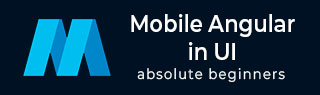
- Mobile Angular UI Tutorial
- Mobile Angular UI - Home
- Mobile Angular UI - Overview
- Mobile Angular UI - Installation
- Mobile Angular UI - Project Setup
- Mobile Angular UI - My First App
- Mobile Angular UI - Layouts
- Mobile Angular UI - Components
- Mobile Angular UI - Dropdowns
- Mobile Angular UI - Accordions
- Mobile Angular UI - Tabs
- Mobile Angular UI - Drag and Drop
- Mobile Angular UI - Scrollable Areas
- Mobile Angular UI - Forms
- Mobile Angular UI - Swipe Gestures
- Mobile Angular UI - Toggle Switch
- Mobile Angular UI - Sections
- Mobile Angular UI - Core Details
- Mobile Angular UI - Touch Events
- Mobile Angular UI - PhoneGap & Cordova
- Mobile Angular UI - Creating APK File
- Mobile Angular UI - APP Development
- Mobile Angular UI - Examples
- Mobile Angular UI Resources
- Mobile Angular UI - Quick Guide
- Mobile Angular UI - Useful Resources
- Mobile Angular UI - Discussion
Mobile Angular UI - Activelinks
To make use of activelinks from mobile angular core module add, mobile-angular-ui.core.activeLinks as a dependency. The example is as follows −
angular.module('myFirstApp', ['mobile-angular-ui.core.activeLinks']);
The class .active is added to the <a> tag whose href matches the current location.For <a> tag with href you should always see the .active class added. It is added/removed when the $locationChangeSuccess or $includeContentLoaded is fired.
For example, consider the following app −
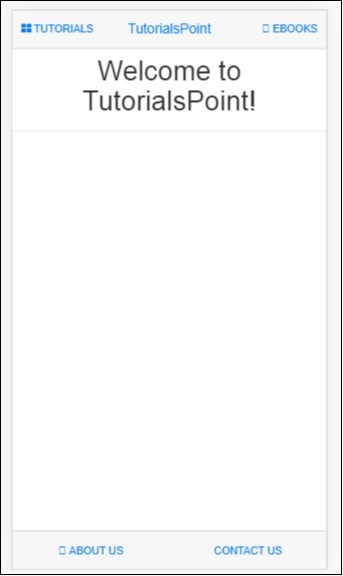
The text: Welcome to TutorialsPoint, comes from home/home.html.
<div class="list-group text-center"> list-group-item-home"> <h1>{{msg}}</h1> </div> </div>
The details of the variable msg, and also adding home/home.html contents is available in app.js −
'use strict'; // // Here is how to define your module // has dependent on mobile-angular-ui // var app=angular.module( 'myFirstApp', [ 'ngRoute', 'mobile-angular-ui', 'mobile-angular-ui.gestures', ] ); app.config(function($routeProvider, $locationProvider) { $routeProvider .when("/", { templateUrl : "src/home/home.html" }); $locationProvider.html5Mode({enabled:true, requireBase:false}); }); app.controller('MainController', function($rootScope, $scope, $routeParams) { $scope.msg="Welcome to TutorialsPoint!"; });
The templateUrl, is replaced with src/home/home.html when the location starts with /. Inside index.html we have a sidebar where the menu is added −
index.html
<!DOCTYPE html> <html> <head> <meta charset="utf-8" /> <title>Mobile Angular UI Demo</title> <meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1" /> <meta name="apple-mobile-web-app-capable" content="yes" /> <meta name="viewport" content="user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimal-ui" /> <meta name="apple-mobile-web-app-status-bar-style" content="yes" /> <link rel="shortcut icon" href="/assets/img/favicon.png" type="image/x-icon" /> <link rel="stylesheet" href="node_modules/mobile-angular-ui/dist/css/mobile-angular-ui-hover.min.css" /> <link rel="stylesheet" href="node_modules/mobile-angular-ui/dist/css/mobile-angular-ui-base.min.css" /> <link rel="stylesheet" href="node_modules/mobile-angular-ui/dist/css/mobile-angular-ui-desktop.min.css" /> <script src="node_modules/angular/angular.min.js"></script> <script src="node_modules/angular-route/angular-route.min.js"></script> <script src="node_modules/mobile-angular-ui/dist/js/mobile-angular-ui.min.js"></script> <script src="node_modules/angular-route/angular-route.min.js"></script> <script src="node_modules/mobile-angular-ui/dist/js/mobile-angular-ui.gestures.min.js"></script> <link rel="stylesheet" href="src/css/app.css" /> <script src="src/js/app.js"></script> <style> a.active { color: blue; } </style> </head> <body ng-app="myFirstApp" ng-controller="MainController" class="listening"> <!