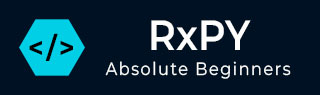
- RxPY Tutorial
- RxPY - Home
- RxPY - Overview
- RxPY - Environment Setup
- RxPY - Latest Release Updates
- RxPY - Working with Observables
- RxPY- Operators
- RxPY - Working With Subject
- RxPY - Concurrency Using Scheduler
- RxPY - Examples
- RxPY Useful Resources
- RxPY - Quick Guide
- RxPY - Useful Resources
- RxPY - Discussion
RxPY - Filtering Operators
debounce
This operator will give the values from the source observable, until the timespan given and ignore the rest of the values if time passes.
Syntax
debounce(duetime)
Parameters
duetime: this will value in seconds or instances of time, the duration that will decide the values to be returned from the source observable.
Example
from rx import of, operators as op from datetime import date test = of(1,2,3,4,5,6,7,8,9,10) sub1 = test.pipe( op.debounce(2.0) ) sub1.subscribe(lambda x: print("The value is {0}".format(x)))
Output
E:\pyrx>python testrx.py The value is 10
distinct
This operator will give all the values that are distinct from the source observable.
Syntax
distinct()
Return value
It will return an observable, where it will have distinct values from the source observable.
Example
from rx import of, operators as op from datetime import date test = of(1, 6, 15, 1, 10, 6, 40, 10, 58, 20, 40) sub1 = test.pipe( op.distinct() ) sub1.subscribe(lambda x: print("The distinct value is {0}".format(x)))
Output
E:\pyrx>python testrx.py The distinct value is 1 The distinct value is 6 The distinct value is 15 The distinct value is 10 The distinct value is 40 The distinct value is 58 The distinct value is 20
element_at
This operator will give an element from the source observable for the index given.
Syntax
element_at(index)
Parameters
index: the number starting from zero for which you need the element from the source observable.
Return value
It will return an observable with the value from the source observable with the index given.
Example
from rx import of, operators as op from datetime import date test = of(1, 6, 15, 1, 10, 6, 40, 10, 58, 20, 40) sub1 = test.pipe( op.element_at(5) ) sub1.subscribe(lambda x: print("The value is {0}".format(x)))
Output
E:\pyrx>python testrx.py The value is 6
filter
This operator will filter values from the source observable based on the predicate function given.
Syntax
filter(predicate_func)
Parameters
predicate_func: This function will decide the values to be filtered from the source observable.
Return value
It will return an observable that will have the filtered values from the source observable based on predicate function.
Example
from rx import of, operators as op from datetime import date test = of(1, 6, 15, 1, 10, 6, 40, 10, 58, 20, 40) sub1 = test.pipe( op.filter(lambda x : x %2==0) ) sub1.subscribe(lambda x: print("The filtered value is {0}".format(x)))
In the example, we have filtered all the even numbers.
Output
E:\pyrx>python testrx.py The filtered value is 6 The filtered value is 10 The filtered value is 6 The filtered value is 40 The filtered value is 10 The filtered value is 58 The filtered value is 20 The filtered value is 40
first
This operator will give the first element from the source observable.
Syntax
first(predicate_func=None)
Parameters
predicate_func: (optional) This function will decide the first element to be picked based on the condition if passed.
Return value
It will return an observable with the first value from the source observable.
Example
from rx import of, operators as op from datetime import date test = of(1, 6, 15, 1, 10, 6, 40, 10, 58, 20, 40) sub1 = test.pipe( op.first() ) sub1.subscribe(lambda x: print("The first element is {0}".format(x)))
Output
E:\pyrx>python testrx.py The first element is 1
Example 2: using predicate_func
from rx import of, operators as op from datetime import date test = of(1, 6, 15, 1, 10, 6, 40, 10, 58, 20, 40) sub1 = test.pipe( op.first(lambda x : x%2==0) ) sub1.subscribe(lambda x: print("The first element is {0}".format(x)))
Output
E:\pyrx>python test1.py The first element is 6
ignore_elements
This operator will ignore all the values from the source Observable, and only execute calls to complete or error callback functions.
Syntax
ignore_elements()
Return value
It returns an observable that will call complete or error based on the source observable.
Example
from rx import of, operators as op from datetime import date test = of(1, 6, 15, 1, 10, 6, 40, 10, 58, 20, 40) sub1 = test.pipe( op.ignore_elements() ) sub1.subscribe(lambda x: print("The first element is {0}".format(x)), lambda e: print("Error : {0}".format(e)), lambda: print("Job Done!"))
Output
E:\pyrx>python testrx.py Job Done!
last
This operator will give the last element from the source observable.
Syntax
last(predicate_func=None)
Parameters
predicate_func: (optional) This function will decide the last element to be picked based on the condition if passed.
Return value
It will return an observable with the last value from the source observable.
Example
from rx import of, operators as op from datetime import date test = of(1, 6, 15, 1, 10, 6, 40, 10, 58, 20, 40) sub1 = test.pipe( op.last() ) sub1.subscribe(lambda x: print("The last element is {0}".format(x)))
Output
E:\pyrx>python test1.py The last element is 40
skip
This operator will give back an observable, that will skip the first occurrence of count items taken as input.
Syntax
skip(count)
Parameters
count: The count is the number of times that the items will be skipped from the source observable.
Return value
It will return an observable that skips values based on the count given.
Example
from rx import of, operators as op from datetime import date test = of(1, 2,3,4,5,6,7,8,9,10) sub1 = test.pipe( op.skip(5) ) sub1.subscribe(lambda x: print("The element is {0}".format(x)))
Output
E:\pyrx>python testrx.py The element is 6 The element is 7 The element is 8 The element is 9 The element is 10
skip_last
This operator will give back an observable, that will skip the last occurrence of count items taken as input.
Syntax
skip_last(count)
Parameters
count: The count is the number of times, that the items will be skipped from the source observable.
Return value
It will return an observable that skips values based on the count given from last.
Example
from rx import of, operators as op from datetime import date test = of(1, 2,3,4,5,6,7,8,9,10) sub1 = test.pipe( op.skip_last(5) ) sub1.subscribe(lambda x: print("The element is {0}".format(x)))
Output
E:\pyrx>python testrx.py The element is 1 The element is 2 The element is 3 The element is 4 The element is 5
take
This operator will give a list of source values in continuous order based on the count given.
Syntax
take(count)
Parameters
count: The count is the number of items, that will be given from the source observable.
Return value
It will return an observable that has the values in continuous order based on count given.
Example
from rx import of, operators as op from datetime import date test = of(1, 2,3,4,5,6,7,8,9,10) sub1 = test.pipe( op.take(5) ) sub1.subscribe(lambda x: print("The element is {0}".format(x)))
Output
E:\pyrx>python testrx.py The element is 1 The element is 2 The element is 3 The element is 4 The element is 5
take_last
This operator will give a list of source values, in continuous order from last based on the count given.
Syntax
take_last(count)
Parameters
count: The count is the number of items, that will be given from the source observable.
Return value
It will return an observable, that has the values in continuous order from last based on count given.
Example
from rx import of, operators as op from datetime import date test = of(1, 2,3,4,5,6,7,8,9,10) sub1 = test.pipe( op.take_last(5) ) sub1.subscribe(lambda x: print("The element is {0}".format(x)))
Output
E:\pyrx>python testrx.py The element is 6 The element is 7 The element is 8 The element is 9 The element is 10