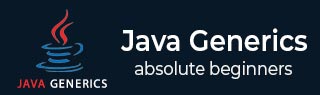
- Java Generics Tutorial
- Java Generics - Home
- Java Generics - Overview
- Java Generics - Environment Setup
- Examples - Generic Classes
- Java Generics - Generic Classes
- Type Parameter Naming Conventions
- Java Generics - Type inference
- Java Generics - Generic Methods
- Java Generics - Multiple Type
- Java Generics - Parameterized Types
- Java Generics - Raw Types
- Examples - Bounded Type
- Bounded Type Parameters
- Java Generics - Multiple Bounds
- Examples - Collections
- Java Generics - Generic List
- Java Generics - Generic Set
- Java Generics - Generic Map
- Examples - Wild Cards
- Upper Bounded Wildcards
- Generics - Unbounded Wildcards
- Lower Bounded Wildcards
- Generics - Guidelines for Wildcards
- Type Erasure
- Java Generics - Types Erasure
- Java Generics - Bound Types Erasure
- Unbounded Types Erasure
- Java Generics - Methods Erasure
- Restrictions on Generics
- Java Generics - No Primitive Types
- Java Generics - No Instance
- Java Generics - No Static field
- Java Generics - No Cast
- Java Generics - No instanceOf
- Java Generics - No Array
- Java Generics - No Exception
- Java Generics - No Overload
- Java Generics Useful Resources
- Java Generics - Quick Guide
- Java Generics - Useful Resources
- Java Generics - Discussion
Java Generics - Multiple Bounds
A type parameter can have multiple bounds.
Syntax
public static <T extends Number & Comparable<T>> T maximum(T x, T y, T z)
Where
maximum − maximum is a generic method.
T − The generic type parameter passed to generic method. It can take any Object.
Description
The T is a type parameter passed to the generic class Box and should be subtype of Number class and must implments Comparable interface. In case a class is passed as bound, it should be passed first before interface otherwise compile time error will occur.
Example
Create the following java program using any editor of your choice.
package com.tutorialspoint; public class GenericsTester { public static void main(String[] args) { System.out.printf("Max of %d, %d and %d is %d\n\n", 3, 4, 5, maximum( 3, 4, 5 )); System.out.printf("Max of %.1f,%.1f and %.1f is %.1f\n\n", 6.6, 8.8, 7.7, maximum( 6.6, 8.8, 7.7 )); } public static <T extends Number & Comparable<T>> T maximum(T x, T y, T z) { T max = x; if(y.compareTo(max) > 0) { max = y; } if(z.compareTo(max) > 0) { max = z; } return max; } // Compiler throws error in case of below declaration /* public static <T extends Comparable<T> & Number> T maximum1(T x, T y, T z) { T max = x; if(y.compareTo(max) > 0) { max = y; } if(z.compareTo(max) > 0) { max = z; } return max; }*/ }
This will produce the following result −
Output
Max of 3, 4 and 5 is 5 Max of 6.6,8.8 and 7.7 is 8.8
Advertisements