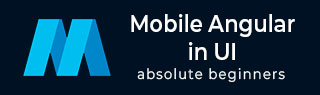
- Mobile Angular UI Tutorial
- Mobile Angular UI - Home
- Mobile Angular UI - Overview
- Mobile Angular UI - Installation
- Mobile Angular UI - Project Setup
- Mobile Angular UI - My First App
- Mobile Angular UI - Layouts
- Mobile Angular UI - Components
- Mobile Angular UI - Dropdowns
- Mobile Angular UI - Accordions
- Mobile Angular UI - Tabs
- Mobile Angular UI - Drag and Drop
- Mobile Angular UI - Scrollable Areas
- Mobile Angular UI - Forms
- Mobile Angular UI - Swipe Gestures
- Mobile Angular UI - Toggle Switch
- Mobile Angular UI - Sections
- Mobile Angular UI - Core Details
- Mobile Angular UI - Touch Events
- Mobile Angular UI - PhoneGap & Cordova
- Mobile Angular UI - Creating APK File
- Mobile Angular UI - APP Development
- Mobile Angular UI - Examples
- Mobile Angular UI Resources
- Mobile Angular UI - Quick Guide
- Mobile Angular UI - Useful Resources
- Mobile Angular UI - Discussion
Mobile Angular UI - My First App
In this chapter, we will create our first app that will run on mobile as well as on desktop.
The project setup we created in previous chapter has the following structure −
uiformobile/ node_modules/ src/ package.json index.html
Follow the steps to build a simple UI using Mobile Angular UI.
Step 1
Add following css files in the html head section as shown below −
<!-- Required for desktop --> <link rel="stylesheet" href="/node_modules/mobile-angular-ui/dist/css/mobile-angular-ui-hover.min.css" /> <!-- Mandatory CSS --> <link rel="stylesheet" href="/node_modules/mobile-angular-ui/dist/css/mobile-angular-ui-base.min.css" /> <!-- Required for desktop --> <link rel="stylesheet" href="/node_modules/mobile-angular-ui/dist/css/mobile-angular-ui-desktop.min.css" />
Next add the js files −
<script src="/node_modules/angular/angular.min.js"></script> <script src="/node_modules/angular-route/angular-route.min.js"></script> <script src="/node_modules/mobile-angular-ui/dist/js/mobile-angular-ui.min.js"></script>
The index.html file will look as follows −
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>My App</title> <!-- Required for desktop --> <link rel="stylesheet" href="/node_modules/mobile-angular-ui/dist/css/mobile-angular-ui-hover.min.css" /> <!-- Mandatory CSS --> <link rel="stylesheet" href="/node_modules/mobile-angular-ui/dist/css/mobile-angular-ui-base.min.css" /> <!-- Required for desktop --> <link rel="stylesheet" href="/node_modules/mobile-angular-ui/dist/css/mobile-angular-ui-desktop.min.css" /> <script src="/node_modules/angular/angular.min.js"></script> <script src="/node_modules/angular-route/angular-route.min.js"></script> <script src="/node_modules/mobile-angular-ui/dist/js/mobile-angular-ui.min.js"></script> </head> <body> </body> </html>
Step 2
We will see the basic layout of the mobile angular UI as below −
<body ng-app="myFirstApp"> <!-- Sidebars --> <div class="sidebar sidebar-left"><!-- ... --></div> <div class="sidebar sidebar-right"><!-- ... --></div> <div class="app"> <div class="navbar navbar-app navbar-absolute-top"><!-- Top Navbar --></div> <div class="navbar navbar-app navbar-absolute-bottom"><!-- Bottom Navbar --></div> <!-- App body --> <div class='app-body'> <div class='app-content'> <ng-view></ng-view> </div> </div> </div><!-- ~ .app --> <!-- Modals and Overlays --> <div ui-yield-to="modals"></div> </body>
Step 3
Create a js/ folder in src/ and add app.js to it.
