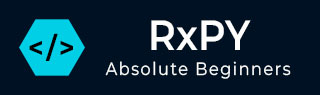
- RxPY Tutorial
- RxPY - Home
- RxPY - Overview
- RxPY - Environment Setup
- RxPY - Latest Release Updates
- RxPY - Working with Observables
- RxPY- Operators
- RxPY - Working With Subject
- RxPY - Concurrency Using Scheduler
- RxPY - Examples
- RxPY Useful Resources
- RxPY - Quick Guide
- RxPY - Useful Resources
- RxPY - Discussion
RxPY - Transformation Operators
buffer
This operator will collect all the values, from the source observable and emit them at regular intervals once the given boundary condition is satisfied.
Syntax
buffer(boundaries)
Parameters
boundaries: The input is observable that will decide when to stop so that the collected values are emitted.
Return value
The return value is observable, that will have all the values collected from source observable based and that is time duration is decided by the input observable taken.
Example
from rx import of, interval, operators as op from datetime import date test = of(1, 2,3,4,5,6,7,8,9,10) sub1 = test.pipe( op.buffer(interval(1.0)) ) sub1.subscribe(lambda x: print("The element is {0}".format(x)))
Output
E:\pyrx>python test1.py The elements are [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
ground_by
This operator will group the values coming from the source observable based on the key_mapper function given.
Syntax
group_by(key_mapper)
Parameters
key_mapper: This function will take care of extracting keys from the source observable.
Return value
It returns an observable with values grouped based on the key_mapper function.
Example
from rx import from_, interval, operators as op test = from_(["A", "B", "C", "D"]) sub1 = test.pipe( op.group_by(lambda v: v[0]) ) sub1.subscribe(lambda x: print("The element is {0}".format(x)))
Output
E:\pyrx>python testrx.py The element is <rx.core.observable.groupedobservable.GroupedObservable object at 0x000000C99A2E6550> The element is <rx.core.observable.groupedobservable.GroupedObservable object at 0x000000C99A2E65C0> The element is <rx.core.observable.groupedobservable.GroupedObservable object at 0x000000C99A2E6588> The element is <rx.core.observable.groupedobservable.GroupedObservable object at 0x000000C99A2E6550>
map
This operator will change each value from the source observable into a new value based on the output of the mapper_func given.
Syntax
map(mapper_func:None)
Parameters
mapper_func: (optional) It will change the values from the source observable based on the output coming from this function.
Example
from rx import of, interval, operators as op test = of(1, 2,3,4,5,6,7,8,9,10) sub1 = test.pipe( op.map(lambda x :x*x) ) sub1.subscribe(lambda x: print("The element is {0}".format(x)))
Output
E:\pyrx>python testrx.py The element is 1 The element is 4 The element is 9 The element is 16 The element is 25 The element is 36 The element is 49 The element is 64 The element is 81 The element is 100
scan
This operator will apply an accumulator function to the values coming from the source observable and return an observable with new values.
Syntax
scan(accumulator_func, seed=NotSet)
Parameters
accumulator_func: This function is applied to all the values from the source observable.
seed:(optional) The initial value to be used inside the accumular_func.
Return value
This operator will return an observable that will have new values based on the accumulator function applied on each value of the source observable.
Example
from rx import of, interval, operators as op test = of(1, 2,3,4,5,6,7,8,9,10) sub1 = test.pipe( op.scan(lambda acc, a: acc + a, 0)) sub1.subscribe(lambda x: print("The element is {0}".format(x)))
Output
E:\pyrx>python testrx.py The element is 1 The element is 3 The element is 6 The element is 10 The element is 15 The element is 21 The element is 28 The element is 36 The element is 45 The element is 55