
- Learning Ruby on Rails
- Rails 2.1 Home
- Rails 2.1 Introduction
- Rails 2.1 Installation
- Rails 2.1 Framework
- Rails 2.1 Dir Structure
- Rails 2.1 Examples
- Rails 2.1 Database Setup
- Rails 2.1 Active Records
- Rails 2.1 Migrations
- Rails 2.1 Controllers
- Rails 2.1 Views
- Rails 2.1 Layouts
- Rails 2.1 Scaffolding
- Rails 2.1 and AJAX
- Rails 2.1 Uploads Files
- Rails 2.1 Sends Emails
- Advanced Ruby on Rails 2.1
- Rails 2.1 RMagick Guide
- Rails 2.1 Basic HTTP Auth
- Rails 2.1 Error Handling
- Rails 2.1 Routes System
- Rails 2.1 Unit Testing
- Advanced Ruby on Rails 2.1
- Rails 2.1 Tips & Tricks
- Quick Reference Guide
- Quick Reference Guide
- Ruby on Rails 2.1 Useful Resources
- Ruby on Rails 2.1 - Resources
- Ruby on Rails 2.1 - Discussion
Ruby on Rails 2.1 - HTTP Basic Authentication
Rails provides various ways of implementing authentication and authorization. But the simplest one is a new module, which has been added in Rails 2.0. This module is a great way to do API authentication over SSL.
To use this authentication, you will need to use SSL for traffic transportation. In our tutorial, we are going to test it without an SSL.
Let us start with our library example that we have discussed throughout the tutorial. We do not have much to do to implement authentication. We will add a few lines in blue in our ~library/app/controllers/book_controller.rb:
Finally, your book_controller.rb file will look as follows −
class BookController < ApplicationController USER_ID, PASSWORD = "zara", "pass123" # Require authentication only for edit and delete operation before_filter :authenticate, :only => [ :edit, :delete ] def list @books = Book.find(:all) end def show @book = Book.find(params[:id]) end def new @book = Book.new @subjects = Subject.find(:all) end def create @book = Book.new(params[:book]) if @book.save redirect_to :action => 'list' else @subjects = Subject.find(:all) render :action => 'new' end end def edit @book = Book.find(params[:id]) @subjects = Subject.find(:all) end def update @book = Book.find(params[:id]) if @book.update_attributes(params[:book]) redirect_to :action => 'show', :id => @book else @subjects = Subject.find(:all) render :action => 'edit' end end def delete Book.find(params[:id]).destroy redirect_to :action => 'list' end def show_subjects @subject = Subject.find(params[:id]) end private def authenticate authenticate_or_request_with_http_basic do |id, password| id == USER_ID && password == PASSWORD end end end
Let us explain these new lines −
The first line is just to define the user ID and password to access various pages.
In the second line, we have put before_filter, which is used to run the configured method authenticate before any action in the controller. A filter may be limited to specific actions by declaring the actions to include or exclude. Both options accept single actions (:only => :index) or arrays of actions (:except => [:foo, :bar]). So here we have put authentication for edit and delete operations only.
Due to the second line, whenever you would try to edit or delete a book record, it will execute private authenticate method.
A private authenticate method is calling uthenticate_or_request_with_http_basic method, which comprises of a block and displays a dialogue box to ask for User ID and Password to proceed. If you enter a correct user ID and password then it will proceed, otherwise it would display ‘access denied’.
Now, try to edit or delete any available record, to do so you would have to go through the authentication process using the following dialogue box.
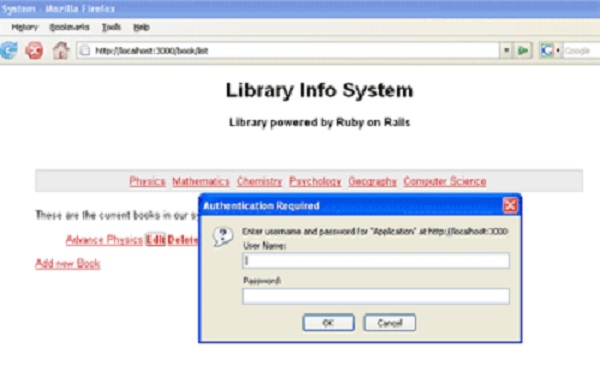