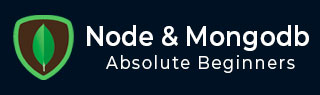
- Node & MongoDB Tutorial
- Node & MongoDB - Home
- Node & MongoDB - Overview
- Node & MongoDB - Environment Setup
- Node & MongoDB Examples
- Node & MongoDB - Connect Database
- Node & MongoDB - Show Databases
- Node & MongoDB - Drop Database
- Node & MongoDB - Create Collection
- Node & MongoDB - Drop Collection
- Node & MongoDB - Display Collections
- Node & MongoDB - Insert Document
- Node & MongoDB - Select Document
- Node & MongoDB - Update Document
- Node & MongoDB - Delete Document
- Node & MongoDB - Embedded Documents
- Node & MongoDB - Limiting Records
- Node & MongoDB - Sorting Records
- Node & MongoDB Useful Resources
- Node & MongoDB - Quick Guide
- Node & MongoDB - Useful Resources
- Node & MongoDB - Discussion
Node & MongoDB - Overview
What is Node.js?
Node.js is a server−side platform built on Google Chrome's JavaScript Engine (V8 Engine). Node.js was developed by Ryan Dahl in 2009 and its latest version is v0.10.36. The definition of Node.js as supplied by its official documentation is as follows −
Node.js is a platform built on Chrome's JavaScript runtime for easily building fast and scalable network applications. Node.js uses an event-driven, non-blocking I/O model that makes it lightweight and efficient, perfect for data-intensive real-time applications that run across distributed devices.
Node.js is an open source, cross-platform runtime environment for developing server-side and networking applications. Node.js applications are written in JavaScript, and can be run within the Node.js runtime on OS X, Microsoft Windows, and Linux.
Node.js also provides a rich library of various JavaScript modules which simplifies the development of web applications using Node.js to a great extent.
Node.js = Runtime Environment + JavaScript Library
mongodb
mongodb is node.js driver to connect and perform database operations on MongoDB. To install mongodb, run the following npm command.
npm install mongodb + mongodb@3.6.9 added 1 package from 1 contributor in 1.781s
Creating/Connecting to Database
Once mongoClient is instantiated, its connect() method can be used to get connection to a database.
// MongoDBClient const client = new MongoClient(url, { useUnifiedTopology: true }); // make a connection to the database client.connect(function(error) { if (error) throw error; console.log("Connected!"); // create or connect to database const db = client.db(database); // close the connection client.close(); });
In case database is not present then above command will create the same.
In subsequent chapters, we'll see the various operations on MongoDB using Node.
To Continue Learning Please Login