-- Sidebars --> <div class="sidebar sidebar-left "> <div class="scrollable"> <h1 class="scrollable-header app-name">Tutorials</h1> <div class="scrollable-content"> <div class="list-group" ui-turn-off='uiSidebarLeft'> <a class="list-group-item" href="/">Home Page </a> <a class="list-group-item" href="#/academic"><i class="fa fa-caret-right"></i>Academic Tutorials </a> <a class="list-group-item" href="#/bigdata"><i class="fa fa-caret-right"></i>Big Data & Analytics </a> <a class="list-group-item" href="#/computerProg"><i class="fa fa-caret-right"></i>Computer Programming </a> <a class="list-group-item" href="#/computerscience"><i class="fa fa-caret-right"></i>Computer Science </a> <a class="list-group-item" href="#/databases"><i class="fa fa-caret-right"></i>Databases </a> <a class="list-group-item" href="#/devops"><i class="fa fa-caret-right"></i>DevOps </a> </div> </div> </div> </div> <div class="sidebar sidebar-right"> <div class="scrollable"> <h1 class="scrollable-header app-name">eBooks</h1> <div class="scrollable-content"> <div class="list-group" ui-toggle="uiSidebarRight"> <a class="list-group-item" href="#/php"> <i class="fa fa-caret-right"></i>PHP </a> <a class="list-group-item" href="#/Javascript"> <i class="fa fa-caret-right"></i>Javascript </a> </div> </div> </div> </div> <div class="app"> <div class="navbar navbar-app navbar-absolute-top"> <div class="navbar-brand navbar-brand-center" ui-yield-to="title"> TutorialsPoint </div> <div class="btn-group pull-left"> <div ui-toggle="uiSidebarLeft" class="btn sidebar-left-toggle"> <i class="fa fa-th-large "></i> Tutorials </div> </div> <div class="btn-group pull-right" ui-yield-to="navbarAction"> <div ui-toggle="uiSidebarRight" class="btn sidebar-right-toggle"> <i class="fal fa-search"></i> eBooks </div> </div> </div> <div class="navbar navbar-app navbar-absolute-bottom"> <div class="btn-group justified"> <a ui-turn-on="aboutus_modal" class="btn btn-navbar"> <i class="fal fa-globe"></i> About us</a> <a ui-turn-on="contactus_overlay" class="btn btn-navbar"> <i class="fal fa-map-marker-alt"></i> Contact us</a> </div> </div> <!-- App body --> <div class='app-body'> <div class='app-content'> <ng-view></ng-view> </div> </div> </div><!-- ~ .app --> <!-- Modals and Overlays --> <div ui-yield-to="modals"></div> </body> </html>
The sidebar details are as follows −
<div class="sidebar sidebar-left "> <div class="scrollable"> <h1 class="scrollable-header app-name">Tutorials</h1> <div class="scrollable-content"> <div class="list-group" ui-turn-off='uiSidebarLeft'> <a class="list-group-item" href="/">Home Page </a> <a class="list-group-item" href="#/academic"><i class="fa fa-caret-right"></i>Academic Tutorials </a> <a class="list-group-item" href="#/bigdata"><i class="fa fa-caret-right"></i>Big Data & Analytics </a> <a class="list-group-item" href="#/computerProg"><i class="fa fa-caret-right"></i>Computer Programming </a> <a class="list-group-item" href="#/computerscience"><i class="fa fa-caret-right"></i>Computer Science </a> <a class="list-group-item" href="#/databases"><i class="fa fa-caret-right"></i>Databases </a> <a class="list-group-item" href="#/devops"><i class="fa fa-caret-right"></i>DevOps </a> </div> </div> </div> </div>
When you happen to open the sidebar of the left side, you will see the Home Tag highlighted as shown below. This is because the .active class is added to the tag that is active at the moment.
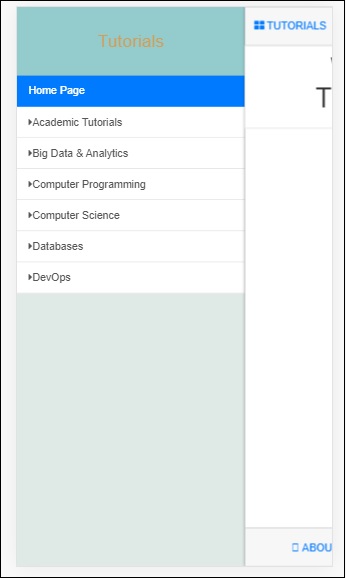
If you inspect using the browser developer tool, you should see the active class added to <a> tag.
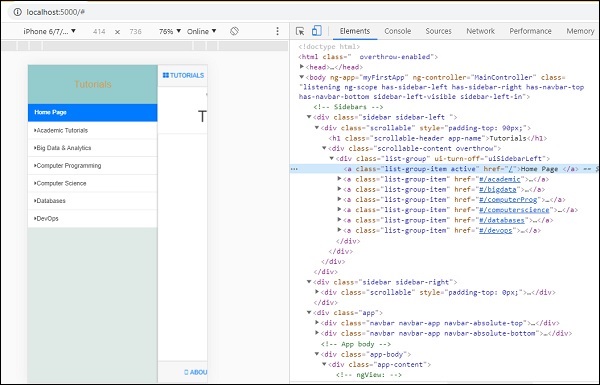
To Continue Learning Please Login