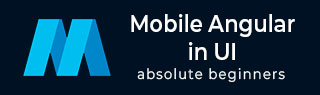
- Mobile Angular UI Tutorial
- Mobile Angular UI - Home
- Mobile Angular UI - Overview
- Mobile Angular UI - Installation
- Mobile Angular UI - Project Setup
- Mobile Angular UI - My First App
- Mobile Angular UI - Layouts
- Mobile Angular UI - Components
- Mobile Angular UI - Dropdowns
- Mobile Angular UI - Accordions
- Mobile Angular UI - Tabs
- Mobile Angular UI - Drag and Drop
- Mobile Angular UI - Scrollable Areas
- Mobile Angular UI - Forms
- Mobile Angular UI - Swipe Gestures
- Mobile Angular UI - Toggle Switch
- Mobile Angular UI - Sections
- Mobile Angular UI - Core Details
- Mobile Angular UI - Touch Events
- Mobile Angular UI - PhoneGap & Cordova
- Mobile Angular UI - Creating APK File
- Mobile Angular UI - APP Development
- Mobile Angular UI - Examples
- Mobile Angular UI Resources
- Mobile Angular UI - Quick Guide
- Mobile Angular UI - Useful Resources
- Mobile Angular UI - Discussion
Mobile Angular UI - Touch Events
To work with Touch and its events you need to add the following module −
mobile-angular-ui.gestures
If you are just interested in the touch module, then you can add only mobile-angular-ui.gestures.touch.
$touch is a service available with touch module. It will work on any input devices you want to work with. It gives details like movement, duration, velocity, direction, etc.
Methods in $touch
The following are the available methods in $touch −
bind
Let us take a look at the bind method.
Syntax
$touch.bind(element, eventHandlers, [options])
Parameters
element − html element you want to deal with touch details.
eventHandlers − An object with handlers for specific touch events. The eventHandlers available are −
start − it's a callback for touchstart event.
end − it’s a callback event for touchend.
move − it’s a callback for touchmove event.
cancel − it’s a callback for touchcancel event.
options − It is an object that can have details as follows −
movementThreshold − An integer value. The number of pixels of movement before start to trigger touchmove handlers.
valid − Its a function that returns a boolean value that decides if a touch should be handled or ignored.
sensitiveArea − It can be a function, or element or BoundingClientRect. Sensitive area defines boundaries to release touch when movement is outside.
pointerTypes − It is an array of pointer that has keys which are a subset of default pointer events map.
Types available in $touch
The following are the types available in $touch −
Property | Type | Description |
---|---|---|
type | string | This will tell you the type of event. For example − touchmove, touchstart, touchend, touchcancel |
timestamp | Date | The timestamp when the touch happened |
duration | int | Difference between current touch event and touch start |
startX | float | X coordinate of touchstart |
startY | float | Y coordinate of touchstart |
prevX | float | X coordinate of the previously happened touchstart or touchmove |
prevY | float | Y coordinate of the previously happened touchstart or touchmove |
x | float | X coordinate of touch event |
y | float | Y coordinate of touch event |
step | float | The distance between prevX,prevY and x,y points |
stepX | float | The distance between prevX and x points |
stepY | float | The distance between prevY and y points |
velocity | float | Velocity in pixels of a touch event per second |
averageVelocity | float | Average velocity of touchstart event per second |
distance | float | The distance between startX, startY and x,y points |
distanceX | float | The distance between startX and x points |
distanceY | float | The distance between startY and y points |
total | float | The total movement i.e horizontal and vertical movement done across the device |
totalX | float | The total movement i.e horizontal direction.It also includes turnarounds and changes of direction |
totalY | float | The total movement i.e vertical direction.It also includes turnarounds and changes of direction |
direction | float | The left, top, bottom, right direction location of touch |
angle | float | The angle in degrees from the x and y axis |
Here is a working example showing the touch types.
index.html
<!DOCTYPE html> <html> <head> <meta charset="utf-8" /> <title>Mobile Angular UI Demo</title> <meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1" /> <meta name="apple-mobile-web-app-capable" content="yes" /> <meta name="viewport" content="user-scalable=no, initial-scale=1.0, maximum-scale=1.0, minimal-ui" /> <meta name="apple-mobile-web-app-status-bar-style" content="yes" /> <link rel="shortcut icon" href="/assets/img/favicon.png" type="image/x-icon" /> <link rel="stylesheet" href="node_modules/mobile-angular-ui/dist/css/mobile-angular-ui-hover.min.css" /> <link rel="stylesheet" href="node_modules/mobile-angular-ui/dist/css/mobile-angular-ui-base.min.css" /> <link rel="stylesheet" href="node_modules/mobile-angular-ui/dist/css/mobile-angular-ui-desktop.min.css" /> <script src="node_modules/angular/angular.min.js"></script> <script src="node_modules/angular-route/angular-route.min.js"></script> <script src="node_modules/mobile-angular-ui/dist/js/mobile-angular-ui.min.js"></script> <script src="node_modules/angular-route/angular-route.min.js"></script> <script src="node_modules/mobile-angular-ui/dist/js/mobile-angular-ui.gestures.min.js"></script> <link rel="stylesheet" href="src/css/app.css" /> <script src="src/js/app.js"></script> <style> a.active { color: blue; } </style> </head> <body ng-app="myFirstApp" ng-controller="MainController" class="listening"> <!