(255), NAME VARCHAR(255), SALARY DOUBLE, PRIMARY KEY (ID)) [EL Fine]: sql: 2021-09-28 08:56:14.836--ClientSession(181326224)--Connection(2134915053)--Thread(Thread[main,5,main])--INSERT INTO Employees (DESIGNATION, NAME, SALARY) VALUES (?, ?, ?) bind => [Technical Manager, Julie, 10000.0] [EL Fine]: sql: 2021-09-28 08:56:14.855--ClientSession(181326224)--Connection(2134915053)--Thread(Thread[main,5,main])--SELECT LAST_INSERT_ID() [EL Fine]: sql: 2021-09-28 08:56:14.883--ClientSession(1573125303)--Connection(2134915053)--Thread(Thread[main,5,main])--INSERT INTO Employees (DESIGNATION, NAME, SALARY) VALUES (?, ?, ?) bind => [Senior Manager, Robert, 20000.0] [EL Fine]: sql: 2021-09-28 08:56:14.885--ClientSession(1573125303)--Connection(2134915053)--Thread(Thread[main,5,main])--SELECT LAST_INSERT_ID() [EL Fine]: sql: 2021-09-28 08:56:14.893--ClientSession(2054787417)--Connection(2134915053)--Thread(Thread[main,5,main])--INSERT INTO Employees (DESIGNATION, NAME, SALARY) VALUES (?, ?, ?) bind => [Software Engineer, Anil, 5000.0] [EL Fine]: sql: 2021-09-28 08:56:14.896--ClientSession(2054787417)--Connection(2134915053)--Thread(Thread[main,5,main])--SELECT LAST_INSERT_ID() [EL Fine]: sql: 2021-09-28 08:56:14.929--ServerSession(712627377)--Connection(2134915053)--Thread(Thread[main,5,main])--SELECT ID, DESIGNATION, NAME, SALARY FROM Employees Id : 1 Name : Julie Salary = 10000.0 Designation = Technical Manager Id : 2 Name : Robert Salary = 20000.0 Designation = Senior Manager Id : 3 Name : Anil Salary = 5000.0 Designation = Software Engineer Sep 28, 2021 8:56:14 AM org.springframework.context.annotation.AnnotationConfigApplicationContext doClose INFO: Closing org.springframework.context.annotation.AnnotationConfigApplicationContext@5383967b: startup date [Tue Sep 28 08:56:12 IST 2021]; root of context hierarchy [EL Config]: connection: 2021-09-28 08:56:14.935--ServerSession(712627377)--Connection(2134915053)--Thread(Thread[main,5,main])--disconnect Sep 28, 2021 8:56:14 AM org.springframework.orm.jpa.LocalEntityManagerFactoryBean destroy INFO: Closing JPA EntityManagerFactory for persistence unit 'EclipseLink_JPA' [EL Info]: connection: 2021-09-28 08:56:14.936--ServerSession(712627377)--Thread(Thread[main,5,main])--file:/F:/Workspace/springorm/target/classes/_EclipseLink_JPA logout successful [EL Config]: connection: 2021-09-28 08:56:14.936--ServerSession(712627377)--Connection(741883443)--Thread(Thread[main,5,main])--disconnect
Here project is built and run using spring configurations. A table Employee is created and have three records. You can verify the same using MySQL console.
Enter password: ******** Welcome to the MySQL monitor. Commands end with ; or \g. Your MySQL connection id is 41 Server version: 8.0.23 MySQL Community Server - GPL Copyright (c) 2000, 2021, Oracle and/or its affiliates. Oracle is a registered trademark of Oracle Corporation and/or its affiliates. Other names may be trademarks of their respective owners. Type 'help;' or '\h' for help. Type '\c' to clear the current input statement. mysql> use tutorialspoint; Database changed mysql> select * from employees; +----+-------------------+--------+--------+ | id | DESIGNATION | NAME | SALARY | +----+-------------------+--------+--------+ | 1 | Technical Manager | Julie | 10000 | | 2 | Senior Manager | Robert | 20000 | | 3 | Software Engineer | Anil | 5000 | +----+-------------------+--------+--------+ 3 rows in set (0.00 sec) mysql>
To Continue Learning Please Login