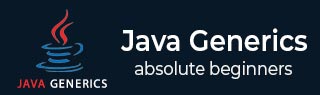
- Java Generics Tutorial
- Java Generics - Home
- Java Generics - Overview
- Java Generics - Environment Setup
- Examples - Generic Classes
- Java Generics - Generic Classes
- Type Parameter Naming Conventions
- Java Generics - Type inference
- Java Generics - Generic Methods
- Java Generics - Multiple Type
- Java Generics - Parameterized Types
- Java Generics - Raw Types
- Examples - Bounded Type
- Bounded Type Parameters
- Java Generics - Multiple Bounds
- Examples - Collections
- Java Generics - Generic List
- Java Generics - Generic Set
- Java Generics - Generic Map
- Examples - Wild Cards
- Upper Bounded Wildcards
- Generics - Unbounded Wildcards
- Lower Bounded Wildcards
- Generics - Guidelines for Wildcards
- Type Erasure
- Java Generics - Types Erasure
- Java Generics - Bound Types Erasure
- Unbounded Types Erasure
- Java Generics - Methods Erasure
- Restrictions on Generics
- Java Generics - No Primitive Types
- Java Generics - No Instance
- Java Generics - No Static field
- Java Generics - No Cast
- Java Generics - No instanceOf
- Java Generics - No Array
- Java Generics - No Exception
- Java Generics - No Overload
- Java Generics Useful Resources
- Java Generics - Quick Guide
- Java Generics - Useful Resources
- Java Generics - Discussion
Java Generics - Lower Bounded Wildcards
The question mark (?), represents the wildcard, stands for unknown type in generics. There may be times when you'll want to restrict the kinds of types that are allowed to be passed to a type parameter. For example, a method that operates on numbers might only want to accept instances of Integer or its superclasses like Number.
To declare a lower bounded Wildcard parameter, list the ?, followed by the super keyword, followed by its lower bound.
Example
Following example illustrates how super is used to specify an lower bound wildcard.
package com.tutorialspoint; import java.util.ArrayList; import java.util.List; public class GenericsTester { public static void addCat(List<? super Cat> catList) { catList.add(new RedCat()); System.out.println("Cat Added"); } public static void main(String[] args) { List<Animal> animalList= new ArrayList<Animal>(); List<Cat> catList= new ArrayList<Cat>(); List<RedCat> redCatList= new ArrayList<RedCat>(); List<Dog> dogList= new ArrayList<Dog>(); //add list of super class Animal of Cat class addCat(animalList); //add list of Cat class addCat(catList); //compile time error //can not add list of subclass RedCat of Cat class //addCat(redCatList); //compile time error //can not add list of subclass Dog of Superclass Animal of Cat class //addCat.addMethod(dogList); } } class Animal {} class Cat extends Animal {} class RedCat extends Cat {} class Dog extends Animal {}
This will produce the following result −
Cat Added Cat Added
Advertisements