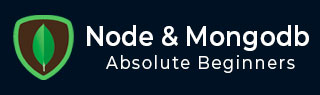
- Node & MongoDB Tutorial
- Node & MongoDB - Home
- Node & MongoDB - Overview
- Node & MongoDB - Environment Setup
- Node & MongoDB Examples
- Node & MongoDB - Connect Database
- Node & MongoDB - Show Databases
- Node & MongoDB - Drop Database
- Node & MongoDB - Create Collection
- Node & MongoDB - Drop Collection
- Node & MongoDB - Display Collections
- Node & MongoDB - Insert Document
- Node & MongoDB - Select Document
- Node & MongoDB - Update Document
- Node & MongoDB - Delete Document
- Node & MongoDB - Embedded Documents
- Node & MongoDB - Limiting Records
- Node & MongoDB - Sorting Records
- Node & MongoDB Useful Resources
- Node & MongoDB - Quick Guide
- Node & MongoDB - Useful Resources
- Node & MongoDB - Discussion
Node & MongoDB - Show Databases
To show databases, you can use admin.listDatabases() method to get the name of all the databases where admin represents the admin class.
MongoClient.connect(url, function(error, client) { // Use the admin database for the operation const adminDb = client.db('myDb').admin(); // List all the available databases adminDb.listDatabases(function(err, dbs) { console.log(dbs); }); });
Example
Try the following example to connect to a MongoDB server −
Copy and paste the following example as mongodb_example.js −
const MongoClient = require('mongodb').MongoClient; // Prepare URL const url = "mongodb://localhost:27017/"; // make a connection to the database MongoClient.connect(url, function(error, client) { if (error) throw error; console.log("Connected!"); // Use the admin database for the operation const adminDb = client.db('myDb').admin(); // List all the available databases adminDb.listDatabases(function(err, dbs) { console.log(dbs); }); // close the connection client.close(); });
Output
Execute the mysql_example.js script using node and verify the output.
node mongodb_example.js Connected! { databases: [ { name: 'admin', sizeOnDisk: 40960, empty: false }, { name: 'config', sizeOnDisk: 36864, empty: false }, { name: 'local', sizeOnDisk: 73728, empty: false } ], totalSize: 151552, ok: 1 }
Advertisements
To Continue Learning Please Login