-- Sidebars --> <div class="sidebar sidebar-left "> <div class="scrollable"> <h1 class="scrollable-header app-name">Tutorials</h1> <div class="scrollable-content"> <div class="list-group" ui-turn-off='uiSidebarLeft'> <a class="list-group-item" href="/">Home Page </a> <a class="list-group-item" href="#/academic"><i class ="fa fa-caret-right"></i>Academic Tutorials </a> <a class="list-group-item" href="#/bigdata"><i class ="fa fa-caret-right"></i>Big Data & Analytics </a> <a class="list-group-item" href="#/computerProg"><i class="fa fa-caret-right"></i>Computer Programming </a> <a class="list-group-item" href="#/computerscience"><i class="fa fa-caret-right"></i>Computer Science </a> <a class="list-group-item" href="#/databases"><i class="fa fa-caret-right"></i>Databases </a> <a class="list-group-item" href="#/devops"><i class="fa fa-caret-right"></i>DevOps </a> </div> </div> </div> </div> <div class="sidebar sidebar-right"> <div class="scrollable"> <h1 class="scrollable-header app-name">eBooks</h1> <div class="scrollable-content"> <div class="list-group" ui-toggle="uiSidebarRight"> <a class="list-group-item" href="#/php"><i class="fa fa-caret-right"></i>PHP </a> <a class="list-group-item" href="#/Javascript"><i class="fa fa-caret-right"></i>Javascript </a> </div> </div> </div> </div> <div class="app"> <div class="navbar navbar-app navbar-absolute-top"> <div class="navbar-brand navbar-brand-center" ui-yield-to="title"> TutorialsPoint </div> <div class="btn-group pull-left"> <div ui-toggle="uiSidebarLeft" class="btn sidebar-left-toggle"> <i class="fa fa-th-large "></i> Tutorials </div> </div> <div class="btn-group pull-right" ui-yield-to="navbarAction"> <div ui-toggle="uiSidebarRight" class="btn sidebar-right-toggle"> <i class="fal fa-search"></i> eBooks </div> </div> </div> <div class="navbar navbar-app navbar-absolute-bottom"> <div class="btn-group justified"> <a ui-turn-on="aboutus_modal" class="btn btn-navbar"><i class="fal fa-globe"></i> About us</a> <a ui-turn-on="contactus_overlay" class="btn btn-navbar"><i class="fal fa-map-marker-alt"></i> Contact us</a> </div> </div> <!-- App body --> <div class='app-body'> <div class='app-content'> <ng-view></ng-view> </div> </div> </div><!-- ~ .app --> <!-- Modals and Overlays --> <div ui-yield-to="modals"></div> </body> </html>
There is touchtest directive added in app.js that makes use of the $touch.bind method.
directive('touchtest', ['$touch', function($touch) { return { restrict: 'C', link: function($scope, elem) { $scope.touch=null; $touch.bind(elem, { start: function(touch) { $scope.containerRect=elem[0].getBoundingClientRect(); $scope.touch=touch; $scope.$apply(); }, cancel: function(touch) { $scope.touch=touch; $scope.$apply(); }, move: function(touch) { $scope.touch=touch; $scope.$apply(); }, end: function(touch) { $scope.touch=touch; $scope.$apply(); } }); } }; }]);
The complete code inside app.js is as follows −
/* eslint no-alert: 0 */ 'use strict'; // // Here is how to define your module // has dependent on mobile-angular-ui // var app=angular.module('myFirstApp', ['ngRoute', 'mobile-angular-ui', 'mobile-angular-ui.gestures', ]); app.config(function($routeProvider, $locationProvider) { $routeProvider .when("/", { templateUrl : "src/home/home.html" }); $locationProvider.html5Mode({enabled:true, requireBase:false}); }); app.directive('touchtest', ['$touch', function($touch) { return { restrict: 'C', link: function($scope, elem) { $scope.touch=null; $touch.bind(elem, { start: function(touch) { $scope.containerRect=elem[0].getBoundingClientRect(); $scope.touch=touch; $scope.$apply(); }, cancel: function(touch) { $scope.touch=touch; $scope.$apply(); }, move: function(touch) { $scope.touch=touch; $scope.$apply(); }, end: function(touch) { $scope.touch=touch; $scope.$apply(); } }); } }; }]); app.controller('MainController', function($rootScope, $scope, $routeParams) { $scope.msg="Welcome to TutorialsPoint!"; });
src/home/home.html
The directive touchtest is used inside the html as shown below −
<div class="section touchtest"> <h4>Touch Around on the screen to see the values changing</h4> <div> <p>type: {{touch.type}}</p> <p>direction: {{touch.direction == null ? '-' : touch.direction}}</p> <p>point: [{{touch.x}}, {{touch.y}}]</p> <p>step: {{touch.step}} [{{touch.stepX}}, {{touch.stepY}}]</p> <p>distance: {{touch.distance}} [{{touch.distanceX}}, {{touch.distanceY}}]</p> <p>total: {{touch.total}} [{{touch.totalX}}, {{touch.totalY}}]</p> <p>velocity: {{touch.velocity}} px/sec</p> <p>averageVelocity: {{touch.averageVelocity}} px/sec</p> <p>angle: {{touch.angle == null ? '-' : touch.angle}} deg</p> </div> </div>
Now let us test the display in the browser. The resultant screen is as follows −
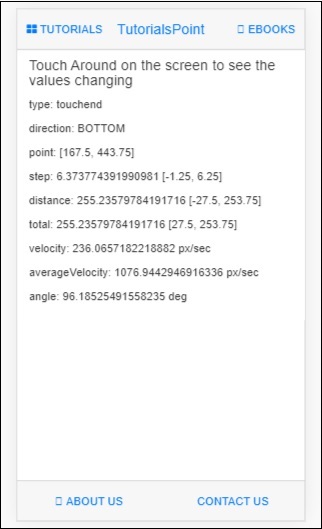
To Continue Learning Please Login