Define the module and add Mobile angular UI and Angular Route as dependency as shown below −
<script type="text/javascript"> 'ngRoute', angular.module('myFirstApp', [ 'mobile-angular-ui' ]); </script>
Add ng-app=”myFirstApp” to the <body> tag −
<body ng-app="myFirstApp">
The mobile-angular-ui module has the following list of modules −
angular.module('mobile-angular-ui', [ 'mobile-angular-ui.core.activeLinks', /* adds .active class to current links */ 'mobile-angular-ui.core.fastclick', /* polyfills overflow: auto */ 'mobile-angular-ui.core.sharedState', /* SharedState service and directives */ 'mobile-angular-ui.core.outerClick', /* outerClick directives */ 'mobile-angular-ui.components.modals', /* modals and overlays */ 'mobile-angular-ui.components.switch', /* switch form input */ 'mobile-angular-ui.components.sidebars', /* sidebars */ 'mobile-angular-ui.components.scrollable', /* uiScrollable directives */ 'mobile-angular-ui.components.capture', /* uiYieldTo and uiContentFor directives */ 'mobile-angular-ui.components.navbars' /* navbars */ ]);
The mobile-angular-ui.min.js, has all the above core and components modules in it. You can also load the required components as per your requirement instead of loading the entire mobile-angular-ui.min.js.
Step 4
Add controller to your body tag as shown below −
<body ng-app="myFirstApp" ng-controller="MainController">
Step 5
In the basic layout, we have added <ng-view></ng-view>, that will load the views for us.
Let us define the routes in app.js using ngRoute. The files that are required for routing are already added in the head section.
Create a folder home/ in src/. Add home.html to it with the following details −
<div class="list-group text-center"> <div class="list-group-item list-group-item-home"> <h1>{{msg}}</h1> </div> </div>
Now when we start the app, by default, we want home.html to be displayed inside <ng-view></ng-view>.
The routing is configured inside app.config() as shown below −
app.config(function($routeProvider, $locationProvider) { $routeProvider .when("/", { templateUrl : "src/home/home.html" }); $locationProvider.html5Mode({enabled:true, requireBase:false}); });
Step 6
We have added {{msg}} inside home.html as shown below −
<div class="list-group text-center"> <div class="list-group-item list-group-item-home"> <h1>{{msg}}</h1> </div> </div>
Let us define the same in the controller as shown below −
app.controller('MainController', function($rootScope, $scope, $routeParams) { $scope.msg="Welcome to Tutorialspoint!" });
Step 7
Now run the command to start the app using the below command −
node server.js
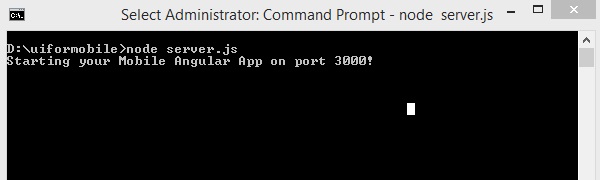
Step 8
Load your app at http://localhost:3000 in the browser −
