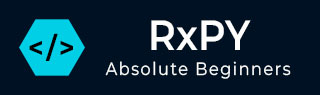
- RxPY Tutorial
- RxPY - Home
- RxPY - Overview
- RxPY - Environment Setup
- RxPY - Latest Release Updates
- RxPY - Working with Observables
- RxPY- Operators
- RxPY - Working With Subject
- RxPY - Concurrency Using Scheduler
- RxPY - Examples
- RxPY Useful Resources
- RxPY - Quick Guide
- RxPY - Useful Resources
- RxPY - Discussion
RxPY - Utility Operators
delay
This operator will delay the source observable emission as per the time or date given.
Syntax
delay(timespan)
Parameters
timespan: this will be the time in seconds or date.
Return value
It will give back an observable with source values emitted after the timeout.
Example
from rx import of, operators as op import datetime test1 = of(1,2,3,4,5) sub1 = test1.pipe( op.delay(5.0) ) sub1.subscribe(lambda x: print("The value is {0}".format(x))) input("Press any key to exit\n")
Output
E:\pyrx>python testrx.py Press any key to exit The value is 1 The value is 2 The value is 3 The value is 4 The value is 5
materialize
This operator will convert the values from the source observable with the values emitted in the form of explicit notification values.
Syntax
materialize()
Return value
This will give back an observable with the values emitted in the form of explicit notification values.
Example
from rx import of, operators as op import datetime test1 = of(1,2,3,4,5) sub1 = test1.pipe( op.materialize() ) sub1.subscribe(lambda x: print("The value is {0}".format(x)))
Output
E:\pyrx>python testrx.py The value is OnNext(1.0) The value is OnNext(2.0) The value is OnNext(3.0) The value is OnNext(4.0) The value is OnNext(5.0) The value is OnCompleted()
time_interval
This operator will give the time elapsed between the values from the source observable.
Syntax
time_interval()
Return value
It will return an observable that will have the time elapsed, between the source value emitted.
Example
from rx import of, operators as op from datetime import date test = of(1,2,3,4,5,6) sub1 = test.pipe( op.time_interval() ) sub1.subscribe(lambda x: print("The value is {0}".format(x)))
Output
E:\pyrx>python testrx.py The value is TimeInterval(value=1, interval=datetime.timedelta(microseconds=1000 )) The value is TimeInterval(value=2, interval=datetime.timedelta(0)) The value is TimeInterval(value=3, interval=datetime.timedelta(0)) The value is TimeInterval(value=4, interval=datetime.timedelta(microseconds=1000 )) The value is TimeInterval(value=5, interval=datetime.timedelta(0)) The value is TimeInterval(value=6, interval=datetime.timedelta(0))
timeout
This operator will give all the values from the source observable, after the elapsed time or else will trigger an error.
Syntax
timeout(duetime)
Parameters
duetime: the time given in seconds.
Return value
It will give back on observable with all values from the source observable.
Example
from rx import of, operators as op from datetime import date test = of(1,2,3,4,5,6) sub1 = test.pipe( op.timeout(5.0) ) sub1.subscribe(lambda x: print("The value is {0}".format(x)))
Output
E:\pyrx>python testrx.py The value is 1 The value is 2 The value is 3 The value is 4 The value is 5 The value is 6
timestamp
This operator will attach a timestamp to all the values from the source observable.
Syntax
timestamp()
Return value
It will give back an observable with all values from the source observable along with a timestamp.
Example
from rx import of, operators as op from datetime import date test = of(1,2,3,4,5,6) sub1 = test.pipe( op.timestamp() ) sub1.subscribe(lambda x: print("The value is {0}".format(x)))
Output
E:\pyrx>python testrx.py The value is Timestamp(value=1, timestamp=datetime.datetime(2019, 11, 4, 4, 57, 44, 667243)) The value is Timestamp(value=2, timestamp=datetime.datetime(2019, 11, 4, 4, 57, 44, 668243)) The value is Timestamp(value=3, timestamp=datetime.datetime(2019, 11, 4, 4, 57, 44, 668243)) The value is Timestamp(value=4, timestamp=datetime.datetime(2019, 11, 4, 4, 57, 44, 668243)) The value is Timestamp(value=5, timestamp=datetime.datetime(2019, 11, 4, 4, 57, 44, 669243)) The value is Timestamp(value=6, timestamp=datetime.datetime(2019, 11, 4, 4, 57, 44, 669243))