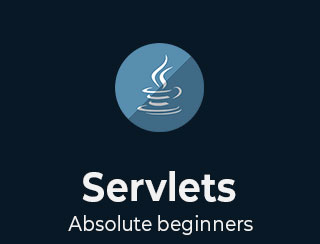
- Servlets Tutorial
- Servlets - Home
- Servlets - Overview
- Servlets - Environment Setup
- Servlets - Life Cycle
- Servlets - Examples
- Servlets - Form Data
- Servlets - Client Request
- Servlets - Server Response
- Servlets - Http Codes
- Servlets - Writing Filters
- Servlets - Exceptions
- Servlets - Cookies Handling
- Servlets - Session Tracking
- Servlets - Database Access
- Servlets - File Uploading
- Servlets - Handling Date
- Servlets - Page Redirect
- Servlets - Hits Counter
- Servlets - Auto Refresh
- Servlets - Sending Email
- Servlets - Packaging
- Servlets - Debugging
- Servlets - Internationalization
- Servlets - Annotations
- Servlets Useful Resources
- Servlets - Questions and Answers
- Servlets - Quick Guide
- Servlets - Useful Resources
- Servlets - Discussion
Servlets - Page Redirection
Page redirection is a technique where the client is sent to a new location other than requested. Page redirection is generally used when a document moves to a new location or may be because of load balancing.
The simplest way of redirecting a request to another page is using method sendRedirect() of response object. Following is the signature of this method −
public void HttpServletResponse.sendRedirect(String location) throws IOException
This method sends back the response to the browser along with the status code and new page location. You can also use setStatus() and setHeader() methods together to achieve the same −
.... String site = "http://www.newpage.com" ; response.setStatus(response.SC_MOVED_TEMPORARILY); response.setHeader("Location", site); ....
Example
This example shows how a servlet performs page redirection to another location −
import java.io.*; import java.sql.Date; import java.util.*; import javax.servlet.*; import javax.servlet.http.*; public class PageRedirect extends HttpServlet { public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // Set response content type response.setContentType("text/html"); // New location to be redirected String site = new String("http://www.photofuntoos.com"); response.setStatus(response.SC_MOVED_TEMPORARILY); response.setHeader("Location", site); } }
Now let us compile above servlet and create following entries in web.xml
.... <servlet> <servlet-name>PageRedirect</servlet-name> <servlet-class>PageRedirect</servlet-class> </servlet> <servlet-mapping> <servlet-name>PageRedirect</servlet-name> <url-pattern>/PageRedirect</url-pattern> </servlet-mapping> ....
Now call this servlet using URL http://localhost:8080/PageRedirect. This would redirect you to URL http://www.photofuntoos.com.