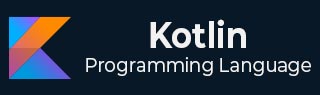
- Kotlin Tutorial
- Kotlin - Home
- Kotlin - Overview
- Kotlin - Environment Setup
- Kotlin - Architecture
- Kotlin - Basic Syntax
- Kotlin - Comments
- Kotlin - Keywords
- Kotlin - Variables
- Kotlin - Data Types
- Kotlin - Operators
- Kotlin - Booleans
- Kotlin - Strings
- Kotlin - Arrays
- Kotlin - Ranges
- Kotlin - Functions
- Kotlin Control Flow
- Kotlin - Control Flow
- Kotlin - if...Else Expression
- Kotlin - When Expression
- Kotlin - For Loop
- Kotlin - While Loop
- Kotlin - Break and Continue
- Kotlin Collections
- Kotlin - Collections
- Kotlin - Lists
- Kotlin - Sets
- Kotlin - Maps
- Kotlin Objects and Classes
- Kotlin - Class and Objects
- Kotlin - Constructors
- Kotlin - Inheritance
- Kotlin - Abstract Classes
- Kotlin - Interface
- Kotlin - Visibility Control
- Kotlin - Extension
- Kotlin - Data Classes
- Kotlin - Sealed Class
- Kotlin - Generics
- Kotlin - Delegation
- Kotlin - Destructuring Declarations
- Kotlin - Exception Handling
- Kotlin Useful Resources
- Kotlin - Quick Guide
- Kotlin - Useful Resources
- Kotlin - Discussion
Kotlin - Comments
A comment is a programmer-readable explanation or annotation in the Kotlin source code. They are added with the purpose of making the source code easier for humans to understand, and are ignored by Kotlin compiler.
Just like most modern languages, Kotlin supports single-line (or end-of-line) and multi-line (block) comments. Kotlin comments are very much similar to the comments available in Java, C and C++ programming languages.
Kotlin Single-line Comments
Single line comments in Kotlin starts with two forward slashes // and end with end of the line. So any text written in between // and the end of the line is ignored by Kotlin compiler.
Following is the sample Kotlin program which makes use of a single-line comment:
// This is a comment fun main() { println("Hello, World!") }
When you run the above Kotlin program, it will generate the following output:
Hello, World!
A single line comment can start from anywhere in the program and will end till the end of the line. For example, you can use single line comment as follows:
fun main() { println("Hello, World!") // This is also a comment }
Kotlin Multi-line Comments
A multi-line comment in Kotlin starts with /* and end with */. So any text written in between /* and */ will be treated as a comment and will be ignored by Kotlin compiler.
Multi-line comments also called Block comments in Kotlin.
Following is the sample Kotlin program which makes use of a multi-line comment:
/* This is a multi-line comment and it can span * as many lines as you like */ fun main() { println("Hello, World!") }
When you run the above Kotlin program, it will generate the following output:
Hello, Word!
Kotlin Nested Comments
Block comments in Kotlin can be nested, which means a single-line comment or multi-line comments can sit inside a multi-line comment as below:
/* This is a multi-line comment and it can span * as many lines as you like /* This is a nested comment */ // Another nested comment */ fun main() { println("Hello, World!") }
When you run the above Kotlin program, it will generate the following output:
Hello, World!
Quiz Time (Interview & Exams Preparation)
Q 1 - Which of the following statements is correct about Kotlin Comments:
A - Kotlin comments are ignored by the compiler
B - Kotlin comments helps programmer to increase the readability of the code
Answer : D
Explanation
All the given three statements are correct for Kotlin comments.
Q 2 - What could be the length of a Kotlin Single-line comment:
Answer : C
Explanation
There is no specification about the length of Kotlin comments, so single-line comment can be as long as a line. A multi-line comment can also span as long as you want.
Q 3 - Which of the following statements is not correct about Kotlin comments
A - Kotlin comments degrade the performance of the Kotlin program
B - Kotlin supports single-line and multi-line comments
C - Kotlin comments are recommended to make code more readable
D - Kotlin comments are very much similar to the comments available in Java, C and C++
Answer : A
Explanation
Statement A is incorrect because comments never impact a Kotlin program regardless the volume of the comments available in the program because they are ignore by the compiler and they do not cconsider while generating the final bytecode.