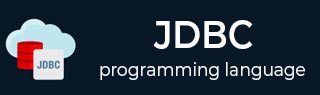
- JDBC Tutorial
- JDBC - Home
- JDBC - Introduction
- JDBC - SQL Syntax
- JDBC - Environment
- JDBC - Sample Code
- JDBC - Driver Types
- JDBC - Connections
- JDBC - Statements
- JDBC - Result Sets
- JDBC - Data Types
- JDBC - Transactions
- JDBC - Exceptions
- JDBC - Batch Processing
- JDBC - Stored Procedure
- JDBC - Streaming Data
- JDBC Examples
- JDBC - Create Database
- JDBC - Select Database
- JDBC - Drop Database
- JDBC - Create Tables
- JDBC - Drop Tables
- JDBC - Insert Records
- JDBC - Select Records
- JDBC - Update Records
- JDBC - Delete Records
- JDBC - WHERE Clause
- JDBC - Like Clause
- JDBC - Sorting Data
- JDBC Useful Resources
- JDBC - Questions and Answers
- JDBC - Quick Guide
- JDBC - Useful Resources
- JDBC - Discussion
- Useful - Java Tutorials
JDBC - Streaming ASCII and Binary Data
A PreparedStatement object has the ability to use input and output streams to supply parameter data. This enables you to place entire files into database columns that can hold large values, such as CLOB and BLOB data types.
There are following methods, which can be used to stream data −
setAsciiStream() − This method is used to supply large ASCII values.
setCharacterStream() − This method is used to supply large UNICODE values.
setBinaryStream() − This method is used to supply large binary values.
The setXXXStream() method requires an extra parameter, the file size, besides the parameter placeholder. This parameter informs the driver how much data should be sent to the database using the stream.
This example would create a database table XML_Data and then XML content would be written into this table.
Copy and paste the following example in FirstApplication.java, compile and run as follows −
import java.io.ByteArrayInputStream; import java.io.ByteArrayOutputStream; import java.io.File; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.IOException; import java.io.InputStream; import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; public class TestApplication { static final String DB_URL = "jdbc:mysql://localhost/TUTORIALSPOINT"; static final String USER = "guest"; static final String PASS = "guest123"; static final String QUERY = "SELECT Data FROM XML_Data WHERE id=100"; static final String INSERT_QUERY="INSERT INTO XML_Data VALUES (?,?)"; static final String CREATE_TABLE_QUERY = "CREATE TABLE XML_Data (id INTEGER, Data LONG)"; static final String DROP_TABLE_QUERY = "DROP TABLE XML_Data"; static final String XML_DATA = "<Employee><id>100</id><first>Zara</first><last>Ali</last><Salary>10000</Salary><Dob>18-08-1978</Dob></Employee>"; public static void createXMLTable(Statement stmt) throws SQLException{ System.out.println("Creating XML_Data table..." ); //Drop table first if it exists. try{ stmt.executeUpdate(DROP_TABLE_QUERY); }catch(SQLException se){ } stmt.executeUpdate(CREATE_TABLE_QUERY); } public static void main(String[] args) { // Open a connection try(Connection conn = DriverManager.getConnection(DB_URL, USER, PASS); Statement stmt = conn.createStatement(); PreparedStatement pstmt = conn.prepareStatement(INSERT_QUERY); ) { createXMLTable(stmt); ByteArrayInputStream bis = new ByteArrayInputStream(XML_DATA.getBytes()); pstmt.setInt(1,100); pstmt.setAsciiStream(2,bis,XML_DATA.getBytes().length); pstmt.execute(); //Close input stream bis.close(); ResultSet rs = stmt.executeQuery(QUERY); // Get the first row if (rs.next ()){ //Retrieve data from input stream InputStream xmlInputStream = rs.getAsciiStream (1); int c; ByteArrayOutputStream bos = new ByteArrayOutputStream(); while (( c = xmlInputStream.read ()) != -1) bos.write(c); //Print results System.out.println(bos.toString()); } // Clean-up environment rs.close(); } catch (SQLException | IOException e) { e.printStackTrace(); } } }
Now let us compile the above example as follows −
C:\>javac FirstApplication.java C:\>
When you run FirstApplication, it produces the following result −
C:\>java FirstApplication Creating XML_Data table... <Employee><id>100</id><first>Zara</first><last>Ali</last><Salary>10000</Salary><Dob>18-08-1978</Dob></Employee> C:\>