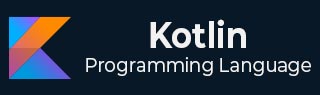
- Kotlin Tutorial
- Kotlin - Home
- Kotlin - Overview
- Kotlin - Environment Setup
- Kotlin - Architecture
- Kotlin - Basic Syntax
- Kotlin - Comments
- Kotlin - Keywords
- Kotlin - Variables
- Kotlin - Data Types
- Kotlin - Operators
- Kotlin - Booleans
- Kotlin - Strings
- Kotlin - Arrays
- Kotlin - Ranges
- Kotlin - Functions
- Kotlin Control Flow
- Kotlin - Control Flow
- Kotlin - if...Else Expression
- Kotlin - When Expression
- Kotlin - For Loop
- Kotlin - While Loop
- Kotlin - Break and Continue
- Kotlin Collections
- Kotlin - Collections
- Kotlin - Lists
- Kotlin - Sets
- Kotlin - Maps
- Kotlin Objects and Classes
- Kotlin - Class and Objects
- Kotlin - Constructors
- Kotlin - Inheritance
- Kotlin - Abstract Classes
- Kotlin - Interface
- Kotlin - Visibility Control
- Kotlin - Extension
- Kotlin - Data Classes
- Kotlin - Sealed Class
- Kotlin - Generics
- Kotlin - Delegation
- Kotlin - Destructuring Declarations
- Kotlin - Exception Handling
- Kotlin Useful Resources
- Kotlin - Quick Guide
- Kotlin - Useful Resources
- Kotlin - Discussion
Kotlin - Data Classes
In this chapter, we will learn about Kotlin Data Classes. A Kotlin Data Class is used to hold the data only and it does not provide any other functionality apart from holding data.
There are following conditions for a Kotlin class to be defined as a Data Class:
The primary constructor needs to have at least one parameter.
All primary constructor parameters need to be marked as val or var.
Data classes cannot be abstract, open, sealed, or inner.
The class may extend other classes or implement interfaces. If you are using Kotlin version before 1.1, the class can only implement interfaces.
Syntax
It's simple to define a Kotlin Data Class. If a Kotlin class is marked with data keyword then it becomes a data class. For example:
data class Book(val name: String, val publisher: String, var reviewScore: Int)
Good thing about Kotlin Data Class is that when you declare a Kotlin Data Class, the compiler generates Constructor, toString(), equals(), hashCode(), and additional copy() and componentN() functions automatically.
Example
A Kotlin Data Class is instantiated the same way as other Kotlin classes:
data class Book(val name: String, val publisher: String, var reviewScore: Int) fun main(args: Array<String>) { val book = Book("Kotlin", "Tutorials Point", 10) println("Name = ${book.name}") println("Publisher = ${book.publisher}") println("Score = ${book.reviewScore}") }
When you run the above Kotlin program, it will generate the following output:
Name = Kotlin Publisher = Tutorials Point Score = 10
Copy Function
The copy() function is created automatically when we define a Kotlin Data Class. This copy function can be used to copy an object altering some of its properties but keeping the rest unchanged. Following is an example:
data class Book(val name: String, val publisher: String, var reviewScore: Int) fun main(args: Array<String>) { val original = Book("Kotlin", "Tutorials Point", 10) val copied = original.copy(reviewScore=5) println("Original Book") println("Name = ${original.name}") println("Publisher = ${original.publisher}") println("Score = ${original.reviewScore}") println("Copied Book") println("Name = ${copied.name}") println("Publisher = ${copied.publisher}") println("Score = ${copied.reviewScore}") }
When you run the above Kotlin program, it will generate the following output:
Original Book Name = Kotlin Publisher = Tutorials Point Score = 10 Copied Book Name = Kotlin Publisher = Tutorials Point Score = 5
toString Function
The toString() function is also created automatically when we define a Kotlin Data Class. This function returns a string representation of the object. Following is an example:
data class Book(val name: String, val publisher: String, var reviewScore: Int) fun main(args: Array<String>) { val book = Book("Kotlin", "Tutorials Point", 10) println(book.toString()) }
When you run the above Kotlin program, it will generate the following output:
Book(name=Kotlin, publisher=Tutorials Point, reviewScore=10)
hashCode() and equals() Functions
The hasCode()function returns hash code for the object. If two objects are equal, hashCode() returns the same integer value for the objects.
The equals() function returns true if two objects are equal or they have same hasCode value otherwise it returns false.
Following is an example:
data class Book(val name: String, val publisher: String, var reviewScore: Int) fun main(args: Array<String>) { val original = Book("Kotlin", "Tutorials Point", 10) val copy1 = original.copy(reviewScore=5) val copy2 = original.copy(reviewScore=7) println("Original Hashcode = ${original.hashCode()}") println("Copy1 Hashcode = ${copy1.hashCode()}") println("Copy2 Hashcode = ${copy2.hashCode()}") if( copy1.equals(copy2)){ println("Copy1 is equal to Copy2.") }else{ println("Copy1 is not equal to Copy2.") } }
When you run the above Kotlin program, it will generate the following output:
Original Hashcode = 1957710662 Copy1 Hashcode = 1957710657 Copy2 Hashcode = 1957710659 Copy1 is not equal to Copy2.
Destructuring Declarations
We can destructure an object into a number of variables using destructing declaration. For example:
data class Book(val name: String, val publisher: String, var reviewScore: Int) fun main(args: Array<String>) { val book = Book("Kotlin", "Tutorials Point", 10) val( name, publisher,reviewScore ) = book println("Name = $name") println("Publisher = $publisher") println("Score = $reviewScore") }
When you run the above Kotlin program, it will generate the following output:
Name = Kotlin Publisher = Tutorials Point Score = 10
Quiz Time (Interview & Exams Preparation)
Q 1 - What is the purpose of Data Classes in Kotlin :
A - Kotlin Data Classes are defined to hold the data only.
B - Kotlin Data Classes are synonym of abstract classes
C - Kotlin Data Classes are defined to hold data and associated functions
Answer : A
Explanation
Kotlin Data Classes are defined to hold the data only.
Q 2 - Which function is not created by default when we define a Kotlin Data Class
Answer : D
Explanation
All the mentioned functions are created automatically when we define Kotlin Data Class.
Q 2 - What is the function of componentN() in Kotlin Data Class
A - It is used to define new property of the class
B - It is used to count the number of properties in the class
C - It is used to destructure an object into a number of variables
Answer : C
Explanation
The function componentN() is used to destructure an object into a number of variables.