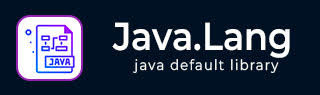
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java - Byte class
Introduction
The Java Byte class class wraps a value of primitive type byte in an object. An object of type Byte contains a single field whose type is byte.
Class Declaration
Following is the declaration for java.lang.Byte class −
public final class Byte extends Number implements Comparable<Byte>
Field
Following are the fields for java.lang.Byte class −
static byte MAX_VALUE − This is constant holding the maximum value a byte can have, 27-1.
static byte MIN_VALUE − This is constant holding the minimum value a byte can have, -27.
static int SIZE − This is the number of bits used to represent a byte value in two's complement binary form.
static Class<Byte> TYPE − This is the Class instance representing the primitive type byte.
Class constructors
Sr.No. | Constructor & Description |
---|---|
1 |
Byte(byte value) This constructs a newly allocated Byte object that represents the specified byte value. |
2 |
Byte(String s) This constructs a newly allocated Byte object that represents the byte value indicated by the String parameter. |
Class methods
Sr.No. | Method & Description |
---|---|
1 | byte byteValue()
This method returns the value of this Byte as a byte. |
2 | int compareTo(Byte anotherByte)
This method compares two Byte objects numerically. |
3 | static Byte decode(String nm)
This method decodes a String into a Byte. |
4 | double doubleValue()
This method returns the value of this Byte as a double. |
5 | boolean equals(Object obj)
This method compares this object to the specified object. |
6 | float floatValue()
This method returns the value of this Byte as a float. |
7 | int hashCode()
This method returns a hash code for this Byte. |
8 | int intValue()
This method returns the value of this Byte as an int. |
9 | long longValue()
This method returns the value of this Byte as a long. |
10 | static byte parseByte(String s)
This method parses the string argument as a signed decimal byte. |
11 | static byte parseByte(String s, int radix)
This method parses the string argument as a signed byte in the radix specified by the second argument. |
12 | short shortValue()
This method returns the value of this Byte as a short. |
13 | String toString()
This method returns a String object representing this Byte's value. |
14 | static String toString(byte b)
This method returns a new String object representing the specified byte. |
15 | static Byte valueOf(byte b)
This method returns a Byte instance representing the specified byte value. |
16 | static Byte valueOf(String s)
This method returns a Byte object holding the value given by the specified String. |
17 | static Byte valueOf(String s, int radix)
This method returns a Byte object holding the value extracted from the specified String when parsed with the radix given by the second argument. |
Methods inherited
This class inherits methods from the following classes −
- java.lang.Object
Example
The following example shows the usage of Byte class to get byte from a string.
package com.tutorialspoint; public class ByteDemo { public static void main(String[] args) { // create a String s and assign value to it String s = "+120"; // create a Byte object b Byte b; // get the value of byte from string b = Byte.valueOf(s); // print b value System.out.println( "Byte value of string " + s + " is " + b ); } }
Output
Let us compile and run the above program, this will produce the following result −
Byte value of string +120 is 120