You will see the following screen in mobile mode −
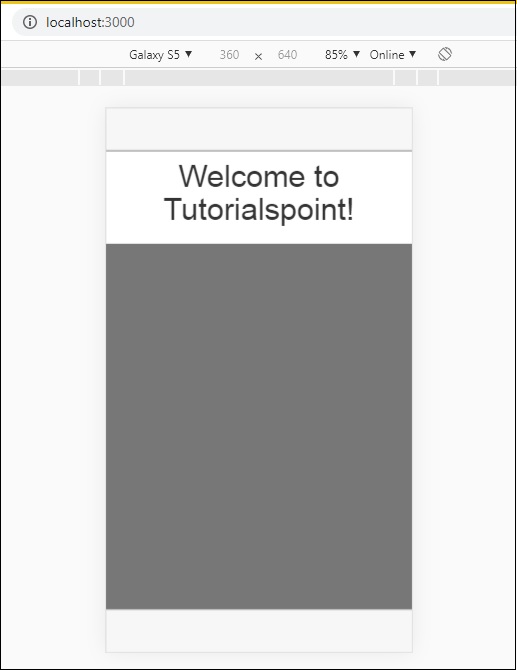
You will see the following screen in Desktop mode −
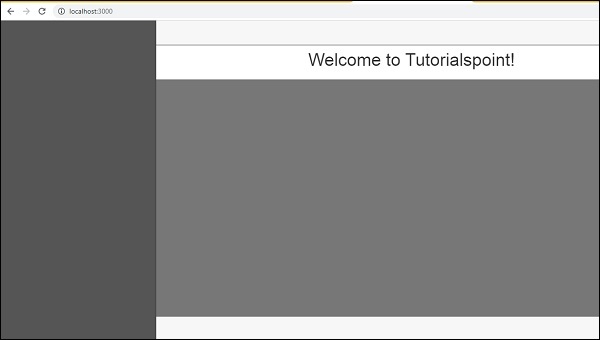
Let us understand the details of each component in Mobile Angular UI in the next chapters.
Here is the final code for the above display. The folder structure so far is as follows −
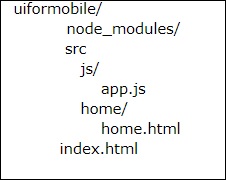
index.html
<!DOCTYPE html> <html> <head> <meta charset="utf-8" /> <title>Mobile Angular UI Demo</title> <meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1" /> <meta name="apple-mobile-web-app-capable" content="yes" /> <meta name="viewport" content="user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimal-ui" /> <meta name="apple-mobile-web-app-status-bar-style" content="yes" /> <link rel="shortcut icon" href="/assets/img/favicon.png" type="image/x-icon" /> <link rel="stylesheet" href="node_modules/mobile-angular-ui/dist/css/mobile-angular-ui-hover.min.css" /> <link rel="stylesheet" href="node_modules/mobile-angular-ui/dist/css/mobile-angular-ui-base.min.css" /> <link rel="stylesheet" href="node_modules/mobile-angular-ui/dist/css/mobile-angular-ui-desktop.min.css" /> <script src="node_modules/angular/angular.min.js"></script> <script src="node_modules/angular-route/angular-route.min.js"></script> <script src="node_modules/mobile-angular-ui/dist/js/mobile-angular-ui.min.js"></script> <script src="src/js/app.js"></script> </head> <body ng-app="myFirstApp" ng-controller="MainController"> <!-- Sidebars --> <div class="sidebar sidebar-left"><!-- ... --></div> <div class="sidebar sidebar-right"><!-- ... --></div> <div class="app"> <div class="navbar navbar-app navbar-absolute-top"><!-- Top Navbar --></div> <div class="navbar navbar-app navbar-absolute-bottom"><!-- Bottom Navbar --></div> <!-- App body --> <div class='app-body'> <div class='app-content'> <ng-view></ng-view> </div> </div> </div><!-- ~ .app --> <!-- Modals and Overlays --> <div ui-yield-to="modals"></div> </body> </html>
js/app.js
/* eslint no-alert: 0 */ 'use strict'; // // Here is how to define your module // has dependent on mobile-angular-ui // var app=angular.module('myFirstApp', [ 'ngRoute', 'mobile-angular-ui' ]); app.config(function($routeProvider, $locationProvider) { $routeProvider .when("/", { templateUrl : "src/home/home.html" }); $locationProvider.html5Mode({enabled:true, requireBase:false}); }); app.controller('MainController', function($rootScope, $scope, $routeParams) { $scope.msg="Welcome to Tutorialspoint!" });
home/home.html
<div class="list-group text-center"> <div class="list-group-item list-group-item-home"> <h1>{{msg}}</h1> </div> </div>
To Continue Learning Please Login